PHP’s preg_match() Function: An In-Depth Guide
When it comes to string manipulation and pattern matching in PHP, the preg_match() function stands as a versatile tool in every developer’s toolkit. Whether you’re dealing with form validation, text parsing, or data extraction, understanding how to use preg_match() effectively can significantly enhance your coding prowess. In this guide, we will delve into the depths of preg_match(), exploring its syntax, use cases, and providing practical examples to demonstrate its power.
1. Understanding Regular Expressions:
Before we dive into the intricacies of the preg_match() function, it’s crucial to grasp the concept of regular expressions. Regular expressions, often abbreviated as regex, are powerful tools for pattern matching and text manipulation. They provide a concise way to describe complex search patterns within strings.
Regular expressions consist of various characters and symbols that represent different patterns. Some common symbols include:
.: Matches any character except a newline.
*: Matches the preceding element zero or more times.
+: Matches the preceding element one or more times.
?: Matches the preceding element zero or one time.
[]: Defines a character set, allowing any character within the set to match.
(): Groups together expressions.
\: Escapes a special character, allowing you to match it literally.
2. Syntax of preg_match():
The preg_match() function is used to perform regular expression matching on a given string. Its syntax is as follows:
php preg_match(pattern, subject [, matches [, flags [, offset]]])
- pattern: The regular expression pattern to match.
- subject: The input string you want to search within.
- matches: An optional array that will hold the matched groups.
- flags: Optional flags that modify the behavior of the function.
- offset: An optional parameter that specifies the starting position for the search within the subject string.
3. Common Flags for preg_match():
Flags in the preg_match() function can alter how pattern matching is performed. Some frequently used flags include:
i: Case-insensitive matching.
m: Treat the subject as a multi-line string.
s: Allow the dot (.) to match newline characters.
u: Treat the pattern and subject as UTF-8 strings.
x: Ignore whitespace and comments in the pattern.
4. Using preg_match() for Basic Pattern Matching:
Let’s start with a simple example of using preg_match() for basic pattern matching. Suppose we want to check if a given string contains the word “PHP.” Here’s how you can achieve this:
php $string = "PHP is a popular scripting language."; $pattern = "/PHP/"; if (preg_match($pattern, $string)) { echo "The string contains the word 'PHP'."; } else { echo "The string does not contain the word 'PHP'."; }
In this example, the pattern /PHP/ specifies the regular expression to match the literal string “PHP.” The preg_match() function returns 1 if a match is found, otherwise 0.
5. Matching and Capturing Groups:
One of the powerful features of preg_match() is the ability to capture specific parts of the matched string using capturing groups. Capturing groups are defined using parentheses (). The content matched by each group can be retrieved using the optional matches parameter of the function.
Consider the following example where we want to extract a date in the format “YYYY-MM-DD” from a string:
php $string = "This event took place on 2023-08-19."; $pattern = "/(\d{4}-\d{2}-\d{2})/"; if (preg_match($pattern, $string, $matches)) { echo "Date: " . $matches[1]; } else { echo "No date found."; }
In this example, the pattern “/(\d{4}-\d{2}-\d{2})/” captures the date in the “YYYY-MM-DD” format. The matched date is stored in the $matches array at index 1.
6. Modifiers and Advanced Matching:
Modifiers are used to refine the behavior of regular expressions. Here are a few examples:
^: Matches the start of a string.
$: Matches the end of a string.
\b: Matches a word boundary.
\s: Matches whitespace characters.
\w: Matches word characters (letters, digits, and underscores).
For instance, if we want to validate an email address and ensure it starts and ends with a word boundary, we can use the following example:
php $email = "user@example.com"; $pattern = "/^\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b$/"; if (preg_match($pattern, $email)) { echo "Valid email address."; } else { echo "Invalid email address."; }
Conclusion
The preg_match() function is a versatile tool that empowers developers to perform advanced pattern matching and text manipulation tasks with ease. By understanding the basics of regular expressions, the syntax of preg_match(), and various flags and modifiers, you can unlock the full potential of this function in your PHP projects. Whether you’re validating user input, parsing data, or searching for specific patterns within strings, preg_match() is an invaluable asset in your coding journey. Experiment with different patterns, explore its capabilities, and enhance your programming repertoire with this powerful function. Happy coding!
Table of Contents
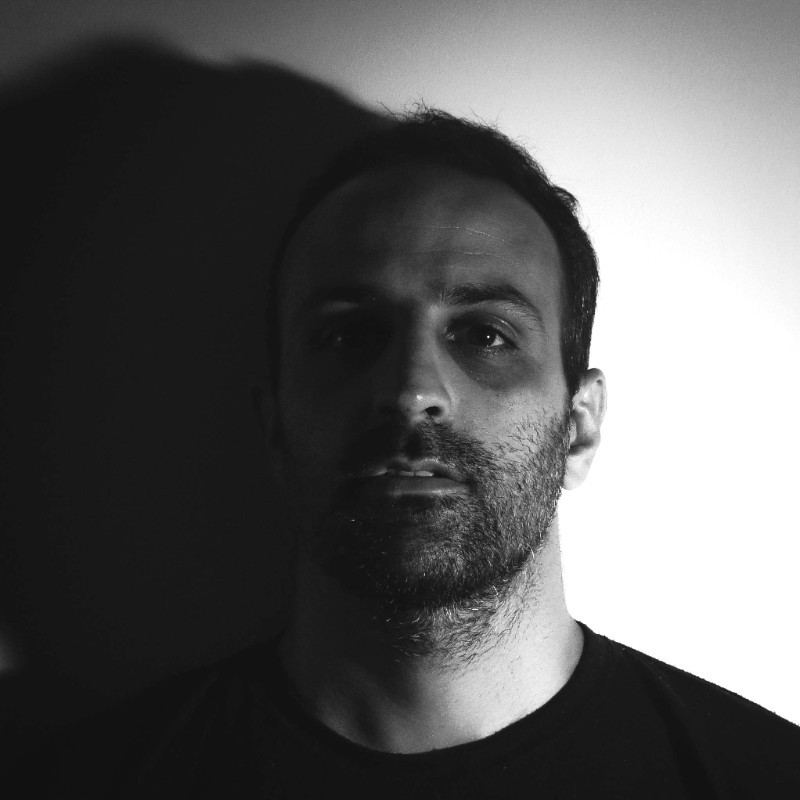
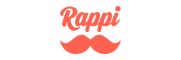