Demystifying Regular Expressions in PHP
Regular Expressions, commonly known as regex, are powerful tools for pattern matching and text manipulation. They provide a concise and flexible way to identify, extract, and replace specific patterns within strings. While they may seem intimidating at first, once you understand the basics and syntax, you’ll find that they can greatly simplify your code. In this blog post, we will demystify Regular Expressions in PHP, breaking them down step-by-step and providing practical examples along the way.
1. What is a Regular Expression?
At its core, a Regular Expression is a sequence of characters that defines a search pattern. The pattern can be as simple as a single word or more complex, involving various rules and constraints. Regular Expressions are widely used in programming languages, including PHP, for tasks such as data validation, parsing, and text manipulation.
2. Basic Syntax
In PHP, a Regular Expression is represented by a string and is enclosed between delimiters, typically forward slashes (/). For instance:
php $pattern = "/hello/";
In this example, the Regular Expression is “hello,” meaning it will search for the exact word “hello” within a string.
3. Matching Characters
Let’s start with the basic building blocks of Regular Expressions: matching characters. You can match a single character by simply including it in the pattern. For example:
php $pattern = "/a/"; // Will match 'a' in "apple"
To match a character at the beginning of a string, you can use the caret (^) symbol:
php $pattern = "/^h/"; // Will match 'h' in "hello"
And to match a character at the end of a string, you can use the dollar sign ($) symbol:
php $pattern = "/o$/"; // Will match 'o' in "hello"
4. Character Classes
Character classes allow you to match any one of a set of characters. You define a character class by enclosing the characters you want to match in square brackets ([]).
php $pattern = "/[aeiou]/"; // Will match any vowel in "hello"
You can also use a hyphen (-) to specify a range of characters:
php $pattern = "/[a-z]/"; // Will match any lowercase letter in "Hello"
To negate a character class, use a caret (^) symbol immediately after the opening bracket:
php $pattern = "/[^0-9]/"; // Will match any non-digit character in "abc123"
5. Metacharacters
Metacharacters are characters with a special meaning in Regular Expressions. Some of the common metacharacters include:
- . (period): Matches any single character, except for a newline.
- \d: Matches any digit. Equivalent to [0-9].
- \D: Matches any non-digit character. Equivalent to [^0-9].
- \w: Matches any word character (alphanumeric and underscore). Equivalent to [a-zA-Z0-9_].
- \W: Matches any non-word character. Equivalent to [^a-zA-Z0-9_].
- \s: Matches any whitespace character (spaces, tabs, newlines).
- \S: Matches any non-whitespace character.
php $pattern = "/\d/"; // Will match any digit in "abc123"
6. Quantifiers
Quantifiers specify how many times a character or group of characters can occur in a match. Some common quantifiers include:
- *: Matches zero or more occurrences of the preceding character/group.
- +: Matches one or more occurrences of the preceding character/group.
- ?: Matches zero or one occurrence of the preceding character/group.
- {n}: Matches exactly n occurrences of the preceding character/group.
- {n,}: Matches n or more occurrences of the preceding character/group.
- {n,m}: Matches between n and m occurrences of the preceding character/group.
php $pattern = "/\d+/"; // Will match any sequence of digits in "abc123def"
7. Greedy and Lazy Matching
By default, quantifiers are “greedy,” meaning they try to match as much as possible. If you want to perform a “lazy” match, add a question mark (?) after the quantifier.
php $pattern = "/<.*>/"; // Greedy match, will match "<div>content</div>" $pattern = "/<.*?>/"; // Lazy match, will match "<div>"
8. Capturing Groups
Capturing groups allow you to extract specific parts of a matched pattern. To create a capturing group, use parentheses (()).
php $pattern = "/(\d{2})-(\d{2})-(\d{4})/"; // Matches dates in the format dd-mm-yyyy preg_match($pattern, "Today's date is 17-07-2023", $matches);
The $matches array will now contain:
csharp Array ( [0] => 17-07-2023 [1] => 17 [2] => 07 [3] => 2023 )
9. Backreferences
Backreferences allow you to reference a captured group within the same pattern. They are denoted by backslashes followed by a number (\1, \2, etc.).
php $pattern = "/(\w+) and /"; // Matches repeated words, e.g., "apple and apple"
10. Modifiers
Modifiers in PHP are used after the closing delimiter of the Regular Expression to alter its behavior. Some common modifiers include:
- i: Case-insensitive matching.
- m: Treat the input as a multi-line string.
- s: Allow the dot (.) metacharacter to match newline characters as well.
- x: Ignore whitespace and allow comments within the Regular Expression.
- u: Use Unicode matching.
php $pattern = "/hello/i"; // Case-insensitive match for "hello", "Hello", "HELLO", etc.
11. Using Regular Expressions in PHP
In PHP, you can use Regular Expressions with the preg_match(), preg_match_all(), preg_replace(), and preg_split() functions. Let’s look at some examples:
11.1. preg_match()
php $pattern = "/hello/"; $string = "Say hello to the world!"; if (preg_match($pattern, $string)) { echo "Match found!"; } else { echo "No match found."; }
11.2. preg_match_all()
php $pattern = "/\d+/"; $string = "I have 3 cats and 5 dogs."; preg_match_all($pattern, $string, $matches); print_r($matches[0]);
11.3. preg_replace()
php $pattern = "/badword/"; $string = "This is a badword example."; $replacement = "***"; echo preg_replace($pattern, $replacement, $string);
11.4. preg_split()
php $pattern = "/\s+/"; $string = "This is a sentence."; $words = preg_split($pattern, $string); print_r($words);
Conclusion
Regular Expressions are a powerful tool that can save you time and effort in text processing tasks. Although they may seem complex at first, mastering the basics will open up a world of possibilities for your PHP projects. With the knowledge gained from this guide, you can confidently wield Regular Expressions to tackle various challenges and make your code more efficient and elegant.
So, go ahead, experiment, and harness the true potential of Regular Expressions in PHP! Happy coding!
Remember that practice makes perfect, so keep honing your Regular Expression skills, and don’t hesitate to refer back to this guide whenever you need a refresher. Happy coding!
Table of Contents
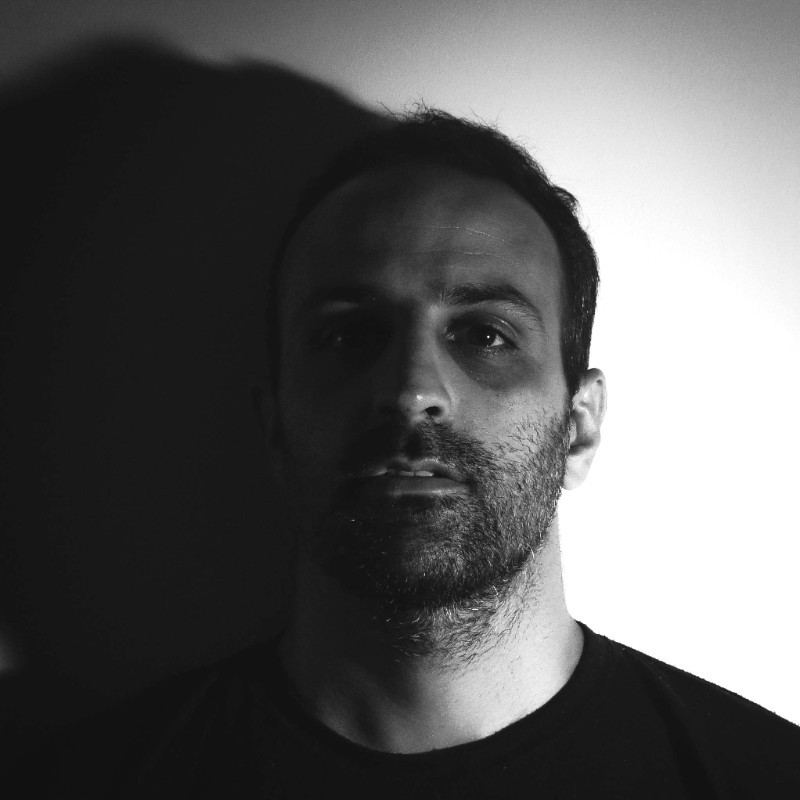
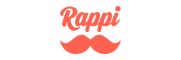