Mastering PHP’s strcmp() Function: A Detailed Guide
PHP is a versatile programming language widely used for web development. It provides a plethora of functions to handle strings, and one of the most important ones is strcmp(). This function is used for comparing two strings, but understanding its nuances and mastering its usage can greatly improve your PHP coding skills. In this detailed guide, we will delve into the world of strcmp(), exploring its purpose, usage, examples, and best practices to help you become a proficient PHP developer.
Table of Contents
1. Understanding strcmp() Basics
Before diving into the advanced usage of strcmp(), let’s start with the basics. The strcmp() function is primarily used for comparing two strings and determining their relationship in terms of lexicographical order. It takes two strings as input and returns an integer value, which signifies the result of the comparison.
1.1. Syntax
php strcmp(string1, string2)
- string1: The first string for comparison.
- string2: The second string for comparison.
1.2. Return Value
- 0: If both strings are equal.
- A positive integer: If string1 is greater than string2.
- A negative integer: If string1 is less than string2.
Let’s explore these return values with some examples.
Example 1: Equal Strings
php $result = strcmp("apple", "apple"); echo $result; // Outputs 0
In this case, both strings are equal, so strcmp() returns 0.
Example 2: First String Greater
php $result = strcmp("banana", "apple"); echo $result; // Outputs a positive integer
Here, “banana” is lexicographically greater than “apple,” so strcmp() returns a positive integer.
Example 3: Second String Greater
php $result = strcmp("apple", "banana"); echo $result; // Outputs a negative integer
Conversely, when “apple” is compared to “banana,” strcmp() returns a negative integer.
Understanding these basic principles is crucial for utilizing strcmp() effectively in your PHP applications. However, there are some subtleties to consider when working with this function in more complex scenarios.
2. Case Sensitivity in strcmp()
By default, strcmp() is case-sensitive, meaning it considers uppercase and lowercase characters as distinct. For many scenarios, this is the desired behavior, but there may be situations where you want to perform a case-insensitive comparison. To achieve this, you can use the strcasecmp() function.
2.1. Syntax
php strcasecmp(string1, string2)
strcasecmp() works similarly to strcmp(), but it performs a case-insensitive comparison. It returns the same integer values as strcmp(), but the comparison is not affected by the letter case.
Let’s see an example:
php $result = strcasecmp("apple", "Apple"); echo $result; // Outputs 0
In this case, strcasecmp() returns 0 because it ignores the case difference between “apple” and “Apple.”
3. Best Practices and Common Use Cases
Now that you have a good grasp of the fundamentals, let’s explore some best practices and common use cases for strcmp().
3.1. Error-Handling with strcmp()
When using strcmp(), it’s essential to consider error handling. If one or both of the input strings are null, it will result in a warning and return null. To avoid this, you can explicitly check for null values before calling strcmp().
php $string1 = "apple"; $string2 = null; if ($string1 !== null && $string2 !== null) { $result = strcmp($string1, $string2); echo $result; } else { echo "One or both strings are null."; }
By performing this check, you ensure that strcmp() is only called when both strings are valid, preventing unexpected warnings or errors.
3.2. Sorting Arrays with strcmp()
strcmp() is often used for sorting arrays of strings. You can use it as a callback function with sorting functions like usort() to define custom sorting criteria. Here’s an example of how to sort an array of names in ascending order:
php $names = ["John", "Alice", "Bob", "Zoe", "Eve"]; usort($names, function($a, $b) { return strcmp($a, $b); }); print_r($names);
This code sorts the $names array alphabetically in ascending order.
3.3. Handling User Input
When working with user input, it’s crucial to validate and sanitize data. strcmp() can be useful for comparing user input to predefined values or patterns. For instance, you can check if a user’s choice matches specific options:
php $userChoice = $_POST['choice']; $options = ["Option A", "Option B", "Option C"]; foreach ($options as $option) { if (strcmp($userChoice, $option) === 0) { echo "User selected: " . $option; break; } }
This code checks if the user’s choice matches any of the predefined options and provides feedback accordingly.
3.4. String Comparison in Multilingual Applications
When working on multilingual applications, you may encounter scenarios where you need to compare strings with different character sets and languages. In such cases, you should be aware that strcmp() uses binary comparison, which may not always yield the desired results.
For multilingual applications, consider using the collator class or the strcoll() function, which provide more accurate and locale-aware string comparisons.
Conclusion
Mastering PHP’s strcmp() function is a valuable skill for any PHP developer. It allows you to compare strings effectively, handle user input, and perform custom sorting in arrays. Understanding its nuances, including case sensitivity and error handling, is essential for writing robust PHP applications.
In this detailed guide, we’ve covered the basics of strcmp(), its case-insensitive counterpart strcasecmp(), and explored best practices and common use cases. Armed with this knowledge, you’ll be better equipped to utilize strcmp() in your PHP projects and write efficient, error-free code.
As you continue your PHP journey, remember that strcmp() is just one of many string manipulation tools at your disposal. By mastering it and exploring other PHP functions, you’ll become a more proficient and versatile developer capable of tackling a wide range of web development tasks. Happy coding!
Table of Contents
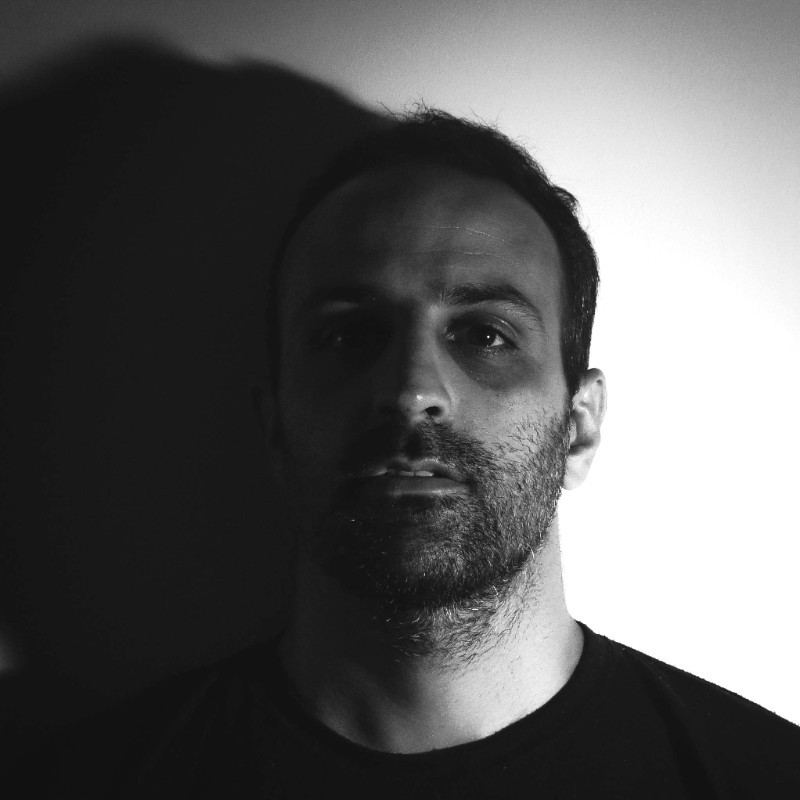
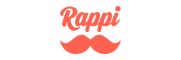