Understanding PHP’s Role in WordPress Development
When it comes to website development, WordPress stands as one of the most popular and versatile content management systems (CMS) available. Behind its user-friendly interface and vast array of themes and plugins lies a powerful programming language known as PHP (Hypertext Preprocessor). In this blog post, we will delve into the crucial role PHP plays in WordPress development, uncovering its core concepts and showcasing practical code samples. By gaining a deeper understanding of PHP’s capabilities, you’ll be empowered to leverage its potential to create dynamic, feature-rich websites on the WordPress platform.
What is PHP?
PHP is a server-side scripting language designed for web development. It was originally created by Rasmus Lerdorf in 1994 and has since evolved into a robust programming language widely used in website development. PHP is known for its simplicity, versatility, and wide-ranging support, making it an ideal choice for building dynamic websites.
One of the primary reasons PHP is favored in WordPress development is its seamless integration with the CMS. WordPress itself is built using PHP, making it the core programming language of the platform. This integration allows developers to leverage PHP’s extensive functionality to customize and extend WordPress in various ways.
PHP’s Integration with WordPress
WordPress, as mentioned earlier, is built using PHP. This means that the core files, plugins, and themes of WordPress are written in PHP. Understanding PHP is essential for comprehending how WordPress operates and for making the most out of the platform’s capabilities.
PHP code is responsible for dynamically generating the HTML and CSS that make up the web pages on a WordPress site. When a user requests a page, the PHP code executes on the server and produces the necessary content, which is then sent to the user’s browser. This dynamic nature enables WordPress websites to have flexible content, customizable layouts, and interactive features.
The WordPress Loop and PHP
The WordPress Loop is a fundamental concept in WordPress development, and it heavily relies on PHP. The Loop retrieves and displays posts from the database, allowing you to create dynamic pages that automatically update as new content is added.
Here’s an example of the basic structure of the WordPress Loop:
php <?php if ( have_posts() ) { while ( have_posts() ) { the_post(); // Display post content here } } ?>
In this code snippet, the have_posts() function checks if there are any posts to display, and the the_post() function retrieves each post, one at a time. You can then utilize PHP to format and display the post content, such as the title, date, author, and content itself.
Customizing Themes and Templates with PHP
WordPress themes define the appearance and layout of a website. They are a collection of PHP files that work together to generate the HTML and CSS for a site. By modifying the PHP code within a theme, developers can customize and tailor the look and feel of a WordPress website.
Let’s take a look at an example of customizing a theme’s template file using PHP:
php <?php // Custom code for the header section get_header(); // Custom code for the main content section if ( have_posts() ) { while ( have_posts() ) { the_post(); // Display post content here } } // Custom code for the sidebar section get_sidebar(); // Custom code for the footer section get_footer(); ?>
In this code snippet, you can see how PHP is used to include different template parts such as the header, main content, sidebar, and footer. By modifying the PHP code within these sections, developers can create unique and personalized WordPress themes.
Extending WordPress Functionality with PHP
WordPress provides a robust set of functions and APIs (Application Programming Interfaces) that allow developers to extend its core functionality. This is where PHP shines in WordPress development, as it enables you to create custom features, implement advanced functionality, and interact with various WordPress components.
Here’s an example of using PHP to create a custom function in WordPress:
php <?php function custom_hello_world() { echo 'Hello, World!'; } // Call the custom function custom_hello_world(); ?>
In this code snippet, we define a custom PHP function named custom_hello_world() that outputs the “Hello, World!” message. You can then call this function within your WordPress theme files or plugins to add new functionality to your website.
Creating Custom Plugins with PHP
WordPress plugins are packages of PHP code that extend the functionality of a WordPress site. They allow you to add new features, modify existing ones, integrate with external services, and more. PHP is at the core of plugin development, as it provides the means to interact with WordPress hooks, filters, and actions.
Consider the following example of a simple custom plugin in WordPress:
php <?php /* Plugin Name: My Custom Plugin Description: A brief description of the plugin. Version: 1.0 Author: Your Name */ function custom_plugin_function() { // Custom plugin code goes here } // Hook the custom function into a WordPress action add_action( 'wp_footer', 'custom_plugin_function' ); ?>
In this code snippet, we define a custom plugin named “My Custom Plugin.” The custom_plugin_function() is hooked into the wp_footer action, which means that the function will be executed when the footer of a WordPress page is rendered. This allows you to inject custom functionality seamlessly into different parts of your WordPress site.
Best Practices for PHP Development in WordPress
To ensure efficient and secure PHP development in WordPress, it’s crucial to follow best practices. Here are some key recommendations:
- Keep your PHP code clean, readable, and well-documented.
- Use proper indentation and coding standards, such as the WordPress Coding Standards.
- Sanitize and validate user input to prevent security vulnerabilities.
- Use appropriate error handling techniques to identify and resolve issues.
- Regularly update your PHP version to take advantage of performance improvements and security patches.
- By adhering to these best practices, you can enhance the quality, performance, and security of your WordPress website.
Conclusion
PHP is the backbone of WordPress development, playing a pivotal role in creating dynamic, feature-rich websites. Understanding PHP’s integration with WordPress, the WordPress Loop, theme customization, plugin development, and best practices is crucial for unlocking the full potential of the platform.
In this blog post, we’ve explored the core concepts of PHP in WordPress, along with practical code samples. Armed with this knowledge, you can confidently embark on your journey to create custom WordPress themes, plugins, and fully tailored websites that meet your specific requirements.
Remember, PHP is a powerful tool, and continuous learning and practice will empower you to push the boundaries of WordPress development and create exceptional online experiences. Happy coding!
Table of Contents
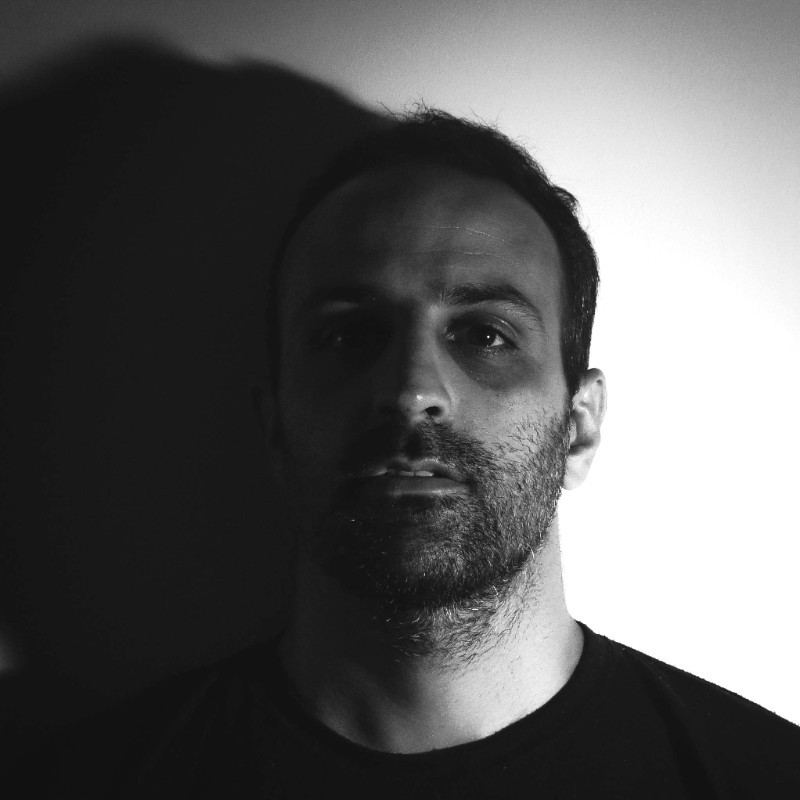
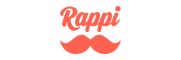