PHP’s str_word_count() Function: Counting Words in Strings
In the world of web development, PHP stands as one of the most versatile and widely used programming languages. It provides a myriad of built-in functions and libraries that simplify common tasks, making it a go-to choice for developers. Among these functions, str_word_count() holds a special place when it comes to parsing and analyzing text. In this blog post, we’ll dive deep into PHP’s str_word_count() function, exploring its features, usage, and practical examples.
Table of Contents
1. Understanding the Basics
Before delving into the specifics of str_word_count(), let’s establish a clear understanding of what it does. In essence, this function is designed to count the number of words within a given string. A word, in this context, is defined as a sequence of characters separated by spaces or punctuation marks. For instance, in the string “Hello, World!”, the function would identify two words: “Hello” and “World.”
1.1. Syntax of str_word_count()
The syntax of str_word_count() is relatively straightforward:
php str_word_count(string $string, int $format = 0, string|null $charlist = null)
Here’s a breakdown of the parameters:
- $string: The input string that you want to analyze.
- $format (optional): An integer that specifies the return format. It can have one of the following values:
- 0 (default): Returns the total word count as an integer.
- 1: Returns an array containing all the words found in the input string.
- 2: Returns an associative array where the key is the word and the value is its position in the string.
- $charlist (optional): A list of additional characters to consider as word characters. By default, it includes letters, numbers, and the underscore (_).
Now, let’s explore these parameters and their functionality through practical examples.
2. Counting Words in a String
Example 1: Basic Word Count
Let’s start with a simple example. Suppose we have the following string:
php $text = "The quick brown fox jumps over the lazy dog";
We want to count the number of words in this string using the default format (0):
php $wordCount = str_word_count($text); echo "Word count: $wordCount";
The output of this code will be:
mathematica Word count: 9
As expected, there are nine words in the given string.
Example 2: Returning an Array of Words
In some cases, you might need to retrieve all the words as an array. You can achieve this by setting the $format parameter to 1:
php $wordsArray = str_word_count($text, 1); print_r($wordsArray);
The output will be an array containing all the words:
php Array ( [0] => The [1] => quick [2] => brown [3] => fox [4] => jumps [5] => over [6] => the [7] => lazy [8] => dog )
This format can be useful if you need to process individual words separately.
Example 3: Getting Word Positions
By setting the $format parameter to 2, you can obtain an associative array that maps each word to its position in the original string:
php $wordsWithPositions = str_word_count($text, 2); print_r($wordsWithPositions);
The output will look like this:
php Array ( [0] => The [4] => quick [10] => brown [16] => fox [20] => jumps [26] => over [31] => the [35] => lazy [40] => dog )
Here, the keys represent the starting positions of each word in the input string.
3. Customizing Word Recognition
The $charlist parameter allows you to customize which characters are recognized as word characters. This can be particularly useful when dealing with strings containing non-standard characters or symbols. Let’s see how it works:
Example 4: Custom Character List
Suppose we have the following string with underscores and hyphens:
php $text = "This_is-a_sample_string";
If we want to count words while considering underscores and hyphens as word characters, we can specify them in the $charlist parameter:
php $wordCountWithCustomChars = str_word_count($text, 0, '_-'); echo "Word count with custom characters: $wordCountWithCustomChars";
The output will be:
vbnet Word count with custom characters: 5
In this case, the function recognizes “This,” “is,” “a,” “sample,” and “string” as words, even though they contain underscores and hyphens.
4. Practical Use Cases
Now that we’ve covered the basics and explored various examples, let’s take a look at some practical use cases where str_word_count() can be beneficial.
4.1. Word Counting in Text Analytics
Text analytics and natural language processing often involve analyzing text data. Counting words is a fundamental step in understanding the content and extracting insights from textual information. str_word_count() can simplify the process of word frequency analysis, sentiment analysis, and more.
4.2. Validating User Input
When building web applications, it’s common to validate user input. You can use str_word_count() to check if a user’s input falls within an acceptable word limit. For instance, you might restrict the length of a comment or a post on a social media platform.
php $maxWordCount = 100; $userInput = $_POST['user_comment']; if (str_word_count($userInput) > $maxWordCount) { echo "Your comment exceeds the maximum word limit."; } else { // Process the comment }
4.3. Generating SEO Metadata
In content management systems and blogging platforms, generating SEO metadata is crucial for improving a website’s visibility on search engines. You can use str_word_count() to automatically generate meta descriptions or extract keywords from blog posts to enhance SEO.
php $blogPostContent = "Lorem ipsum dolor sit amet, consectetur adipiscing elit..."; $metaDescription = substr($blogPostContent, 0, 150); // Extract the first 150 characters for meta description
5. Common Pitfalls and Considerations
While str_word_count() is a valuable tool, there are some considerations and common pitfalls to be aware of:
5.1. Multibyte Character Support
If your application deals with multibyte character sets, such as UTF-8, you should be cautious when using str_word_count(). By default, it operates on byte-level, which may not accurately count words in languages with complex characters. To handle multibyte characters correctly, consider using the mb_str_word_count() function provided by the Multibyte String extension in PHP.
5.2. Punctuation and Symbols
str_word_count() considers some punctuation marks as word separators. If you have specific requirements for how punctuation should be treated, you may need to preprocess the string or use regular expressions to achieve the desired behavior.
5.3. Word Definitions
The definition of a “word” can vary depending on context. str_word_count() uses a basic algorithm to split words, which may not align with more advanced linguistic definitions. If your application requires precise word boundaries, you might need to implement a custom word tokenizer.
Conclusion
PHP’s str_word_count() function is a valuable asset for any developer working with text data. It simplifies the process of counting words in strings and offers flexibility in how you retrieve and manipulate the word data. Whether you’re analyzing text, validating user input, or enhancing SEO, this function can streamline your tasks and improve the efficiency of your PHP applications.
As you continue to explore PHP and its rich ecosystem of functions, str_word_count() will undoubtedly prove to be a handy tool in your toolkit. So, go ahead, experiment with different formats and options, and leverage this function to enhance your web development projects. Happy coding!
Table of Contents
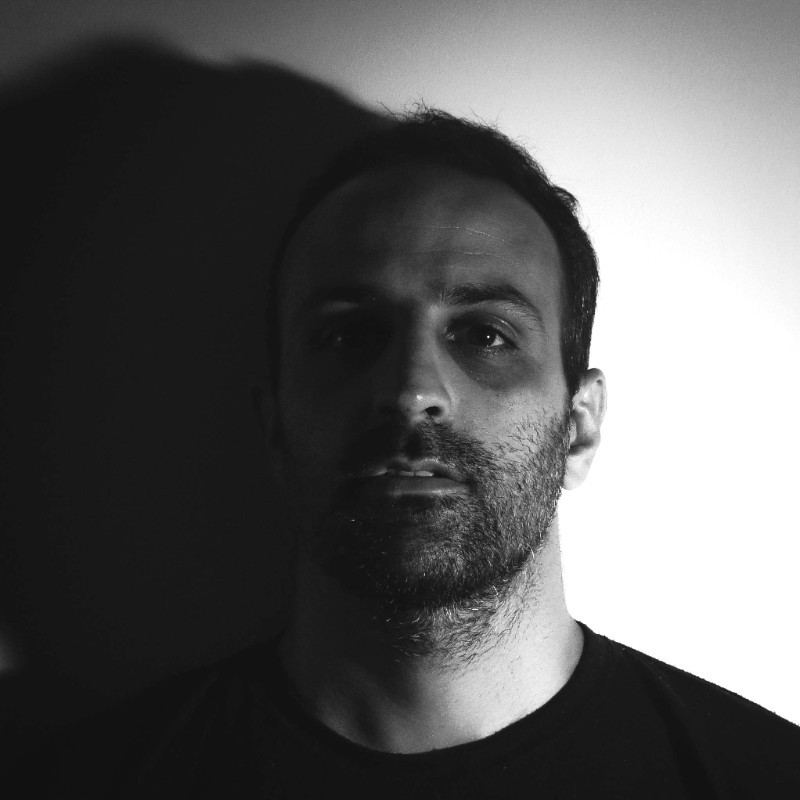
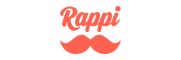