How to Leverage PHP’s setcookie() Function
When it comes to building dynamic and interactive web applications, managing user sessions and storing user preferences are crucial aspects. PHP, as a widely used server-side scripting language, offers a range of functions to handle these tasks effectively. One such function is setcookie(), which allows developers to set cookies on the client’s browser. In this guide, we will delve into the details of PHP’s setcookie() function, its applications, and best practices to leverage its potential for enhancing your web applications.
1. Understanding Cookies:
Before we dive into the specifics of setcookie(), it’s essential to understand what cookies are and why they are useful. Cookies are small pieces of data that a web server can send to a user’s browser. These cookies are then stored on the user’s computer and can be accessed by the server on subsequent requests. Cookies are commonly used for various purposes, such as session management, user authentication, and storing user preferences.
2. Using PHP’s setcookie() Function:
The setcookie() function in PHP enables developers to set cookies with various parameters. Let’s explore the basic syntax of the function:
php setcookie(name, value, expire, path, domain, secure, httponly);
- name: The name of the cookie.
- value: The value to be stored in the cookie.
- expire: The expiration time of the cookie (in Unix timestamp format).
- path: The path on the server where the cookie is available.
- domain: The domain associated with the cookie.
- secure: If true, the cookie should only be transmitted over secure HTTPS connections.
- httponly: If true, the cookie cannot be accessed through JavaScript.
3. Setting a Basic Cookie:
Let’s start with a simple example of setting a cookie using the setcookie() function:
php $name = "user"; $value = "John"; $expire = time() + 3600; // Cookie will expire in 1 hour $path = "/"; $domain = "example.com"; $secure = false; $httponly = true; setcookie($name, $value, $expire, $path, $domain, $secure, $httponly);
In this example, we’ve set a cookie named “user” with the value “John.” The cookie will expire in one hour and is accessible across the entire domain.
4. Managing User Sessions:
One of the most common applications of cookies is managing user sessions. Cookies can be used to maintain user sessions and keep users logged in across different pages of a website. Let’s see how the setcookie() function can be used for session management:
php // Start a session session_start(); // Set a session cookie $userID = 123; $expire = time() + 3600; // Expiring in 1 hour $path = "/"; $domain = "example.com"; setcookie("sessionID", $userID, $expire, $path, $domain);
In this example, we’ve used the session_start() function to initiate a session. Then, we set a cookie named “sessionID” containing the user’s ID, which can be used to identify the session on subsequent requests. This enables the user to stay logged in for the specified duration.
5. Enhancing User Experience with Preferences:
Cookies can also be employed to enhance user experience by storing user preferences or settings. For instance, consider a website where users can customize the color theme. Cookies can be used to remember the chosen theme and apply it consistently. Here’s how you can implement this:
php // Assuming the user selected a theme $selectedTheme = "dark"; // Set a cookie for the selected theme $expire = time() + 604800; // Expiring in 1 week $path = "/"; $domain = "example.com"; setcookie("theme", $selectedTheme, $expire, $path, $domain);
In this example, the chosen theme is stored in a cookie named “theme.” The cookie will expire in one week, ensuring that the user’s preferred theme persists across visits.
6. Best Practices for Using setcookie():
While setcookie() offers great flexibility, it’s essential to follow best practices to ensure proper functionality and security:
- Sanitize Data: Always sanitize user input before using it in cookies to prevent potential security vulnerabilities.
- Use HTTPS: When dealing with sensitive information, set the “secure” parameter to true, ensuring the cookie is transmitted only over secure connections.
- Limit Sensitive Data: Avoid storing sensitive information like passwords in cookies. Instead, store references or tokens and validate them on the server side.
- Be Mindful of Expiry: Set appropriate expiry times for cookies. Use shorter expiry times for sensitive data and longer ones for user preferences.
- Clear Unused Cookies: Regularly clean up cookies that are no longer necessary to prevent clutter and potential privacy concerns.
Conclusion
PHP’s setcookie() function is a powerful tool for managing user sessions, storing preferences, and enhancing the user experience in web applications. By understanding its parameters and best practices, you can leverage cookies effectively to create more interactive and personalized websites. Whether you’re building an e-commerce platform, a social networking site, or a content-based app, the setcookie() function can play a pivotal role in providing a seamless and customized user experience. Remember to implement security measures and adhere to best practices to ensure that your cookie-based functionalities are robust and secure.
Table of Contents
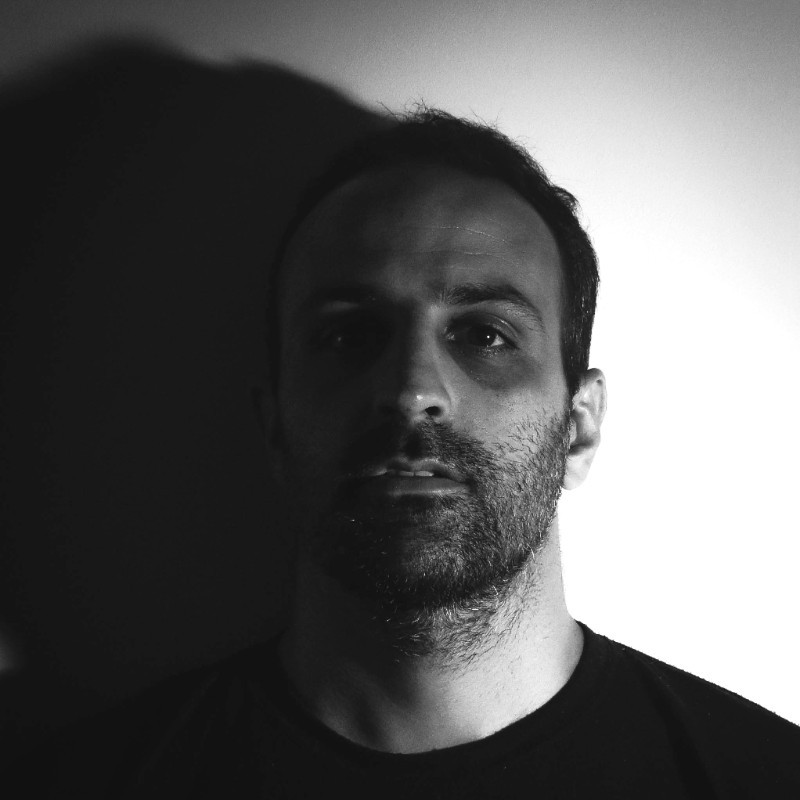
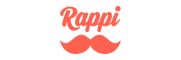