How to send emails?
Sending emails in PHP is a common task for web applications, whether it’s for user registration, notifications, or other communication. To send emails from your PHP application, you can use the built-in `mail()` function or a third-party library like PHPMailer for more advanced features and better control over the sending process. Here’s a basic guide on how to send emails using the `mail()` function:
- Configure Your SMTP Server (if not using `mail()`):
– If you’re not using the `mail()` function, you’ll need to configure an SMTP (Simple Mail Transfer Protocol) server. You can use a local or remote SMTP server like Gmail or SendGrid. Gather the SMTP server address, port, and authentication credentials.
- Set Up the Email Content:
– Create the email content, including the subject, message body, and sender/receiver email addresses. You can use HTML to format the email content.
```php $to = "recipient@example.com"; $subject = "Subject of your email"; $message = "<html><body><p>Hello, this is the message body.</p></body></html>"; $headers = "From: sender@example.com\r\n"; $headers .= "Reply-To: sender@example.com\r\n"; $headers .= "Content-Type: text/html; charset=UTF-8\r\n"; ```
- Send the Email:
– Use the `mail()` function to send the email. Specify the recipient’s email address, subject, message, and headers.
```php $mailSent = mail($to, $subject, $message, $headers); if ($mailSent) { echo "Email sent successfully!"; } else { echo "Email sending failed."; } ```
- SMTP Configuration (if using a library):
– If you opt for a library like PHPMailer, configure the library to use your SMTP server. This provides more flexibility and control over email sending, including support for attachments and advanced features.
```php $mail = new PHPMailer; $mail->isSMTP(); $mail->Host = 'smtp.example.com'; $mail->SMTPAuth = true; $mail->Username = 'your_username'; $mail->Password = 'your_password'; $mail->SMTPSecure = 'tls'; $mail->Port = 587; ```
- Send the Email (with PHPMailer):
– With PHPMailer, sending an email is straightforward:
```php $mail->setFrom('sender@example.com', 'Sender Name'); $mail->addAddress('recipient@example.com', 'Recipient Name'); $mail->Subject = 'Subject of your email'; $mail->Body = 'Hello, this is the message body in HTML format.'; if ($mail->send()) { echo 'Email sent successfully!'; } else { echo 'Email sending failed.'; } ```
By following these steps, you can send emails from your PHP application using the `mail()` function or a library like PHPMailer, allowing you to efficiently integrate email communication into your web application.
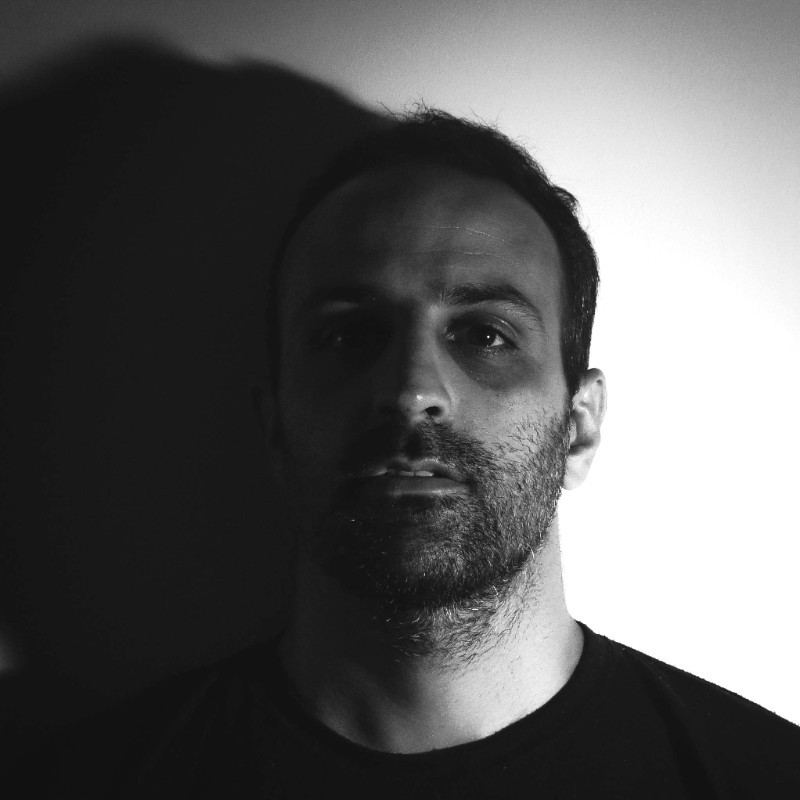
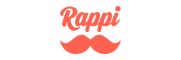