Making the Most Out of PHP’s ord() Function
PHP is a versatile programming language, widely used for web development, and offers a rich set of functions to manipulate strings, characters, and data. One such function that can be extremely useful but is often overlooked is ord(). The ord() function returns the ASCII value of the first character in a string, opening up a world of possibilities for text processing, character validation, and more.
Table of Contents
In this blog post, we’ll explore the ord() function in depth, uncovering its various applications and demonstrating how to harness its power effectively in your PHP projects. We’ll provide code samples, practical examples, and tips to help you make the most out of this underrated PHP function.
Understanding the Basics
Before diving into practical examples, let’s begin by understanding the basics of the ord() function.
Syntax
The ord() function in PHP has a simple syntax:
php ord(string $string): int|false
- $string: The input string for which you want to retrieve the ASCII value of the first character.
The function returns an integer representing the ASCII value of the first character in the string or false if the string is empty.
ASCII Values
The ASCII (American Standard Code for Information Interchange) system assigns a unique numeric value to each character. These values are used to represent characters in computers and are the basis for character encoding in many programming languages.
For example, the ASCII value for the letter ‘A’ is 65, ‘B’ is 66, and so on. You can use the ord() function to retrieve these values programmatically.
Now that we have a basic understanding of the ord() function, let’s explore some practical applications.
Application 1: Character Validation
One of the most common use cases for the ord() function is character validation. You can use it to ensure that a string contains only specific types of characters, such as alphabets, digits, or symbols.
Example 1: Validating Alphabetic Characters
php function isAlphabetic($string) { $string = trim($string); if (empty($string)) { return false; } for ($i = 0; $i < strlen($string); $i++) { $ascii = ord($string[$i]); if (($ascii < 65 || $ascii > 90) && ($ascii < 97 || $ascii > 122)) { return false; // Non-alphabetic character found } } return true; // All characters are alphabetic } // Test cases var_dump(isAlphabetic("Hello")); // true var_dump(isAlphabetic("12345")); // false var_dump(isAlphabetic("Hello123")); // false
In this example, the isAlphabetic() function checks if a given string contains only alphabetic characters. It iterates through each character, uses ord() to obtain its ASCII value, and then validates whether the character is within the ASCII range of uppercase and lowercase alphabets.
Example 2: Validating Numeric Characters
php function isNumeric($string) { $string = trim($string); if (empty($string)) { return false; } for ($i = 0; $i < strlen($string); $i++) { $ascii = ord($string[$i]); if ($ascii < 48 || $ascii > 57) { return false; // Non-numeric character found } } return true; // All characters are numeric } // Test cases var_dump(isNumeric("12345")); // true var_dump(isNumeric("Hello")); // false var_dump(isNumeric("123Hello")); // false
This isNumeric() function validates whether a string contains only numeric characters. It follows a similar approach to isAlphabetic(), using ord() to obtain ASCII values and checking if they fall within the range of numeric characters.
Application 2: Password Strength Check
The ord() function can also be used in password strength checks. For instance, you might want to ensure that a user’s password contains a mix of uppercase, lowercase letters, digits, and special symbols. Here’s a simplified example:
php function isStrongPassword($password) { $length = strlen($password); // Check length if ($length < 8) { return false; } // Initialize character type counters $hasLower = false; $hasUpper = false; $hasDigit = false; $hasSymbol = false; for ($i = 0; $i < $length; $i++) { $ascii = ord($password[$i]); if ($ascii >= 97 && $ascii <= 122) { $hasLower = true; // Lowercase letter found } elseif ($ascii >= 65 && $ascii <= 90) { $hasUpper = true; // Uppercase letter found } elseif ($ascii >= 48 && $ascii <= 57) { $hasDigit = true; // Digit found } else { $hasSymbol = true; // Special symbol found } } // Check if all character types are present return $hasLower && $hasUpper && $hasDigit && $hasSymbol; } // Test cases var_dump(isStrongPassword("Passw0rd$")); // true var_dump(isStrongPassword("weak")); // false
This isStrongPassword() function checks if a password contains at least one lowercase letter, one uppercase letter, one digit, and one special symbol while ensuring a minimum length of 8 characters.
Application 3: Sorting Characters
You can use the ord() function to create custom sorting algorithms for strings based on the ASCII values of characters.
Example: Sorting Characters in a String
php function sortString($string) { $length = strlen($string); $charArray = []; // Convert the string to an array of characters for ($i = 0; $i < $length; $i++) { $charArray[] = $string[$i]; } // Sort the array based on ASCII values using ord() usort($charArray, function($a, $b) { return ord($a) - ord($b); }); // Convert the sorted array back to a string return implode('', $charArray); } $inputString = "bcaed"; $sortedString = sortString($inputString); echo $sortedString; // Output: "abcde"
In this example, the sortString() function converts a string into an array of characters, sorts them based on their ASCII values using ord(), and then converts the sorted array back into a string. This allows you to sort strings in a custom order.
Application 4: Reversing a String
The ord() function can also be used to reverse a string character by character.
Example: Reversing a String
php function reverseString($string) { $length = strlen($string); $reversedString = ''; for ($i = $length - 1; $i >= 0; $i--) { $reversedString .= $string[$i]; } return $reversedString; } $inputString = "Hello, World!"; $reversedString = reverseString($inputString); echo $reversedString; // Output: "!dlroW ,olleH"
Here, the reverseString() function iterates through the input string in reverse order and builds a new string character by character. The ord() function isn’t directly involved in this example, but it’s essential to understand character positions when working with strings.
Application 5: Custom String Manipulation
The ord() function can be a valuable tool for creating custom string manipulation functions. Let’s look at an example where we replace all occurrences of a specific character in a string with another character.
Example: Custom String Replacement
php function replaceChar($string, $find, $replaceWith) { $length = strlen($string); $result = ''; for ($i = 0; $i < $length; $i++) { if ($string[$i] == $find) { $result .= $replaceWith; } else { $result .= $string[$i]; } } return $result; } $inputString = "Hello, World!"; $modifiedString = replaceChar($inputString, 'o', '*'); echo $modifiedString; // Output: "Hell*, W*rld!"
In this example, the replaceChar() function replaces all occurrences of a specified character (in this case, ‘o’) with another character (‘*’). The ord() function isn’t directly used here, but it helps us navigate and manipulate characters within the string.
Tips for Efficient Use of ord()
While the ord() function can be a valuable asset in your PHP toolbox, it’s essential to use it efficiently. Here are some tips to keep in mind:
1. Avoid Excessive Calls
Calling ord() within a loop or multiple times unnecessarily can impact performance. Minimize calls by storing the result in a variable if you need to reference it multiple times.
2. Handle Multibyte Characters
The ord() function returns the ASCII value of the first character, which may not work correctly for multibyte character encodings like UTF-8. Consider using multibyte string functions (mb_*) for handling such encodings.
3. Sanitize Input
When using ord() for character validation or manipulation, ensure that you sanitize and validate input strings to prevent unexpected behavior.
4. Use Alternative Functions
For certain tasks like string sorting and character replacement, PHP provides dedicated functions (usort(), str_replace()) that may be more efficient and easier to use than ord().
Conclusion
The ord() function in PHP might seem simple at first glance, but it offers a wide range of possibilities for character manipulation, validation, and text processing. By understanding its fundamentals and exploring practical examples, you can unlock its full potential in your PHP projects.
Whether you’re validating user input, implementing custom sorting algorithms, or performing character replacement, the ord() function can be a valuable tool in your coding arsenal. So, the next time you encounter a text-related task in PHP, consider making the most out of PHP’s ord() function to simplify and enhance your code.
Table of Contents
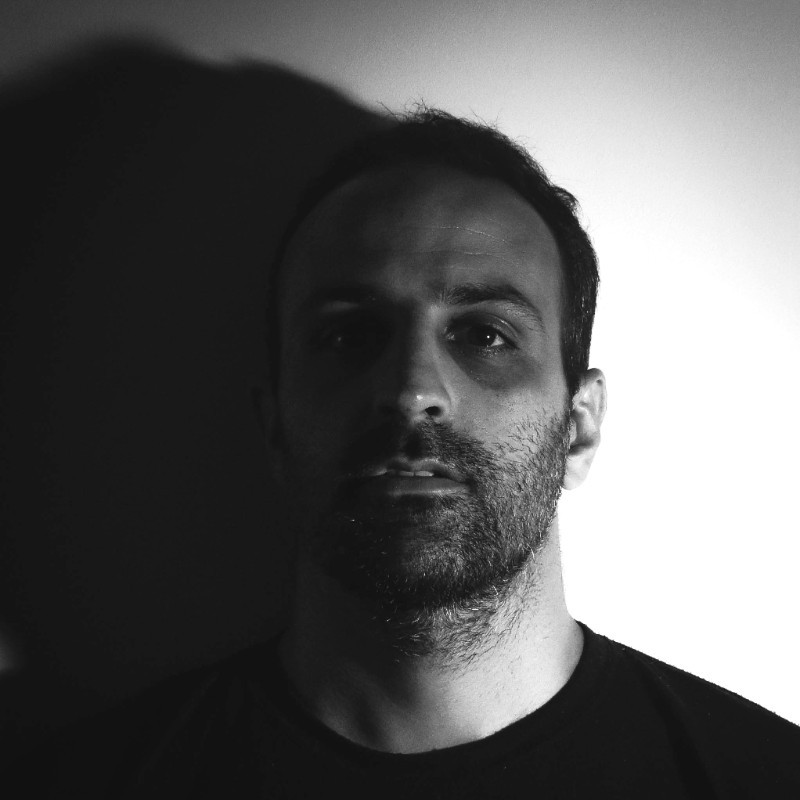
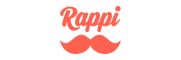