Getting Started with PHP’s str_split() Function
When it comes to working with strings in PHP, having efficient and flexible functions at your disposal is essential. One such function that can greatly simplify your string manipulation tasks is the str_split() function. This often-underutilized function allows you to split a string into an array of smaller chunks, making it easier to process, analyze, and transform your textual data. In this guide, we’ll delve into the world of str_split(), exploring its capabilities, use cases, and providing you with real-world examples to get you started on the right foot.
Table of Contents
1. Understanding the Basics of str_split()
1.1. What is the str_split() Function?
In PHP, the str_split() function is a powerful tool designed to split a given string into an array of smaller substrings. Each substring corresponds to a specified length, which can be defined by the user. This function is incredibly useful for tasks where you need to divide a string into manageable portions, such as processing text data, extracting specific information, or formatting text for output.
1.2. Syntax and Parameters
The basic syntax of the str_split() function is as follows:
php str_split(string $string, int $length = 1): array
- string: The input string that you want to split.
- length: An optional parameter that defines the length of each substring. If not provided, the default value is 1.
2. Simple Usage Examples
2.1. Splitting a String into Equal Chunks
Let’s start with a straightforward example of using str_split() to split a string into equal chunks. Suppose you have a string containing a sentence, and you want to split it into individual words for further processing.
php $originalString = "PHP is a versatile scripting language used for web development"; $wordsArray = str_split($originalString, 4); print_r($wordsArray);
In this example, the output will be an array containing:
php Array ( [0] => PHP [1] => is [2] => a v [3] => ersa [4] => tile [5] => scr [6] => ipti [7] => ng l [8] => angu [9] => age [10] => use [11] => d f [12] => or [13] => web [14] => dev [15] => elop [16] => ment )
2.2. Handling Multibyte Characters
The str_split() function is also versatile when it comes to handling multibyte characters, such as those found in various languages. Consider a scenario where you want to split a UTF-8 encoded string into individual characters:
php $utf8String = "?????"; // "Hello" in Japanese $charactersArray = str_split($utf8String, 1); print_r($charactersArray);
In this case, the output will be an array containing:
php Array ( [0] => ? [1] => ? [2] => ? [3] => ? [4] => ? )
3. Practical Use Cases
3.1. Creating Acronyms from Words
One practical application of the str_split() function is creating acronyms from words. Let’s say you have a list of terms, and you want to generate acronyms by taking the first letter from each word:
php $terms = "Hyper Text Markup Language"; $words = explode(" ", $terms); // Split the input string into words $acronym = ""; foreach ($words as $word) { $letters = str_split($word); $acronym .= $letters[0]; } echo "Acronym: $acronym";
For the input “Hyper Text Markup Language,” the output will be:
makefile Acronym: HTML
3.2. Formatting Credit Card Numbers
Another use case involves formatting credit card numbers for display. Let’s assume you have a credit card number without spaces, and you want to present it in groups of four digits each:
php $creditCardNumber = "1234567890123456"; $segments = str_split($creditCardNumber, 4); $formattedNumber = implode(" ", $segments); echo "Formatted Credit Card Number: $formattedNumber";
The output will be:
yaml Formatted Credit Card Number: 1234 5678 9012 3456
4. Tips and Best Practices
4.1. Memory Considerations
While str_split() is a convenient function, it’s important to be mindful of memory usage, especially when dealing with large strings. Splitting a string into small chunks can lead to increased memory consumption, which might become an issue in memory-constrained environments. Always consider the overall memory requirements of your application when using this function.
4.2. Error Handling
When working with the str_split() function, it’s crucial to validate the input string and handle potential errors. If you provide an empty string or a string with a negative length parameter, the function will return an empty array. Make sure to include appropriate checks to handle such cases gracefully and prevent unexpected behavior.
Conclusion
The str_split() function is a valuable tool in PHP for manipulating strings with ease. Whether you need to split a string into chunks for processing or transform text data into more structured formats, this function provides a versatile solution. By understanding its syntax, parameters, and practical applications, you can harness its capabilities to streamline your string manipulation tasks. Remember to keep memory considerations and error handling in mind, and you’ll be well on your way to becoming proficient with the str_split() function. As you explore its possibilities, you’ll find it to be an indispensable asset in your PHP programming toolkit.
Table of Contents
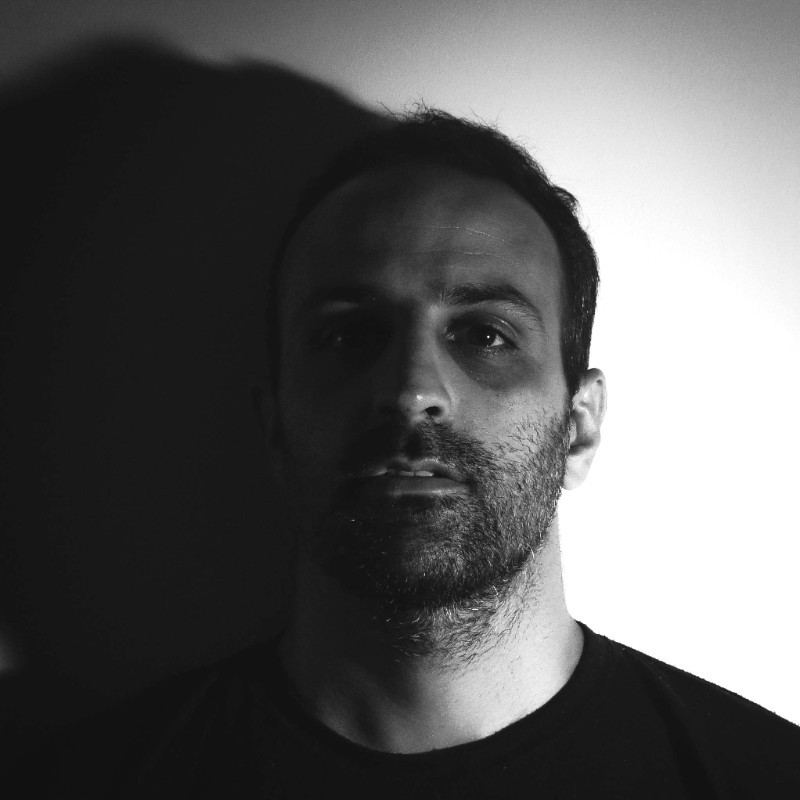
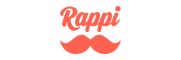