The Intricacies of PHP’s sprintf() Function
PHP is a versatile programming language known for its wide range of functions and features that make developers’ lives easier. One such function that deserves attention is sprintf(). While it may seem straightforward at first glance, diving deeper into its intricacies reveals its true power.
Table of Contents
In this blog post, we’ll explore the sprintf() function, dissect its syntax, and delve into various use cases with code samples. By the end of this journey, you’ll have a comprehensive understanding of how to harness the full potential of sprintf() in your PHP projects.
1. Understanding sprintf() Basics
Before we dive into the intricacies, let’s start with the basics. At its core, sprintf() is a function used for formatting strings. It allows you to create formatted strings by inserting values into a placeholder within a template string. The template string contains placeholders marked by percent signs followed by a format specifier.
Here’s the basic syntax of sprintf():
php string sprintf(string $format, mixed ...$args)
- $format: The template string with placeholders.
- $args: An array or a list of values to be inserted into the placeholders.
To illustrate, let’s look at a simple example:
php $name = "John"; $age = 30; $message = sprintf("Hello, my name is %s, and I am %d years old.", $name, $age); echo $message;
In this example, %s and %d are placeholders for a string and an integer, respectively. The corresponding values $name and $age are inserted into these placeholders using sprintf(), resulting in the formatted string: “Hello, my name is John, and I am 30 years old.”
Now that we have a basic understanding, let’s dive into the intricacies of sprintf().
2. Format Specifiers
The real power of sprintf() lies in its format specifiers. Format specifiers define the type of data that should be inserted into the placeholder and allow you to control how that data is formatted within the string. Here are some commonly used format specifiers:
- %s: Insert a string.
- %d: Insert an integer.
- %f: Insert a floating-point number.
- %x: Insert an integer in hexadecimal format.
- %b: Insert an integer in binary format.
Let’s explore these format specifiers in action:
2.1. Inserting Strings (%s)
php $name = "Alice"; $message = sprintf("Hello, %s!", $name); echo $message; // Output: "Hello, Alice!"
2.2. Inserting Integers (%d)
php $quantity = 5; $message = sprintf("You have %d apples.", $quantity); echo $message; // Output: "You have 5 apples."
2.3. Inserting Floating-Point Numbers (%f)
php $price = 19.99; $message = sprintf("The price is $%.2f.", $price); echo $message; // Output: "The price is $19.99."
2.4. Inserting Hexadecimal Numbers (%x)
php $hexValue = 255; $message = sprintf("The hexadecimal representation is %x.", $hexValue); echo $message; // Output: "The hexadecimal representation is ff."
2.5. Inserting Binary Numbers (%b)
php $binaryValue = 9; $message = sprintf("The binary representation is %b.", $binaryValue); echo $message; // Output: "The binary representation is 1001."
These examples demonstrate the flexibility and versatility of sprintf() when it comes to formatting different types of data within strings.
3. Precision and Width
Format specifiers can be customized further with precision and width parameters. These parameters allow you to control the number of decimal places for floating-point numbers or specify the minimum width for strings and integers.
3.1. Precision for Floating-Point Numbers
You can specify the number of decimal places for floating-point numbers using the precision parameter. It follows the format specifier and is represented as a period (.) followed by a number.
php $pi = 3.14159265359; $message = sprintf("The value of pi is approximately %.2f.", $pi); echo $message; // Output: "The value of pi is approximately 3.14."
In this example, %.2f specifies that the floating-point number should have two decimal places.
3.2. Minimum Width for Strings and Integers
You can also specify a minimum width for strings and integers using the width parameter. It’s represented as a number that appears before the format specifier. This is particularly useful when aligning values within a formatted string.
php $code = "ABC123"; $message = sprintf("Product Code: %10s", $code); echo $message; // Output: "Product Code: ABC123"
In this example, %10s specifies that the string should be at least 10 characters wide, padding with spaces if necessary.
4. Mixing Format Specifiers
What makes sprintf() even more powerful is the ability to mix and match format specifiers within a single template string. This allows you to create complex formatted strings with ease.
php $product = "Widget"; $quantity = 3; $price = 19.99; $total = $quantity * $price; $message = sprintf("You ordered %d %s%s for a total of $%.2f.", $quantity, $product, ($quantity > 1 ? 's' : ''), $total); echo $message; // Output: "You ordered 3 Widgets for a total of $59.97."
In this example, we combine integers, strings, and floating-point numbers within the same string using different format specifiers, making it easy to generate dynamic output.
5. Controlling Alignment
To enhance the visual appeal of your formatted strings, you can control the alignment of values within the placeholders. The alignment is controlled by adding a plus sign (+) or a minus sign (-) before the width specifier.
- +: Forces the sign (+ or -) to be displayed before positive and negative numbers.
- -: Left-aligns the value within the specified width.
Let’s see these alignment options in action:
5.1. Aligning Numbers with Signs
php $positive = 42; $negative = -17; $message1 = sprintf("Positive: %+5d", $positive); $message2 = sprintf("Negative: %+5d", $negative); echo $message1; // Output: "Positive: +42" echo $message2; // Output: "Negative: -17"
In this example, the %+5d format specifier ensures that the positive number is displayed with a plus sign, and both numbers are right-aligned within a width of 5 characters.
5.2. Left-Aligning Text
php $leftAligned = "Left Align"; $message = sprintf("Text: %-15s", $leftAligned); echo $message; // Output: "Text: Left Align "
Here, the %-15s format specifier left-aligns the text within a width of 15 characters.
6. Handling Dynamic Placeholder Counts
In some cases, you might have a dynamic number of placeholders in your template string. Fortunately, sprintf() can handle this scenario by accepting an array of values instead of individual arguments. This is particularly useful when generating strings dynamically based on user inputs or database queries.
php $placeholders = ["Alice", 25, "developer"]; $template = "Hello, my name is %s, I am %d years old, and I work as a %s."; $message = vsprintf($template, $placeholders); echo $message; // Output: "Hello, my name is Alice, I am 25 years old, and I work as a developer."
Here, vsprintf() is used with an array of values, allowing you to generate the formatted string without knowing the exact number of placeholders in advance.
7. Error Handling
When using sprintf(), it’s essential to be cautious about the number of placeholders and the number of values provided. Mismatched counts can lead to unexpected behavior or errors.
If you provide too few values, you’ll likely encounter warnings or notices, depending on your PHP error reporting settings. If you provide too many values, the extra values are ignored.
To mitigate these issues, you can use error suppression with @ to suppress warnings and notices. However, it’s generally a better practice to ensure that your template strings and values match correctly.
Conclusion
PHP’s sprintf() function is a versatile tool for formatting strings in a wide range of ways. By mastering format specifiers, precision, width, alignment, and dynamic placeholders, you can create well-formatted and visually appealing output for your PHP applications.
Whether you’re generating dynamic reports, building user-friendly interfaces, or formatting data for storage, sprintf() is a valuable addition to your PHP toolkit. Understanding its intricacies allows you to harness its full potential and make your PHP code more efficient and readable.
Table of Contents
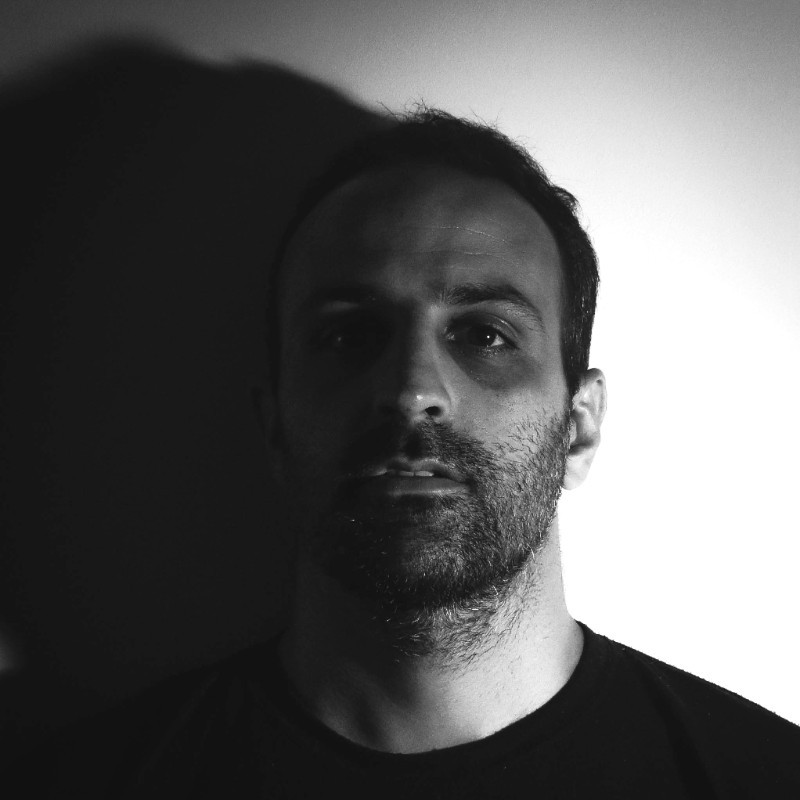
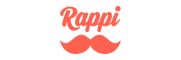