A Deep Dive into PHP’s printf() Function
PHP, a popular server-side scripting language, offers a wide array of functions to manipulate and format strings. Among these functions, printf() stands out as a powerful tool for formatting text output. In this comprehensive guide, we’ll take a deep dive into PHP’s printf() function, exploring its usage, format specifiers, and practical examples. By the end, you’ll have a solid understanding of how to leverage printf() to enhance your PHP applications.
Table of Contents
1. Understanding printf()
1.1. What is printf()?
printf() is a built-in PHP function primarily used for formatting and displaying text. It allows you to construct complex output strings by specifying placeholders for variables and defining how they should be displayed. This function is particularly useful for generating well-structured, human-readable text, such as reports, invoices, or any formatted content.
1.2. Basic Syntax
The basic syntax of printf() is as follows:
php printf(format_string, arg1, arg2, ...)
- format_string: A string that defines the format of the output.
- arg1, arg2, …: Variables or values to be inserted into the format string.
Let’s dive deeper into each aspect of the printf() function.
2. Format Specifiers
Format specifiers are placeholders within the format string that indicate where and how the corresponding values should be inserted and displayed. They start with a percent sign (%) followed by a character representing the data type or format of the variable. Here are some common format specifiers:
- %s: String
- %d or %i: Integer
- %f: Floating-point number
- %b: Binary
- %x or %X: Hexadecimal
- %c: Character
- %e or %E: Scientific notation
- %o: Octal
- %%: Literal percent sign
Example 1: Using %s for Strings
php $name = "John"; printf("Hello, %s!", $name);
Output:
Hello, John!
In this example, %s is used to insert the value of the $name variable into the format string.
Example 2: Using %d for Integers
php $age = 30; printf("I am %d years old.", $age);
Output:
css I am 30 years old.
Here, %d is used to display the integer value of $age within the text.
3. Specifying Width and Precision
Format specifiers can be customized further by specifying width and precision. Width defines the minimum number of characters the output should occupy, while precision defines the number of decimal places for floating-point numbers. These customizations are achieved by adding numbers to the format specifier.
Example 3: Specifying Width and Precision
php $price = 19.99; printf("The price is %10.2f dollars.", $price);
Output:
csharp The price is 19.99 dollars.
In this example, %10.2f is used, where 10 specifies the width (minimum characters) and .2 specifies the precision (two decimal places) of the floating-point number.
4. Formatting Flags
Formatting flags further enhance the control over the appearance of the output. They are added to the format specifier and affect how the data is displayed. Some commonly used formatting flags include:
- -: Left-justify the output.
- +: Display the sign of positive numbers.
- 0: Pad with leading zeros.
- #: Prefix with 0x for hexadecimal, 0 for octal, or include decimal point for floating-point.
- (space): Leave a space for positive numbers.
Example 4: Using Formatting Flags
php $number = 42; printf("Formatted number: %+08d", $number);
Output:
yaml Formatted number: +0000042
In this example, + is used as a formatting flag along with 08d, where 0 pads with leading zeros, and 8 specifies the width.
5. Multiple Format Specifiers
printf() allows you to use multiple format specifiers within a single format string to display multiple variables in a single output. The order of the arguments after the format string should match the order of the format specifiers.
Example 5: Using Multiple Format Specifiers
php $name = "Alice"; $age = 25; printf("Name: %s, Age: %d", $name, $age);
Output:
yaml Name: Alice, Age: 25
In this example, two variables ($name and $age) are inserted into the format string using %s and %d respectively.
6. Returning the Formatted String
By default, printf() displays the formatted string directly to the output. However, if you want to capture the formatted string in a variable instead of displaying it, you can use the sprintf() function. sprintf() works similarly to printf() but returns the formatted string instead.
Example 6: Using sprintf()
php $name = "Bob"; $formattedString = sprintf("Hello, %s!", $name); echo $formattedString;
Output:
Hello, Bob!
Here, sprintf() is used to create the formatted string, which is then stored in the $formattedString variable and later displayed using echo.
7. Practical Examples
Now that you have a good understanding of printf() and its various components, let’s explore some practical examples to see how it can be applied in real-world scenarios.
Example 7: Currency Formatting
php $amount = 1234.56; printf("Total: $%01.2f", $amount);
Output:
bash Total: $1234.56
In this example, we format a currency amount with a dollar sign, leading zeros, and two decimal places.
Example 8: Displaying Data in Columns
php $items = [ ["Item 1", 10.99], ["Item 2", 24.95], ["Item 3", 5.49], ]; foreach ($items as $item) { printf("%-15s %8.2f\n", $item[0], $item[1]); }
Output:
mathematica Item 1 10.99 Item 2 24.95 Item 3 5.49
Here, we use printf() to display a list of items with aligned columns for item names and prices.
Example 9: Displaying Dates
php $date = "2023-09-13"; $formattedDate = date("F j, Y", strtotime($date)); printf("Today's date is: %s", $formattedDate);
Output:
vbnet Today's date is: September 13, 2023
In this example, we format a date string into a more readable format using printf() and the date() function.
Conclusion
PHP’s printf() function is a versatile tool for formatting text output in a wide range of scenarios. By understanding format specifiers, width, precision, and formatting flags, you can harness the power of printf() to create well-structured, human-readable text in your PHP applications. Whether you need to format currency, display data in columns, or format dates, printf() provides the flexibility and control you need to achieve your desired output. So go ahead, experiment with different format strings, and elevate the quality of your PHP projects with this essential function.
Table of Contents
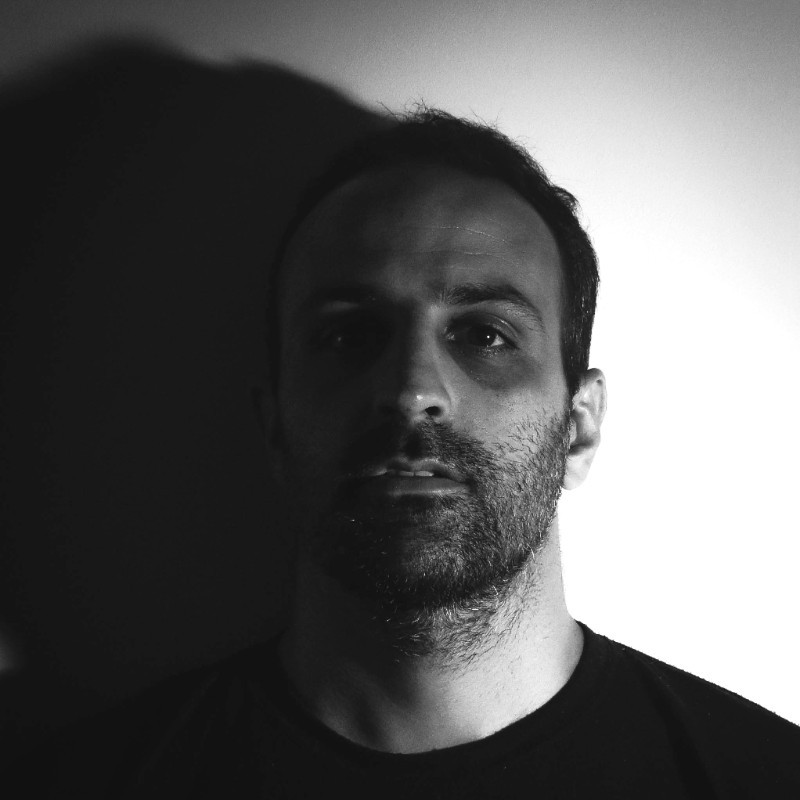
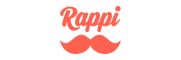