The Fundamentals of PHP’s range() Function
If you’re a PHP developer looking to efficiently generate numerical sequences, the range() function is a powerful tool at your disposal. It simplifies the process of creating arrays with sequential elements, making it an essential function for tasks such as looping, creating ranges of values, and more. In this comprehensive guide, we’ll delve deep into the fundamentals of PHP’s range() function, exploring its syntax, capabilities, and practical applications.
Table of Contents
1. Understanding the range() Function
Before diving into the intricacies of the range() function, let’s establish a basic understanding of what it is and what it does.
1.1. What is the range() Function?
The range() function in PHP is a built-in function used to create an array containing a range of elements. These elements can be integers, characters, or even dates. The function generates a sequential list of values, making it a handy tool for various programming tasks.
1.1.1. Syntax of the range() Function
The syntax for the range() function is straightforward:
php range($start, $end, $step);
- $start: The starting value of the sequence.
- $end: The end value of the sequence.
- $step (optional): The increment value. If not provided, it defaults to 1.
1.2. Generating a Simple Numeric Range
Let’s start with a basic example to generate a simple numeric range using the range() function:
php $numbers = range(1, 5); print_r($numbers);
In this example, we’ve created an array $numbers that contains a range of values from 1 to 5. When we print_r() the array, the output will be:
php Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 [4] => 5 )
As you can see, the range() function has generated a sequential array with values starting from 1 and ending at 5.
1.3. Specifying a Step Value
You can also specify a step value to control the increment between elements in the range. Here’s an example:
php $even_numbers = range(2, 10, 2); print_r($even_numbers);
In this code, we’ve generated an array of even numbers from 2 to 10 with a step of 2. The output will be:
php Array ( [0] => 2 [1] => 4 [2] => 6 [3] => 8 [4] => 10 )
By providing a step value of 2, we ensured that only even numbers were included in the array.
2. Practical Applications of the range() Function
Now that we’ve covered the basics, let’s explore some practical use cases for the range() function.
2.1. Looping Through a Range
One of the most common use cases for the range() function is looping through a range of values. Here’s an example of how you can use it in a for loop:
php foreach (range(1, 5) as $number) { echo $number . " "; }
This code will output:
1 2 3 4 5
2.2. Creating an Alphabet Array
You can also use the range() function to create an array containing the letters of the alphabet:
php $alphabet = range('A', 'Z'); print_r($alphabet);
The output will be:
php Array ( [0] => A [1] => B [2] => C [3] => D [4] => E [5] => F [6] => G [7] => H [8] => I [9] => J [10] => K [11] => L [12] => M [13] => N [14] => O [15] => P [16] => Q [17] => R [18] => S [19] => T [20] => U [21] => V [22] => W [23] => X [24] => Y [25] => Z )
This can be useful when you need to work with letters or characters in your code.
2.3. Generating Date Ranges
The range() function can even be used to generate date ranges. Suppose you want to create an array of dates for a specific month:
php $start_date = '2023-09-01'; $end_date = '2023-09-30'; $date_range = range($start_date, $end_date, '+1 day'); print_r($date_range);
In this example, we’ve created an array $date_range that includes all the dates from September 1, 2023, to September 30, 2023. The output will be a list of dates for the entire month.
3. Advanced Usage of the range() Function
While we’ve covered the basics and practical applications of the range() function, there are more advanced techniques you can employ.
3.1. Reversing a Range
You can easily reverse a range of values by providing the end value as lower than the start value. Here’s an example:
php $reverse_range = range(5, 1); print_r($reverse_range);
The output will be:
php Array ( [0] => 5 [1] => 4 [2] => 3 [3] => 2 [4] => 1 )
3.2. Using Negative Step Values
To count down or create a range in reverse order, you can use negative step values. For instance, let’s create a countdown from 10 to 1:
php $countdown = range(10, 1, -1); print_r($countdown);
The output will be:
php Array ( [0] => 10 [1] => 9 [2] => 8 [3] => 7 [4] => 6 [5] => 5 [6] => 4 [7] => 3 [8] => 2 [9] => 1 )
3.3. Combining Multiple Ranges
You can also combine multiple ranges into a single array using the array_merge() function. For instance, let’s create an array containing numbers from 1 to 5 and numbers from 10 to 15:
php $range1 = range(1, 5); $range2 = range(10, 15); $combined_range = array_merge($range1, $range2); print_r($combined_range);
The output will be:
php Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 [4] => 5 [5] => 10 [6] => 11 [7] => 12 [8] => 13 [9] => 14 [10] => 15 )
Conclusion
In this guide, we’ve explored the fundamentals of PHP’s range() function, from its basic syntax to its practical applications and advanced usage. Whether you need to generate numeric sequences, work with characters, or create date ranges, the range() function is a versatile tool that can simplify your coding tasks. By mastering this function, you’ll be better equipped to tackle a wide range of programming challenges in PHP. Start incorporating it into your projects and watch your code become more efficient and readable.
Table of Contents
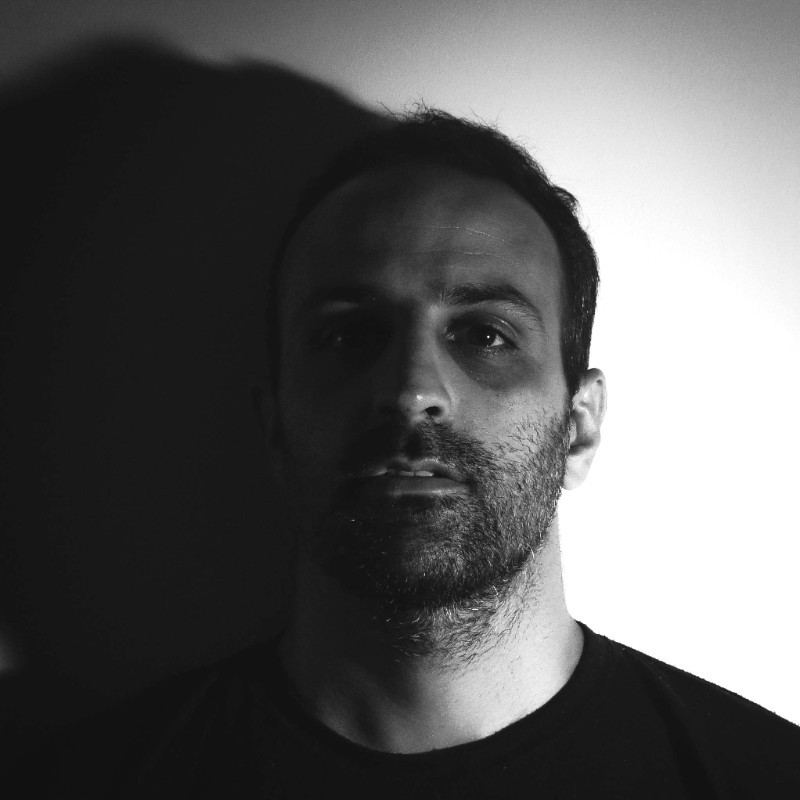
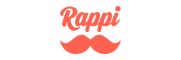