Exploring PHP’s rand() Function: Generating Random Numbers
Random numbers play a crucial role in programming for various applications, from generating unique identifiers to simulating chance-based events in games. PHP, being a versatile server-side scripting language, provides a built-in function called rand() to generate random numbers. In this blog post, we will dive deep into PHP’s rand() function, exploring its usage, features, limitations, and best practices.
Table of Contents
1. Introduction to rand() Function
PHP’s rand() function is a simple yet powerful tool for generating random numbers. It produces pseudo-random integers between 0 and a specified maximum value. This function does not require any external libraries, making it readily available for all PHP developers.
To start using rand(), you simply call the function without any arguments. Here’s a basic example:
php $randomNumber = rand(); echo $randomNumber;
This code will generate and print a random integer each time it is executed.
2. Generating Random Integers
By default, rand() generates random integers within the full range of a 32-bit signed integer. On most systems, this range is from -2,147,483,648 to 2,147,483,647. For many applications, this range is more than sufficient.
If you need to generate random integers within a specific range, you can pass two arguments to rand(). The first argument specifies the minimum value, and the second argument specifies the maximum value. Here’s an example:
php $min = 1; $max = 100; $randomNumber = rand($min, $max); echo $randomNumber;
This code will generate a random integer between 1 and 100, inclusive.
3. Generating Random Floats
While rand() primarily generates random integers, you can use it to generate random floats as well. To do this, you need to manipulate the result of rand() by dividing it by a range and adding an offset.
Here’s an example of generating a random float between 0 and 1:
php $randomFloat = rand() / getrandmax(); echo $randomFloat;
In this code, getrandmax() returns the maximum possible random integer value, and dividing rand() by it ensures the result is a floating-point number between 0 and 1.
4. Setting Minimum and Maximum Values
If you want more control over the precision and range of random float values, you can specify your own minimum and maximum values. Here’s an example:
php $min = 5.0; $max = 10.0; $randomFloat = ($min + lcg_value() * (abs($max - $min))); echo $randomFloat;
In this code, we’ve set the minimum and maximum values to 5.0 and 10.0, respectively, to generate random floats in that range.
5. Seeding Randomness with srand()
Pseudo-random number generators like rand() are deterministic, meaning they produce the same sequence of random numbers if given the same starting point, or seed. In most cases, this is desirable because it allows you to reproduce results when needed. However, there are situations where you may want to change the sequence of random numbers.
The srand() function allows you to set the seed for rand(), which can be useful in scenarios like randomizing content or creating different game outcomes on each run. Here’s how you can use srand():
php $seed = 42; // Your chosen seed value srand($seed); $randomNumber = rand(); echo $randomNumber;
By setting the seed to a specific value, you can ensure that subsequent calls to rand() will produce the same sequence of random numbers.
6. Common Use Cases for rand()
The rand() function finds applications in various domains of web development. Here are some common use cases:
6.1. Generating Random Passwords
Creating random passwords is essential for user security. You can use rand() to generate random characters for passwords. Here’s a simplified example:
php $length = 8; $password = ''; $characters = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789'; for ($i = 0; $i < $length; $i++) { $randomIndex = rand(0, strlen($characters) - 1); $password .= $characters[$randomIndex]; } echo $password;
This code generates an 8-character random password composed of letters (both uppercase and lowercase) and numbers.
6.2. Shuffling Arrays
Randomly shuffling the order of elements in an array can be useful in various scenarios, such as displaying randomized content or implementing game mechanics. PHP’s rand() can be employed to achieve this. Here’s a simple example:
php $items = ['A', 'B', 'C', 'D', 'E']; shuffle($items); foreach ($items as $item) { echo $item . ' '; }
This code shuffles the elements in the $items array, ensuring a random order each time it’s executed.
6.3. Simulating Dice Rolls
When creating games or simulations, you often need to simulate the roll of dice or other chance-based events. rand() is perfect for simulating dice rolls:
php $min = 1; $max = 6; $roll = rand($min, $max); echo "You rolled a $roll!";
This code simulates rolling a six-sided die, generating a random number between 1 and 6.
7. Best Practices for Using rand()
While rand() is a convenient function for generating random numbers, there are some best practices to keep in mind:
7.1. Use the random_int() Function for Cryptographic Purposes
If you need random numbers for cryptographic purposes, such as generating secure tokens or passwords, it’s recommended to use the random_int() function instead of rand(). random_int() provides cryptographically secure random integers and is less predictable than rand().
7.2. Be Cautious with Seeding
Seeding the random number generator can be useful, but it can also lead to predictable sequences if done carelessly. Avoid using easily guessable seed values in security-critical applications.
7.3. Avoid Overusing rand() in Loops
Generating random numbers in a loop can be computationally expensive, especially if the loop runs frequently. If possible, generate random numbers outside the loop and reuse them.
7.4. Consider Using External Libraries
For more advanced random number generation needs, consider using external libraries like random_compat or the random_bytes() function for cryptographic randomness.
Conclusion
PHP’s rand() function is a versatile tool for generating random numbers in a wide range of applications. Whether you’re creating random passwords, simulating dice rolls, or shuffling arrays, rand() can simplify the process. However, it’s crucial to use it appropriately and consider alternatives like random_int() for security-related tasks.
By understanding the capabilities and limitations of rand(), you can harness its power effectively in your PHP projects, adding a touch of randomness where needed. So go ahead and experiment with rand(), and let your code embrace the unpredictable world of random numbers.
Table of Contents
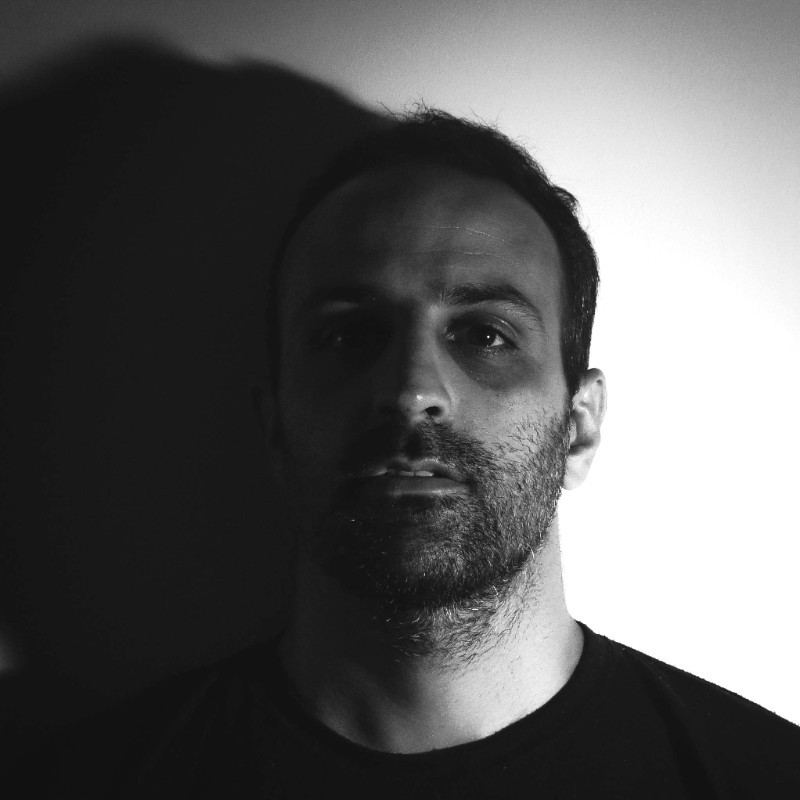
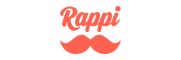