Exploring the Power of PHP’s SimpleXML
In the world of web development, managing and manipulating XML data has become an integral part of creating dynamic and interactive websites. XML (Extensible Markup Language) is widely used for structuring and representing data, making it essential for developers to efficiently parse and manipulate XML documents. This is where PHP’s SimpleXML comes into play, offering a convenient and straightforward way to work with XML data in your PHP applications. In this blog, we’ll delve into the power of SimpleXML, exploring its features, use cases, and showcasing code samples to illustrate its capabilities.
1. Understanding SimpleXML
SimpleXML is a PHP extension that provides a simple and intuitive approach to parsing and manipulating XML documents. It abstracts the complexities of working with XML data, allowing developers to access and manipulate XML elements and attributes with ease. Unlike more traditional methods like using the DOM extension, SimpleXML is specifically designed to be user-friendly and efficient for common XML processing tasks.
2. The Basics of SimpleXML
Getting started with SimpleXML is remarkably straightforward. Once you have an XML document, you can load it into a SimpleXML object using the simplexml_load_string() or simplexml_load_file() functions. Let’s take a look at an example:
php $xmlString = '<?xml version="1.0"?> <book> <title>Exploring PHP</title> <author>John Doe</author> </book>'; $book = simplexml_load_string($xmlString);
In this example, we’ve loaded an XML string into a SimpleXML object called $book. Now, we can easily access its elements and attributes:
php echo "Title: " . $book->title . "<br>"; echo "Author: " . $book->author . "<br>";
3. Navigating Through XML
SimpleXML allows you to navigate through XML documents using a convenient object-oriented syntax. You can access child elements, attributes, and their values using the arrow (->) operator. If an element has multiple occurrences, SimpleXML returns an array of elements, making it easy to iterate through them.
php $xml = simplexml_load_file('data.xml'); // Accessing child elements foreach ($xml->person as $person) { echo "Name: " . $person->name . "<br>"; echo "Age: " . $person->age . "<br>"; } // Accessing attributes foreach ($xml->book as $book) { echo "Title: " . $book->title . "<br>"; echo "Author: " . $book->author . "<br>"; echo "Published Year: " . $book['year'] . "<br>"; }
4. Manipulating XML Data
Beyond parsing, SimpleXML excels at manipulating XML data, allowing you to add, modify, and delete elements and attributes effortlessly.
4.1. Adding Elements and Attributes
To add a new element or attribute, you can use the same object-oriented syntax. The addChild() method adds a new child element, and you can assign values directly.
php $root = $xml->addChild('newPerson'); $root->addChild('name', 'Jane Doe'); $root->addChild('age', 28); $newBook = $xml->addChild('book'); $newBook->addChild('title', 'Advanced PHP'); $newBook->addChild('author', 'Jane Smith'); $newBook->addAttribute('year', '2023');
4.2. Modifying Elements and Attributes
Modifying elements and attributes is equally straightforward. You can directly assign new values to them.
php $firstPerson = $xml->person[0]; $firstPerson->name = 'Updated Name'; $firstPerson->age = 30; $firstBook = $xml->book[0]; $firstBook->title = 'Revised PHP Basics'; $firstBook->author = 'Updated Author'; $firstBook['year'] = '2022';
4.3. Deleting Elements and Attributes
Removing elements and attributes is as simple as using the unset() function.
php unset($xml->person[1]); // Remove the second person unset($xml->book[2]['year']); // Remove the year attribute of the third book
5. Use Cases of SimpleXML
The power and simplicity of SimpleXML make it a valuable tool for a variety of use cases.
5.1. Reading Configuration Files
Many applications rely on configuration files to store settings. XML configuration files can be easily read and processed using SimpleXML.
php $config = simplexml_load_file('config.xml'); $databaseHost = $config->database->host; $databaseUsername = $config->database->username; $databasePassword = $config->database->password;
5.2. Consuming Web Services
When consuming data from web services that provide responses in XML format, SimpleXML makes it painless to extract the required information.
php $response = file_get_contents('https://api.example.com/data.xml'); $data = simplexml_load_string($response); foreach ($data->item as $item) { echo "Item: " . $item->name . "<br>"; echo "Price: " . $item->price . "<br>"; }
5.3. Generating RSS Feeds
Creating and updating RSS feeds for your website becomes effortless with SimpleXML.
php $rss = new SimpleXMLElement('<?xml version="1.0" encoding="UTF-8"?><rss></rss>'); $rss->addAttribute('version', '2.0'); $channel = $rss->addChild('channel'); $channel->addChild('title', 'My Website'); $channel->addChild('link', 'https://www.example.com'); $channel->addChild('description', 'Latest updates from my website'); $item = $channel->addChild('item'); $item->addChild('title', 'New Blog Post'); $item->addChild('link', 'https://www.example.com/blog/post1'); $item->addChild('pubDate', 'Sat, 07 Aug 2023 00:00:00 GMT'); echo $rss->asXML();
Conclusion
PHP’s SimpleXML extension unlocks a world of possibilities when it comes to parsing and manipulating XML data. Its user-friendly syntax and powerful capabilities make it a must-have tool for any developer working with XML. Whether you’re reading configuration files, consuming web services, or generating XML documents, SimpleXML simplifies the process and enhances your productivity. By exploring the features and examples provided in this blog, you’re now equipped to harness the true power of SimpleXML and elevate your XML-related tasks to a whole new level. Happy coding!
Table of Contents
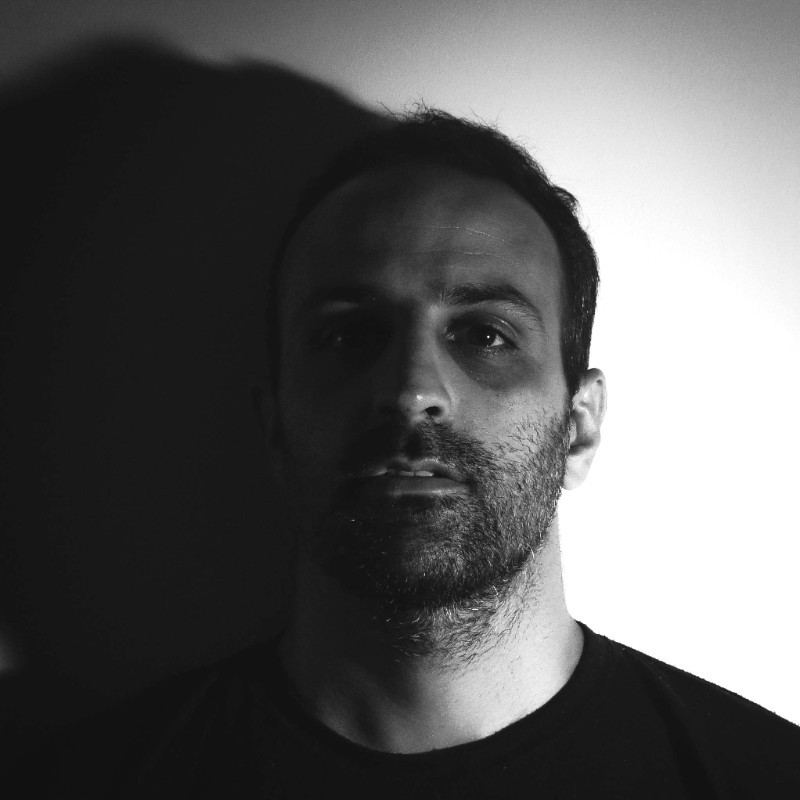
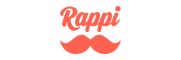