PHP’s str_repeat() Function: Creating Patterns with Strings
Strings are fundamental to programming, and PHP offers a versatile set of tools to manipulate them. One such tool that can be incredibly useful in various scenarios is the str_repeat() function. This function allows you to create intricate patterns within strings by repeating a given substring multiple times. In this comprehensive guide, we’ll explore the ins and outs of the str_repeat() function, its syntax, use cases, and provide you with practical code examples.
Table of Contents
1. Understanding the str_repeat() Function
Before we dive into the details, let’s get a clear understanding of what the str_repeat() function does. In essence, it takes two arguments:
- Input String: This is the base string that you want to repeat a specific number of times.
- Number of Repetitions: The number of times you want the input string to be repeated.
The function then returns a new string formed by repeating the input string the specified number of times.
Here’s the basic syntax of the str_repeat() function:
php str_repeat(string $input_string, int $multiplier): string
Now that we know the function’s signature, let’s explore its various applications.
2. Creating Simple Patterns
The most straightforward use of str_repeat() is to create simple patterns within strings. This can be particularly handy for generating repeating characters like hyphens, asterisks, or underscores to separate sections of your output.
Example 1: Creating a Divider Line
Suppose you want to create a divider line in your console output. You can use str_repeat() like this:
php $divider = str_repeat('-', 30); echo $divider;
In this example, we create a line of hyphens that repeats 30 times. The output will be:
diff ------------------------------
This is a quick and efficient way to generate repetitive elements in your strings without the need for extensive loops or manual concatenation.
3. Padding Text
Another common use case for str_repeat() is text padding. You can use it to add a specific character (e.g., spaces) to the beginning or end of a string to achieve a desired length.
Example 2: Padding Text to a Fixed Length
Let’s say you have a product name that you want to display in a fixed-width format. You can use str_repeat() to pad the product name with spaces to ensure it always takes up the same amount of space:
php $productName = "Widget"; $paddedProductName = str_pad($productName, 10, ' '); echo "|$paddedProductName|"; // Enclose in vertical bars for clarity
In this example, we pad the product name with spaces to make it 10 characters wide. The output will be:
|Widget |
4. Generating Complex Patterns
While str_repeat() is great for simple patterns and padding, it can also be used creatively to generate more complex patterns within strings. Let’s explore some advanced use cases.
Example 3: Creating a Checkerboard Pattern
Imagine you want to generate a checkerboard pattern for a game board. You can use str_repeat() in combination with loops to achieve this:
php $size = 8; // Size of the checkerboard $board = ''; for ($row = 0; $row < $size; $row++) { for ($col = 0; $col < $size; $col++) { if (($row + $col) % 2 === 0) { $board .= str_repeat('?', 2); // Black square } else { $board .= str_repeat('?', 2); // White square } } $board .= "\n"; } echo $board;
In this example, we create an 8×8 checkerboard pattern using black squares (‘?’) and white squares (‘?’). The str_repeat() function is used to repeat each square character twice, and loops are used to construct the entire board. The output will resemble a classic checkerboard.
???????? ???????? ???????? ???????? ???????? ???????? ???????? ????????
This example demonstrates the power of combining str_repeat() with loops to create more intricate patterns.
5. Handling Special Characters
When working with special characters or escape sequences within your patterns, you need to be aware of how str_repeat() behaves. Since str_repeat() operates on a per-character basis, special characters are treated like individual characters, and their escape sequences should be properly escaped.
Example 4: Repeating Escape Sequences
Let’s say you want to create a string with newline characters for formatting:
php $pattern = str_repeat("\n", 3); echo "Line 1$pattern Line 2";
In this example, we’re attempting to insert three newline characters between “Line 1” and “Line 2.” However, since str_repeat() treats each character individually, this won’t produce the expected output. Instead, you’ll get three separate newline characters, resulting in three empty lines.
To achieve the desired output, you can escape the newline character like this:
php $pattern = str_repeat("\\n", 3); echo "Line 1$pattern Line 2";
Now, you’ll get the expected output with three newline characters between the lines:
mathematica Line 1 Line 2
Always be mindful of how escape sequences are handled when using str_repeat() with special characters.
6. Practical Use Cases
Now that we’ve covered the basics and some advanced usage of str_repeat(), let’s explore practical scenarios where this function can be incredibly handy.
6.1. Templating
In web development, you often need to generate HTML or XML tags with specific attributes multiple times. str_repeat() can help you create templates for repeating elements like table rows, list items, or form inputs with ease.
Example 5: Generating Table Rows
php $rowTemplate = '<tr><td>%s</td><td>%s</td></tr>'; $data = [ ['John', 'Doe'], ['Jane', 'Smith'], ['Bob', 'Johnson'], ]; $tableRows = ''; foreach ($data as $row) { $tableRows .= sprintf($rowTemplate, ...$row); } echo "<table>$tableRows</table>";
In this example, we create an HTML table by repeatedly applying the $rowTemplate for each data entry. This approach is much cleaner and more maintainable than manually concatenating HTML strings.
6.2. Text Generation
Generating repetitive text is a common task in programming. Whether it’s generating placeholder text for testing or creating random data for a script, str_repeat() can make the task more efficient.
Example 6: Generating Placeholder Text
php $placeholderText = 'Lorem ipsum dolor sit amet, consectetur adipiscing elit.'; $repeatedText = str_repeat($placeholderText, 5); echo $repeatedText;
In this example, we generate a block of Lorem Ipsum text by repeating a predefined string five times. This is useful when you need filler text for testing or design purposes.
6.3. Creating Custom Sequences
You can use str_repeat() to create custom sequences for various purposes. For instance, generating sequential invoice or order numbers with specific formats.
Example 7: Generating Invoice Numbers
php $baseInvoiceNumber = 'INV-'; for ($i = 1; $i <= 5; $i++) { $invoiceNumber = $baseInvoiceNumber . str_pad($i, 4, '0', STR_PAD_LEFT); echo $invoiceNumber . "\n"; }
In this example, we create a sequence of invoice numbers with leading zeros to make them four digits long. The str_repeat() function is used to repeat the base invoice number prefix.
Conclusion
PHP’s str_repeat() function is a versatile tool that can help you create a wide range of patterns within strings, from simple separators to complex designs. By understanding its capabilities and combining it with other PHP functions, you can streamline your string manipulation tasks and make your code more efficient and readable.
In this guide, we’ve explored the basics of str_repeat(), including how to create simple patterns, pad text, generate complex designs, handle special characters, and apply it to practical use cases. Armed with this knowledge, you can leverage str_repeat() to enhance your PHP applications and streamline your string-related tasks.
Table of Contents
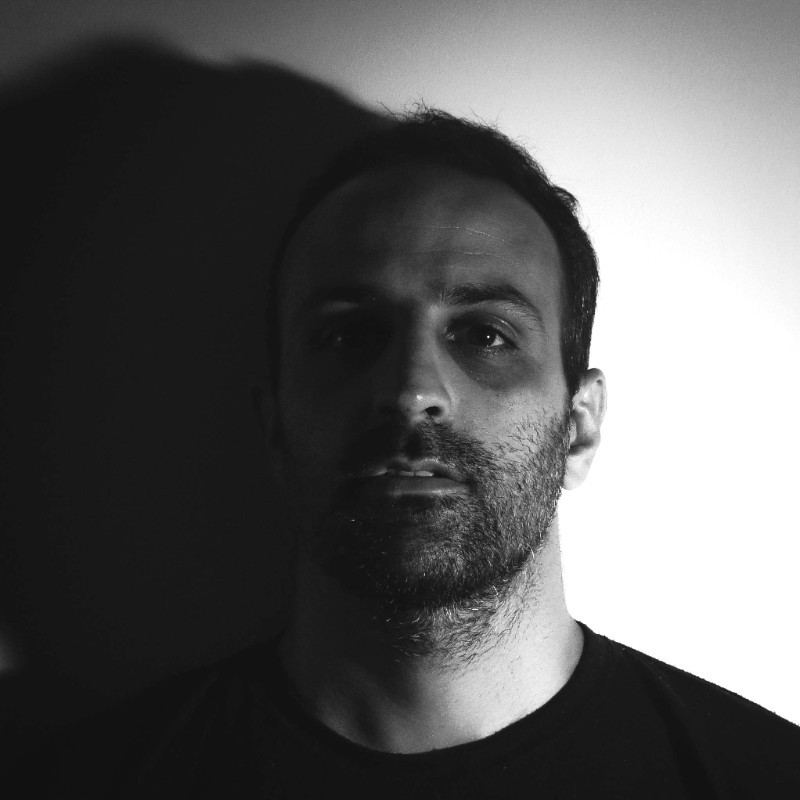
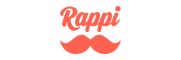