An In-depth Look into PHP’s round() Function
PHP is a versatile scripting language used for web development, and it offers a wide range of built-in functions to simplify common tasks. One such function is round(), which is essential for working with numbers in various applications. In this comprehensive guide, we will delve deep into PHP’s round() function, exploring its functionality, use cases, and practical examples.
Table of Contents
1. Understanding the Basics of the round() Function
Before we dive into the intricacies of the round() function, let’s start with the basics. The round() function is primarily used for rounding a floating-point number to the nearest integer, with the option to specify the number of decimal places to round to.
1.1. Syntax
Here’s the basic syntax of the round() function:
php round($number, $precision = 0, $mode = PHP_ROUND_HALF_UP);
- $number: The number you want to round.
- $precision (optional): The number of decimal places to round to (default is 0).
- $mode (optional): The rounding mode (default is PHP_ROUND_HALF_UP).
Now, let’s break down each parameter:
- $number: This is the value you want to round. It can be any numeric value, including a variable, constant, or the result of an expression.
- $precision: This parameter determines the number of decimal places to round to. If not specified, it defaults to 0, meaning the number will be rounded to the nearest integer.
- $mode: The rounding mode parameter specifies how to handle midpoints (values equidistant from the two nearest integers). PHP offers several predefined constants for rounding modes, including:
- PHP_ROUND_HALF_UP: Round halves up (default).
- PHP_ROUND_HALF_DOWN: Round halves down.
- PHP_ROUND_HALF_EVEN: Round halves to the nearest even integer.
- PHP_ROUND_HALF_ODD: Round halves to the nearest odd integer.
Now that we have a basic understanding of the round() function, let’s explore its various use cases and practical examples.
2. Rounding to the Nearest Integer
The most common use of the round() function is to round a number to the nearest integer. By default, if you don’t specify the $precision parameter, the function will round to the nearest integer.
Example 1: Basic Rounding
php $number = 3.7; $rounded = round($number); echo "Rounded value: $rounded"; // Output: Rounded value: 4
In this example, $number is rounded to the nearest integer, which is 4. The round() function takes care of rounding up when the decimal part is greater than or equal to 0.5 and rounding down when it’s less than 0.5.
3. Rounding to a Specific Decimal Place
Sometimes, you may need to round a number to a specific number of decimal places. You can achieve this by providing the $precision parameter.
Example 2: Rounding to Two Decimal Places
php $number = 7.896; $rounded = round($number, 2); echo "Rounded value: $rounded"; // Output: Rounded value: 7.9
In this example, we round $number to two decimal places, resulting in 7.9. The round() function takes care of rounding up or down as needed to achieve the specified precision.
4. Choosing the Rounding Mode
The choice of rounding mode can have a significant impact on the result when rounding numbers. Let’s explore different rounding modes using the round() function.
Example 3: Rounding Down
php $number = 3.5; $rounded = round($number, 0, PHP_ROUND_HALF_DOWN); echo "Rounded value: $rounded"; // Output: Rounded value: 3
In this example, we specify the rounding mode as PHP_ROUND_HALF_DOWN, which rounds down when the decimal part is exactly 0.5. So, 3.5 is rounded down to 3.
Example 4: Rounding Half to Even
php $number = 2.5; $rounded = round($number, 0, PHP_ROUND_HALF_EVEN); echo "Rounded value: $rounded"; // Output: Rounded value: 2
Here, we use the PHP_ROUND_HALF_EVEN mode, which rounds halves to the nearest even integer. As a result, 2.5 is rounded to 2.
Example 5: Rounding Half to Odd
php $number = 2.5; $rounded = round($number, 0, PHP_ROUND_HALF_ODD); echo "Rounded value: $rounded"; // Output: Rounded value: 3
In contrast to the previous example, we use the PHP_ROUND_HALF_ODD mode, which rounds halves to the nearest odd integer. So, 2.5 is rounded to 3.
5. Dealing with Negative Numbers
The round() function can also handle negative numbers and decimal places with ease.
Example 6: Rounding Negative Numbers
php $number = -2.75; $rounded = round($number, 1); echo "Rounded value: $rounded"; // Output: Rounded value: -2.8
In this case, we round a negative number to one decimal place, resulting in -2.8. The function correctly handles negative values and performs the rounding as expected.
6. Rounding with Variables and Expressions
The round() function is not limited to rounding static numbers; you can use it with variables and expressions as well.
Example 7: Rounding with Variables
php $price = 19.99; $discount = 0.15; $finalPrice = round($price - ($price * $discount), 2); echo "Final price after discount: $finalPrice"; // Output: Final price after discount: 16.99
In this example, we calculate the final price after applying a discount to a product’s price. The round() function ensures that the final price is rounded to two decimal places.
Example 8: Rounding Expressions
php $total = 23.75; $taxRate = 0.0875; $taxAmount = round($total * $taxRate, 2); echo "Tax amount: $taxAmount"; // Output: Tax amount: 2.08
Here, we calculate the tax amount by multiplying the total by the tax rate. The round() function is used to round the tax amount to two decimal places.
7. Handling Special Cases
In some cases, you might need to handle specific rounding scenarios. Let’s explore a few examples of such scenarios.
Example 9: Rounding Up Always
php $number = 5.001; $roundedUp = round($number, 0, PHP_ROUND_HALF_UP); echo "Rounded up: $roundedUp"; // Output: Rounded up: 6
If you want to always round up, regardless of the decimal part, you can use the PHP_ROUND_HALF_UP mode. In this example, 5.001 is rounded up to 6.
Example 10: Rounding Down Always
php $number = 7.999; $roundedDown = round($number, 0, PHP_ROUND_HALF_DOWN); echo "Rounded down: $roundedDown"; // Output: Rounded down: 7
Conversely, if you want to always round down, even if the decimal part is greater than or equal to 0.5, you can use the PHP_ROUND_HALF_DOWN mode. Here, 7.999 is rounded down to 7.
Conclusion
In this in-depth exploration of PHP’s round() function, we’ve learned how to round numbers effectively, handle decimal precision, and choose the appropriate rounding mode for different scenarios. Whether you’re working with financial calculations, data analysis, or any application requiring precise number rounding, the round() function is a valuable tool in your PHP toolkit. By mastering this function, you can ensure your code produces accurate results and meets the requirements of your projects.
PHP’s round() function is just one of many powerful functions in the language. As you continue to develop your PHP skills, you’ll discover even more ways to streamline your code and achieve your programming goals with ease. Happy coding!
Table of Contents
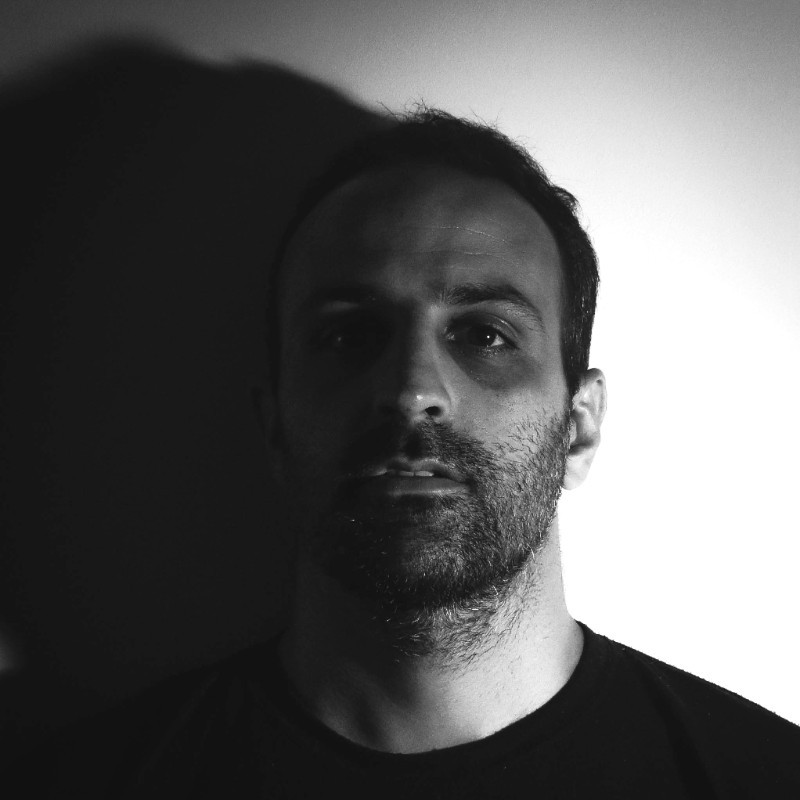
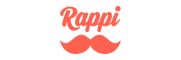