Understanding PHP String Functions: A Comprehensive Guide
PHP is a powerful programming language widely used for web development. One of its fundamental features is the handling of strings. Strings are essential for storing and processing text data, making them a crucial part of any web application. PHP offers a rich set of built-in string functions that enable developers to perform various operations on strings, such as manipulating, searching, and transforming them.
In this comprehensive guide, we will dive into the world of PHP string functions, exploring their functionalities with detailed explanations and code samples. Whether you are a beginner or an experienced developer, this guide will help you understand the various PHP string functions and how to leverage them effectively in your projects.
1. Getting Started with Strings in PHP:
Strings in PHP are sequences of characters enclosed within single quotes or double quotes. Double-quoted strings allow variable interpolation, which means variables within the string will be evaluated and replaced with their values. Let’s see some examples:
php $singleQuoted = 'This is a single-quoted string.'; $doubleQuoted = "This is a double-quoted string."; $variable = 'PHP'; $interpolated = "I love $variable"; // Output: I love PHP
1.1. What are Strings?
A string is a sequence of characters, such as letters, numbers, and symbols. In PHP, strings can be represented using single quotes or double quotes. Single-quoted strings are treated as literal strings, while double-quoted strings allow variable interpolation and escape sequences.
1.2. Declaring Strings:
To declare a string, you can use either single quotes or double quotes:
php $singleQuoted = 'This is a single-quoted string.'; $doubleQuoted = "This is a double-quoted string.";
1.3. Concatenation:
String concatenation is the process of combining two or more strings into a single string. In PHP, concatenation is done using the dot (.) operator:
php $greeting = 'Hello, '; $name = 'John'; $message = $greeting . $name; // Output: Hello, John
1.4. String Length:
You can find the length of a string using the strlen() function:
php $str = 'Hello'; $length = strlen($str); // Output: 5
2. Manipulating Strings:
PHP provides various functions to manipulate strings, such as extracting substrings, replacing text, and removing whitespace.
2.1. Substrings:
To extract a substring from a string, you can use the substr() function:
php $str = 'Hello, World!'; $substring = substr($str, 0, 5); // Output: Hello
The substr() function takes three arguments: the original string, the starting index, and the length of the substring.
2.2. Extracting Parts of a String:
The strstr() function can be used to extract a part of a string from the first occurrence of a specific substring:
php $str = 'Hello, World!'; $substring = strstr($str, 'World'); // Output: World!
To get the substring before the needle, you can set the third argument to true:
php $str = 'Hello, World!'; $substring = strstr($str, 'World', true); // Output: Hello,
2.3. Replacing Text in a String:
To replace occurrences of a substring within a string, you can use the str_replace() function:
php $str = 'Hello, World!'; $newStr = str_replace('World', 'John', $str); // Output: Hello, John!
2.4. Removing Whitespace:
To remove whitespace from the beginning and end of a string, you can use the trim() function:
php $str = ' Hello, World! '; $trimmedStr = trim($str); // Output: Hello, World!
If you only want to remove whitespace from the left or right side, you can use ltrim() and rtrim() respectively.
3. Searching within Strings:
PHP provides several functions for searching within strings, allowing you to find substrings or characters with ease.
3.1. Finding Substrings:
The strpos() function helps you find the position of the first occurrence of a substring within a string:
php $str = 'Hello, World!'; $pos = strpos($str, 'World'); // Output: 7
If the substring is not found, strpos() will return false.
3.2. Searching from the End:
To find the last occurrence of a substring within a string, you can use strrpos():
php $str = 'Hello, World!'; $pos = strrpos($str, 'o'); // Output: 8
3.3. Case-Insensitive Search:
If you need a case-insensitive search, you can use stripos() or strripos():
php $str = 'Hello, World!'; $pos = stripos($str, 'world'); // Output: 7
3.4. Regular Expression Search:
For more complex search patterns, you can use preg_match() to perform regular expression searches:
php $str = 'Hello, World!'; if (preg_match('/\bW\w+/', $str, $matches)) { echo $matches[0]; // Output: World }
4. Modifying Case:
PHP offers functions to change the case of strings, making it easier to work with different text formats.
4.1. Converting to Lowercase:
The strtolower() function converts a string to lowercase:
php $str = 'Hello, World!'; $lowercase = strtolower($str); // Output: hello, world!
4.2. Converting to Uppercase:
To convert a string to uppercase, you can use strtoupper():
php $str = 'Hello, World!'; $uppercase = strtoupper($str); // Output: HELLO, WORLD!
4.3. Capitalizing Strings:
The ucfirst() function capitalizes the first letter of a string:
php $str = 'hello, world!'; $capitalized = ucfirst($str); // Output: Hello, world!
To capitalize the first letter of each word in a string, you can use ucwords():
php $str = 'hello, world!'; $capitalizedWords = ucwords($str); // Output: Hello, World!
5. String Comparison:
When working with strings, you often need to compare them. PHP provides functions for different types of string comparisons.
5.1. Comparing Two Strings:
To compare two strings, you can use strcmp() or strcasecmp():
php $str1 = 'apple'; $str2 = 'banana'; if (strcmp($str1, $str2) === 0) { echo 'Strings are equal'; } else { echo 'Strings are not equal'; // Output: Strings are not equal }
5.2. Case-Insensitive Comparison:
If you need a case-insensitive comparison, use strcasecmp():
php $str1 = 'Hello'; $str2 = 'hello'; if (strcasecmp($str1, $str2) === 0) { echo 'Strings are equal'; // Output: Strings are equal } else { echo 'Strings are not equal'; }
5.3. Binary Comparison:
For binary-safe comparisons, you can use strncmp() or strncasecmp():
php $str1 = 'apple'; $str2 = 'appetizer'; if (strncmp($str1, $str2, 4) === 0) { echo 'First 4 characters are equal'; // Output: First 4 characters are equal } else { echo 'First 4 characters are not equal'; }
6. Formatting Strings:
Formatting strings is crucial for displaying data correctly. PHP provides various functions to format strings as needed.
6.1. Padding Strings:
You can pad a string with characters to a certain length using str_pad():
php $str = 'Hello'; $paddedStr = str_pad($str, 10, '-'); // Output: Hello-----
6.2. Trimming Strings:
We’ve already seen how to remove whitespace from the beginning and end of a string with trim(). However, rtrim() and ltrim() are useful for removing specific characters from the right or left side:
php $str = 'Hello, World!'; $trimmedStr = rtrim($str, '!'); // Output: Hello, World
6.3. Formatting Numbers in Strings:
When dealing with numbers in strings, number_format() is helpful for formatting them with commas as thousands separators:
php $num = 1234567.89; $formattedNum = number_format($num); // Output: 1,234,567.89
6.4. Localized String Formatting:
For localized formatting, you can use the setlocale() and numfmt_format() functions:
php $locale = 'en_US'; setlocale(LC_ALL, $locale); $number = 1234567.89; $formatter = numfmt_create($locale, NumberFormatter::DECIMAL); $localized = numfmt_format($formatter, $number); // Output: 1,234,567.89
7. Parsing and Splitting Strings:
PHP provides functions to parse strings into arrays or extract specific portions using delimiters.
7.1. Breaking a String into an Array:
You can use explode() to split a string into an array based on a delimiter:
php $str = 'apple,banana,orange'; $fruits = explode(',', $str); // Output: ['apple', 'banana', 'orange']
7.2. Extracting Substrings using Delimiters:
The strstr() function can also be used to extract substrings based on a delimiter:
php $str = 'https://www.example.com'; $domain = strstr($str, '://'); // Output: //www.example.com
To get the substring after the delimiter, set the third argument to true.
7.3. Parsing Query Strings:
PHP has a convenient function, parse_str(), to parse query strings into associative arrays:
php $queryString = 'name=John&age=30&gender=male'; parse_str($queryString, $params); print_r($params); /* Output: Array ( [name] => John [age] => 30 [gender] => male ) */
8. Encoding and Escaping:
When working with URLs, HTML, or databases, it’s crucial to encode and escape strings properly to avoid security vulnerabilities.
8.1. URL Encoding and Decoding:
To encode and decode URLs, you can use urlencode() and urldecode():
php $str = 'Hello, World!'; $encodedStr = urlencode($str); // Output: Hello%2C+World%21 $decodedStr = urldecode($encodedStr); // Output: Hello, World!
8.2. HTML Entities and Special Characters:
PHP provides htmlspecialchars() to escape special characters for HTML output:
php $str = '<script>alert("XSS!");</script>'; $escapedStr = htmlspecialchars($str); // Output: <script>alert("XSS!");</script>
8.3. Escaping Strings for Databases:
When inserting data into a database, you must escape strings to prevent SQL injection attacks. The commonly used function is mysqli_real_escape_string():
php $unsafeStr = "John's data"; $escapedStr = mysqli_real_escape_string($connection, $unsafeStr);
Conclusion:
In this comprehensive guide, we’ve explored the world of PHP string functions, covering everything from basic manipulations to advanced searching and formatting. Understanding these functions is crucial for any PHP developer, as strings are an integral part of web development.
By utilizing the various PHP string functions discussed here, you can efficiently manipulate, search, and transform strings, enhancing the functionality and security of your web applications. Experiment with these functions, and they will undoubtedly become valuable tools in your PHP development arsenal. Happy coding!
Table of Contents
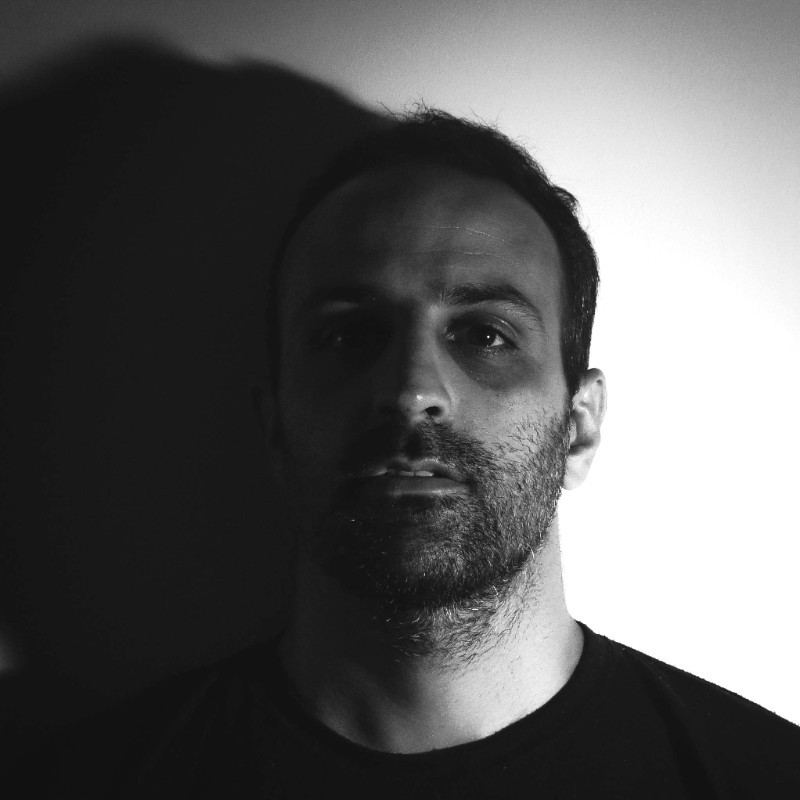
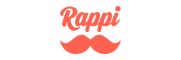