Understanding the PHP’s strcasecmp() Function
When working with strings in PHP, there are often situations where you need to compare two strings to determine if they are equal, regardless of their case. PHP provides several string comparison functions to help you achieve this, and one of the most useful among them is strcasecmp(). In this blog, we will delve into the strcasecmp() function, exploring its syntax, usage, and providing practical examples to illustrate its power and versatility.
Table of Contents
1. What is strcasecmp()?
strcasecmp() is a PHP function designed for case-insensitive string comparisons. It’s incredibly handy when you want to compare two strings while disregarding their letter case (uppercase or lowercase). This function returns 0 if the two strings are equal in a case-insensitive manner, a negative integer if the first string is less than the second, and a positive integer if the first string is greater than the second.
2. Syntax of strcasecmp()
Before we dive into examples, let’s understand the syntax of the strcasecmp() function:
php strcasecmp(string $str1, string $str2): int
- $str1: The first string to compare.
- $str2: The second string to compare.
The function takes two strings as arguments and returns an integer value, indicating the result of the comparison.
3. Using strcasecmp() for Case-Insensitive Comparisons
To understand how strcasecmp() works, let’s look at a few practical examples.
Example 1: Basic Usage
php $string1 = "Hello"; $string2 = "hello"; $result = strcasecmp($string1, $string2); if ($result === 0) { echo "The strings are equal."; } else { echo "The strings are not equal."; }
In this example, $string1 and $string2 contain “Hello” and “hello,” respectively. When we use strcasecmp() to compare these strings, it returns 0, indicating that the strings are equal in a case-insensitive manner. Therefore, the output will be “The strings are equal.”
Example 2: Case-Insensitive Sorting
strcasecmp() is also valuable when sorting an array of strings in a case-insensitive manner. Consider the following example:
php $fruits = ["apple", "Banana", "Cherry", "banana", "cherry", "Apple"]; usort($fruits, function($a, $b) { return strcasecmp($a, $b); }); print_r($fruits);
In this example, we have an array of fruits with mixed cases. We use usort() along with strcasecmp() as the comparison function to sort the array in a case-insensitive way. The result will be [“apple”, “Apple”, “Banana”, “banana”, “Cherry”, “cherry”], demonstrating that the sorting ignores the case of the letters.
4. Handling Special Characters
strcasecmp() is not limited to English characters. It can also handle special characters and accented letters, making it suitable for internationalization purposes.
Example 3: Case-Insensitive Comparison with Special Characters
php $string1 = "Café"; $string2 = "CAFE"; $result = strcasecmp($string1, $string2); if ($result === 0) { echo "The strings are equal."; } else { echo "The strings are not equal."; }
In this example, $string1 contains “Café” with an accented ‘e,’ while $string2 contains “CAFE” in uppercase. Despite the accent and case difference, strcasecmp() treats them as equal and outputs “The strings are equal.”
5. Common Pitfalls and Tips
While strcasecmp() is a powerful tool for case-insensitive string comparisons, it’s essential to be aware of some common pitfalls and tips:
Pitfall 1: Use Identical Comparison (===) for Strict Equality
In the examples we’ve seen, we use === to check if the result of strcasecmp() is 0 to determine equality. This is because strcasecmp() returns 0 when strings are equal in a case-insensitive manner. However, if you need to check for strict equality, including the same case, use === directly on the strings.
Pitfall 2: Be Mindful of Locale
strcasecmp() uses a binary comparison, which means it doesn’t consider locale-specific rules for case-insensitivity. If your application needs to handle locale-specific comparisons, consider using strcoll() instead.
Tip 1: Keep String Comparison in Mind
Remember that strcasecmp() only compares two strings. If you’re dealing with more complex comparisons or pattern matching, you might need to explore regular expressions or other string manipulation functions.
Tip 2: Consider Case-Folding
For Unicode strings and internationalization, it’s often recommended to use case-folding functions like mb_strtolower() or mb_strtoupper() in conjunction with strcasecmp() for more accurate case-insensitive comparisons.
Conclusion
The strcasecmp() function in PHP is a valuable tool for performing case-insensitive string comparisons. Its simplicity and ease of use make it a preferred choice when you need to compare strings without worrying about their case. Whether you’re validating user input, sorting data, or handling internationalization, strcasecmp() is a reliable function to have in your PHP toolkit. By understanding its syntax and common use cases, you can make your PHP applications more robust and user-friendly, especially when dealing with diverse sets of data and languages.
Table of Contents
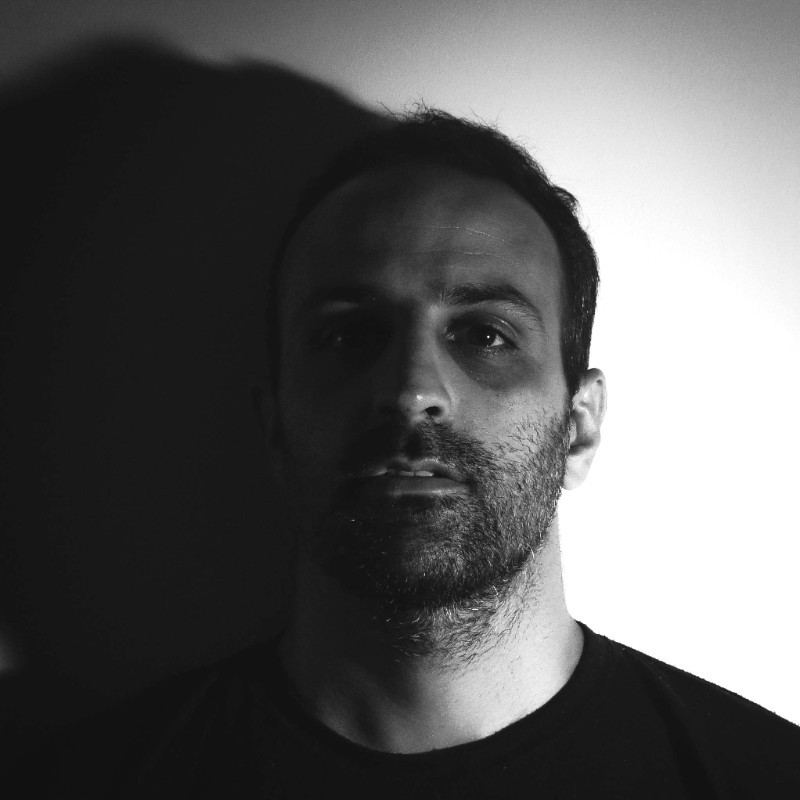
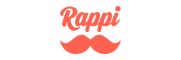