The Art of PHP’s preg_replace() Function
Regular expressions are like a secret weapon for developers, allowing them to perform intricate string manipulations with ease. When it comes to handling complex string patterns, PHP’s preg_replace() function steps up to the plate. In this guide, we’ll delve into the art of using preg_replace() to master string transformations and solve various real-world challenges.
1. Introduction to preg_replace()
1.1. What is preg_replace()?
At its core, the preg_replace() function is a versatile tool in PHP used for searching and replacing text using regular expressions. Unlike simpler string functions, like str_replace(), preg_replace() allows you to harness the power of pattern matching to manipulate strings in intricate ways. Regular expressions are a sequence of characters defining a search pattern, and preg_replace() employs these patterns to perform substitutions within strings.
1.2. Benefits of using regular expressions
Regular expressions offer a plethora of advantages, such as:
- Pattern Flexibility: Regular expressions provide a concise way to define complex search patterns, enabling you to match a wide range of text variations.
- Efficient String Manipulation: With preg_replace(), you can perform multiple replacements in one pass, saving execution time.
- Dynamic Replacements: Regular expressions allow you to dynamically generate replacement strings based on captured groups, providing unprecedented flexibility.
- Text Validation and Parsing: They are not just for replacements; you can use regular expressions to validate input strings or extract specific data patterns.
2. Basic Syntax and Usage
2.1. Syntax breakdown
The basic syntax of preg_replace() involves three main arguments:
php $result = preg_replace($pattern, $replacement, $subject); $pattern: The regular expression pattern to search for. $replacement: The text to replace the matched pattern with. $subject: The input string you want to perform replacements on.
2.2. Simple text replacement
Let’s start with a simple example. Suppose you want to replace all occurrences of the word “apple” with “orange” in a given text:
php $text = "I have an apple, and she has an apple too."; $pattern = "/apple/"; $replacement = "orange"; $result = preg_replace($pattern, $replacement, $text); echo $result;
Output:
css I have an orange, and she has an orange too.
In this case, the $pattern is a basic string, but you can easily see the potential for more intricate patterns.
2.3. Case-insensitive replacements
Sometimes, you might want to replace text regardless of its case. You can achieve this using the i modifier in your pattern:
php $text = "Red, red, GREEN, blue, RED"; $pattern = "/red/i"; $replacement = "colorful"; $result = preg_replace($pattern, $replacement, $text); echo $result;
Output:
colorful, colorful, GREEN, blue, colorful
The i modifier ensures a case-insensitive match, making the replacement process more versatile.
3. Pattern Modifiers
3.1. Exploring modifiers like i, m, and s
Modifiers are characters that follow the closing delimiter in a regular expression pattern and affect how the pattern is interpreted. Some commonly used modifiers are:
- i: Case-insensitive matching, as seen in the previous example.
- m: Multiline matching, where ^ and $ match the start and end of each line, not just the start and end of the entire string.
- s: Allows the dot (.) to match newline characters as well.
For instance, with the m modifier:
php $text = "Line 1: Hello there\nLine 2: How are you?"; $pattern = "/^Line \d+: /m"; $replacement = ""; $result = preg_replace($pattern, $replacement, $text); echo $result;
Output:
sql Hello there How are you?
Here, the m modifier enables matching at the start of each line, ensuring the pattern is applied to each line independently.
4. Backreferences and Subpatterns
4.1. Utilizing captured groups
Captured groups are portions of a pattern enclosed in parentheses, and they allow you to extract and use specific parts of a matched pattern in your replacement. Consider the following example:
php $text = "John Doe, Alex Smith, Jane Johnson"; $pattern = "/(\w+) (\w+)/"; $replacement = "$2, $1"; $result = preg_replace($pattern, $replacement, $text); echo $result;
Output:
Doe, John Smith, Alex Johnson, Jane
In this case, (\w+) and (\w+) are two captured groups, capturing the first and last names respectively. The replacement string $2, $1 swaps their positions.
4.2. Dynamic replacements
You can use not only captured groups but also functions to generate replacement strings dynamically. Suppose you want to capitalize the first letter of each word:
php $text = "hello world, how are you?"; $pattern = "/\b(\w)/e"; $replacement = 'strtoupper("$1")'; $result = preg_replace($pattern, $replacement, $text); echo $result;
Output:
sql Hello World, How Are You?
Here, the \b(\w) pattern captures the first letter of each word, and the e modifier evaluates the replacement as PHP code.
5. Advanced Use Cases
5.1. Removing HTML tags from a string
You can leverage preg_replace() to remove HTML tags from a string:
php $html = "<p>Welcome <strong>to</strong> our <em>website</em></p>"; $pattern = "/<[^>]*>/"; $replacement = ""; $result = preg_replace($pattern, $replacement, $html); echo $result;
Output:
css Welcome to our website
In this example, the pattern /<[^>]*>/ matches any HTML tag and removes it.
5.2. Extracting specific data patterns
Imagine you have a string containing dates in the format “YYYY-MM-DD” and you want to extract only the years:
php $text = "Dates: 2022-01-15, 2019-09-08, 2023-05-20"; $pattern = "/(\d{4})-\d{2}-\d{2}/"; $replacement = "$1"; $result = preg_replace($pattern, $replacement, $text); echo $result;
Output:
yaml Dates: 2022, 2019, 2023
The pattern (\d{4})-\d{2}-\d{2} captures the year, and the replacement $1 keeps only the year.
5.3. URL beautification using regex
You can transform URLs for better readability:
php $url = "https://example.com/products.php?category=electronics"; $pattern = "/^(https:\/\/.+\/)([^?]+)(.*)$/"; $replacement = '<a href="$1$2">$2</a>'; $result = preg_replace($pattern, $replacement, $url); echo $result;
Output:
php <a href="https://example.com/products.php">products.php</a>?category=electronics
The pattern captures different parts of the URL, allowing you to create a link with a cleaner display text.
6. Limitations and Performance Considerations
6.1. When not to use preg_replace()
While powerful, preg_replace() might not be the best choice for every situation. It’s not recommended for tasks like simple string replacements, as the overhead of regex processing can be unnecessary.
6.2. Performance tips for efficient regex
- Specificity: Craft your patterns to be as specific as possible to avoid unnecessary matches.
- Limit Greedy Quantifiers: Greedy quantifiers like .* can lead to performance bottlenecks. Use non-greedy alternatives like .*? when necessary.
- Alternatives: In some cases, alternatives can slow down matching. Arrange them with the most likely matches first.
7. Best Practices
7.1. Writing readable and maintainable regex
Commenting: Use comments within your regex to explain complex patterns.
Whitespace: Utilize the x modifier to allow whitespace and comments, enhancing readability.
Break It Down: For complex patterns, break them into smaller subpatterns and combine them logically.
7.2. Testing and validating patterns
Online Tools: Use online regex testers to fine-tune your patterns before implementation.
Test Cases: Ensure your regex handles various test cases, including edge cases.
Conclusion
In conclusion, the preg_replace() function in PHP is a versatile and powerful tool for string
manipulation through regular expressions. Its ability to handle complex pattern matching and dynamic replacements makes it a valuable asset for developers facing various challenges. By mastering the art of preg_replace(), you can efficiently transform strings, extract data, and perform advanced text manipulations, opening up a world of possibilities for your PHP projects. Remember to practice, experiment, and refine your regex skills to fully leverage the capabilities of preg_replace() and become a regex ninja in your development journey.
Table of Contents
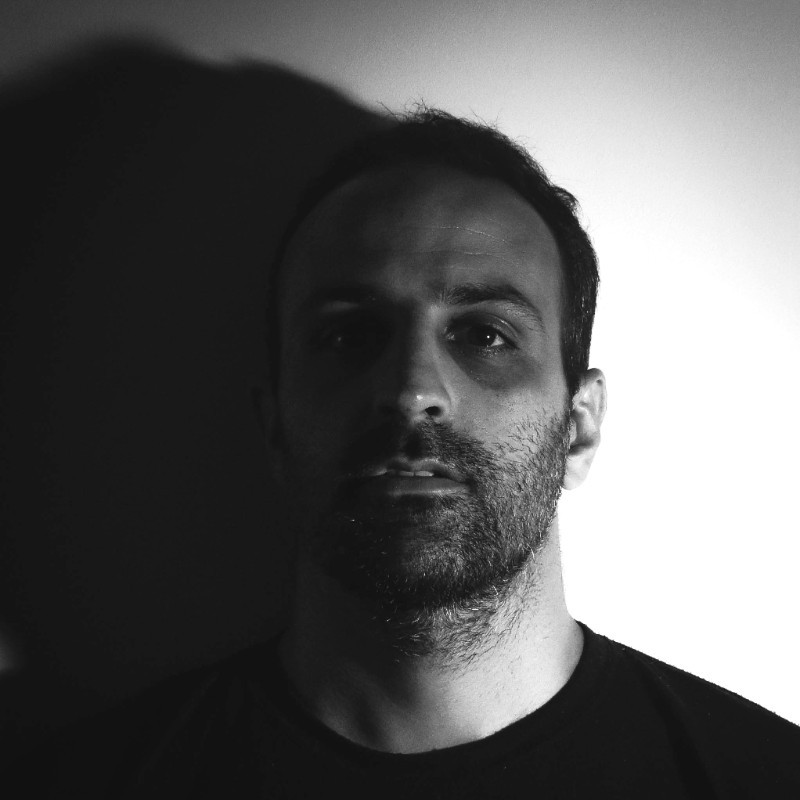
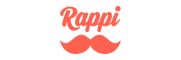