Using PHP to Develop a Simple CRUD Application
In the world of web development, CRUD (Create, Read, Update, Delete) operations are fundamental to managing data within applications. Whether it’s a blog, an e-commerce platform, or a content management system, CRUD functionality is essential for allowing users to interact with data stored in a database. PHP, a widely used server-side scripting language, is an excellent choice for building such applications due to its simplicity, flexibility, and robustness.
In this tutorial, we’ll guide you through the process of creating a simple CRUD application using PHP. By the end, you’ll have a functional web application that can perform basic database operations with ease. We’ll break down each step, providing explanations and code samples to ensure a smooth learning experience.
1. Prerequisites
Before we dive into building our CRUD application, let’s ensure you have the necessary tools and knowledge:
- Basic understanding of PHP and MySQL.
- A local development environment with PHP and MySQL installed.
- A text editor or integrated development environment (IDE) for coding.
2. Setting Up the Project
Step 1: Database Configuration
Our first task is to set up the database that will store the data for our CRUD application. Start by creating a new database in MySQL using phpMyAdmin or any other MySQL management tool. For this tutorial, we’ll name our database “crud_app.”
Next, we need a table to store our data. Let’s create a table named “users” to manage user information. This table will have the following columns:
- id (auto-incremented)
- name (for storing user names)
- email (for storing user emails)
- created_at (timestamp for record creation)
- updated_at (timestamp for record update)
Here’s the SQL query to create the “users” table:
sql CREATE TABLE users ( id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(255) NOT NULL, email VARCHAR(255) NOT NULL, created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP, updated_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP );
Step 2: Project Structure
Let’s set up the project structure. Create the following folders and files within your project directory:
markdown - crud_app - assets - css - style.css - js - script.js - includes - db_connection.php - index.php - create.php - read.php - update.php - delete.php
In this structure, the “assets” folder will contain CSS and JavaScript files, while the “includes” folder will store a PHP file for handling database connections.
Step 3: Establish Database Connection
To interact with the database, we need to establish a connection. Create a file named “db_connection.php” inside the “includes” folder and add the following code:
php <?php $servername = "localhost"; $username = "your_mysql_username"; $password = "your_mysql_password"; $dbname = "crud_app"; // Create connection $conn = new mysqli($servername, $username, $password, $dbname); // Check connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); }
Replace your_mysql_username and your_mysql_password with your actual MySQL credentials.
Step 4: Styling the Application
For the sake of simplicity, we’ll use basic CSS to style our application. Create a “style.css” file inside the “css” folder and add the following styles:
css /* style.css */ body { font-family: Arial, sans-serif; line-height: 1.6; margin: 0; padding: 0; } .container { max-width: 800px; margin: 0 auto; padding: 20px; } h1 { text-align: center; margin-bottom: 20px; } form { display: flex; flex-direction: column; max-width: 400px; margin: 0 auto; } input, button { margin-bottom: 10px; padding: 10px; } button { cursor: pointer; background-color: #007bff; color: #fff; border: none; } button:hover { background-color: #0056b3; }
With this CSS, our application will have a clean and responsive layout.
3. Building the CRUD Application
Now that we’ve set up the project structure and database connection, let’s proceed to build the CRUD application. We’ll implement each CRUD operation in separate PHP files for better organization and maintenance.
Step 1: Creating Records (Create)
In this step, we’ll create the “create.php” file to add new records to our database.
php // create.php <?php include "includes/db_connection.php"; if ($_SERVER["REQUEST_METHOD"] === "POST") { $name = $_POST["name"]; $email = $_POST["email"]; $sql = "INSERT INTO users (name, email) VALUES ('$name', '$email')"; if ($conn->query($sql) === TRUE) { header("Location: index.php"); } else { echo "Error: " . $sql . "<br>" . $conn->error; } $conn->close(); } ?> <!DOCTYPE html> <html> <head> <title>CRUD Application - Create</title> <link rel="stylesheet" href="assets/css/style.css"> </head> <body> <div class="container"> <h1>Create New User</h1> <form method="post"> <input type="text" name="name" placeholder="Name" required> <input type="email" name="email" placeholder="Email" required> <button type="submit">Create User</button> </form> </div> </body> </html>
In this code, we’ve created a form that accepts user input for name and email. When the form is submitted, the data is sent to the same “create.php” file using the POST method. The PHP code processes the form data and inserts it into the “users” table. If the operation is successful, the user is redirected to the “index.php” page (which we’ll create later), and if an error occurs, it is displayed on the page.
Step 2: Reading Records (Read)
Now, let’s create the “read.php” file to display all the records from the database in a tabular format.
php // read.php <?php include "includes/db_connection.php"; $sql = "SELECT * FROM users"; $result = $conn->query($sql); $users = array(); if ($result->num_rows > 0) { while ($row = $result->fetch_assoc()) { $users[] = $row; } } $conn->close(); ?> <!DOCTYPE html> <html> <head> <title>CRUD Application - Read</title> <link rel="stylesheet" href="assets/css/style.css"> </head> <body> <div class="container"> <h1>Users List</h1> <table> <tr> <th>ID</th> <th>Name</th> <th>Email</th> <th>Actions</th> </tr> <?php foreach ($users as $user): ?> <tr> <td><?php echo $user["id"]; ?></td> <td><?php echo $user["name"]; ?></td> <td><?php echo $user["email"]; ?></td> <td> <a href="update.php?id=<?php echo $user["id"]; ?>">Edit</a> <a href="delete.php?id=<?php echo $user["id"]; ?>">Delete</a> </td> </tr> <?php endforeach; ?> </table> </div> </body> </html>
In this code, we first retrieve all the records from the “users” table using a SELECT query. The fetched data is stored in the $users array. We then display the data in an HTML table with columns for ID, name, email, and actions (edit and delete links).
Step 3: Updating Records (Update)
Next, we’ll create the “update.php” file to allow users to edit existing records.
php // update.php <?php include "includes/db_connection.php"; if ($_SERVER["REQUEST_METHOD"] === "GET" && isset($_GET["id"])) { $id = $_GET["id"]; $sql = "SELECT * FROM users WHERE id = $id"; $result = $conn->query($sql); if ($result->num_rows == 1) { $user = $result->fetch_assoc(); } else { echo "User not found."; } $conn->close(); } if ($_SERVER["REQUEST_METHOD"] === "POST") { $id = $_POST["id"]; $name = $_POST["name"]; $email = $_POST["email"]; $sql = "UPDATE users SET name = '$name', email = '$email' WHERE id = $id"; if ($conn->query($sql) === TRUE) { header("Location: index.php"); } else { echo "Error: " . $sql . "<br>" . $conn->error; } $conn->close(); } ?> <!DOCTYPE html> <html> <head> <title>CRUD Application - Update</title> <link rel="stylesheet" href="assets/css/style.css"> </head> <body> <div class="container"> <h1>Edit User</h1> <form method="post"> <input type="hidden" name="id" value="<?php echo $user["id"]; ?>"> <input type="text" name="name" value="<?php echo $user["name"]; ?>" required> <input type="email" name="email" value="<?php echo $user["email"]; ?>" required> <button type="submit">Update User</button> </form> </div> </body> </html>
In this code, we handle two scenarios: displaying the existing user data in the form for editing (when the page is accessed via GET with the “id” parameter) and updating the user data in the database when the form is submitted via POST.
Step 4: Deleting Records (Delete)
Lastly, let’s create the “delete.php” file to allow users to delete records from the database.
php // delete.php <?php include "includes/db_connection.php"; if ($_SERVER["REQUEST_METHOD"] === "GET" && isset($_GET["id"])) { $id = $_GET["id"]; $sql = "DELETE FROM users WHERE id = $id"; if ($conn->query($sql) === TRUE) { header("Location: index.php"); } else { echo "Error deleting record: " . $conn->error; } $conn->close(); } ?>
In this code, we handle the deletion of a user record when the “id” parameter is passed via GET. The corresponding record is deleted from the “users” table, and the user is redirected to the “index.php” page.
Conclusion
Congratulations! You’ve successfully built a simple CRUD application using PHP. Throughout this tutorial, you’ve learned how to set up the project, establish a database connection, and perform basic CRUD operations: create, read, update, and delete. By applying this knowledge, you can now create more sophisticated web applications with advanced database interactions.
Remember that this is just the beginning of your PHP journey. As you continue to develop your skills, you’ll be able to build more complex applications and explore the vast world of web development.
Table of Contents
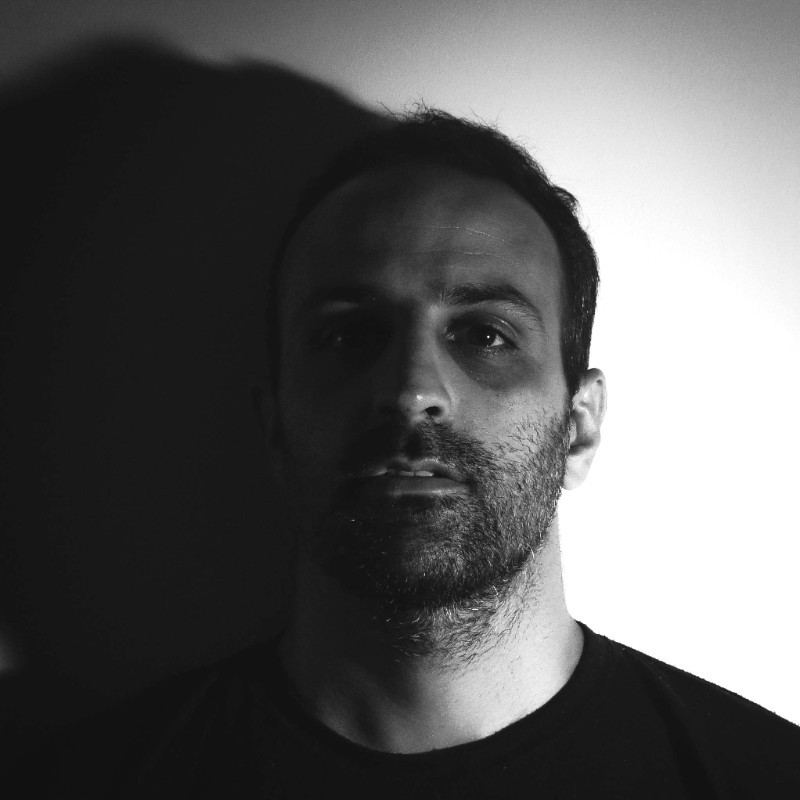
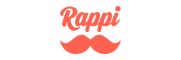