PHP Q & A
How to work with strings?
Working with strings in PHP is a fundamental aspect of web development, as strings are used to handle text and manipulate data. Here’s a comprehensive guide on how to work with strings in PHP:
Creating and Assigning Strings:
- String Declaration: In PHP, you can create strings by enclosing text within either single quotes (`’`) or double quotes (`”`). For example:
```php $singleQuoted = 'This is a single-quoted string.'; $doubleQuoted = "This is a double-quoted string."; ```
- Concatenation: To combine or concatenate strings, you can use the `.` operator. For example:
```php $firstName = "John"; $lastName = "Doe"; $fullName = $firstName . " " . $lastName; // Concatenating first and last names ```
String Manipulation:
- String Length: To find the length of a string, you can use the `strlen()` function:
```php $text = "Hello, World!"; $length = strlen($text); // $length now holds 13 ```
- Substring: You can extract a portion of a string using the `substr()` function:
```php $text = "Hello, World!"; $substring = substr($text, 0, 5); // $substring holds "Hello" ```
- String Replacement: To replace a part of a string, you can use `str_replace()`:
```php $text = "Hello, World!"; $newText = str_replace("World", "PHP", $text); // $newText holds "Hello, PHP!" ```
- String Conversion: You can convert strings to uppercase and lowercase using `strtoupper()` and `strtolower()`:
```php $text = "Hello, World!"; $uppercase = strtoupper($text); // $uppercase holds "HELLO, WORLD!" $lowercase = strtolower($text); // $lowercase holds "hello, world!" ```
String Interpolation:
- Variable Interpolation: In double-quoted strings, you can directly interpolate variables by enclosing them in curly braces `{}`:
```php $name = "Alice"; echo "Hello, {$name}!"; ```
- Escape Sequences: You can include special characters in strings using escape sequences. For example:
```php $escapedText = "This is a \"quoted\" string."; // Using double quotes inside a double-quoted string ```
Working with strings is crucial for handling user input, building dynamic web content, and processing data in PHP applications. Understanding these string manipulation techniques will help you create more interactive and functional web pages and applications.
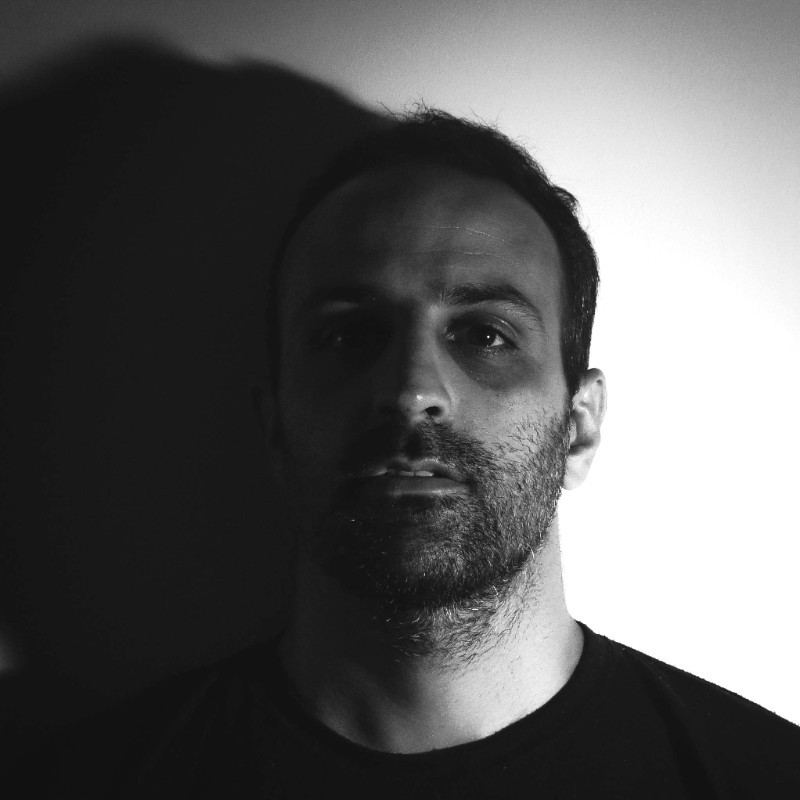
Previously at
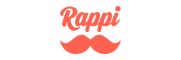
Full Stack Engineer with extensive experience in PHP development. Over 11 years of experience working with PHP, creating innovative solutions for various web applications and platforms.