Unlocking the Power of PHP’s strtolower() Function
In the realm of web development, text manipulation plays a significant role. Whether you’re dealing with user input, managing database records, or generating dynamic content, the need to handle text effectively arises frequently. PHP, a popular server-side scripting language, offers a plethora of functions to streamline text operations. Among them, strtolower() is a powerful tool that can simplify your coding tasks and enhance user experience. In this blog, we will explore the potential of strtolower() and how it can be a game-changer in your PHP projects.
Table of Contents
1. What is strtolower()?
To begin, let’s understand what strtolower() is all about. In PHP, strtolower() is a built-in function that stands for “string to lowercase.” It does precisely what its name suggests – it converts all alphabetic characters in a given string to lowercase. This simple yet versatile function can be a valuable asset in various scenarios.
1.1. Syntax of strtolower()
The syntax of strtolower() is straightforward:
php strtolower(string $string)
Here, $string represents the input string you want to convert to lowercase. It’s important to note that strtolower() doesn’t modify the original string; instead, it returns a new string with all alphabetic characters converted to lowercase.
2. Why Use strtolower()?
Now that we know what strtolower() does, let’s delve into the reasons why you should consider using it in your PHP projects.
2.1. Uniform Data Handling
In web applications, it’s common to receive user-generated content, such as usernames, email addresses, or search queries. Users may enter text in various cases (e.g., uppercase, lowercase, or mixed case). To ensure consistency and avoid potential issues, it’s essential to convert this input to a uniform format.
Consider the scenario where you want to validate email addresses. By applying strtolower() to the user’s input, you can ensure that the email domain (e.g., “@example.com”) is consistently in lowercase, regardless of how the user typed it. This simplifies validation and reduces the chances of overlooking valid email addresses due to case discrepancies.
2.2. Improved Search Functionality
Search functionality is a crucial component of many web applications. When users perform searches, they may not always type their queries in the same case as the stored data. Using strtolower() can help you overcome this challenge.
For instance, suppose you have a database of product names, and you want to implement a search feature. By converting both the user’s search query and the product names to lowercase, you can perform case-insensitive searches effortlessly. This ensures that users find what they’re looking for, even if their search queries don’t exactly match the stored data’s case.
2.3. Case-Insensitive Comparisons
In some cases, you may need to compare two strings for equality without considering their case. strtolower() simplifies this process by allowing you to convert both strings to lowercase before comparison.
Here’s an example:
php $userInput = "SecretPassword"; $storedPassword = "secretpassword"; if (strtolower($userInput) === strtolower($storedPassword)) { echo "Access granted!"; } else { echo "Access denied!"; }
In this code snippet, both the user’s input and the stored password are converted to lowercase for comparison. This ensures that the comparison is case-insensitive, making it easier to authenticate users regardless of how they enter their passwords.
2.4. Localization and Internationalization
When developing websites for a global audience, you may encounter various character sets and languages. Some languages have specific rules for case conversion, and blindly converting characters to lowercase may not yield the desired results.
However, PHP’s strtolower() function is aware of these rules and can handle them correctly. It ensures that characters with special casing rules are converted appropriately, making it a reliable choice for internationalization and localization tasks.
3. Practical Examples
Let’s explore some practical examples to illustrate how strtolower() can be used effectively in real-world PHP scenarios.
Example 1: User Registration
Consider a user registration form where users provide their email addresses. To ensure uniformity in the database, you can use strtolower() to convert the email domain to lowercase before storing it:
php $userEmail = $_POST['email']; // User input: "User@Example.com" $cleanedEmail = strtolower($userEmail); // Result: "user@example.com" // Store $cleanedEmail in the database
By doing this, you ensure that all email domains are stored in lowercase, regardless of how users enter them during registration.
Example 2: Case-Insensitive Username Check
In a user authentication system, you might want to check if a username already exists while allowing for case-insensitive comparisons. Here’s how you can use strtolower() for this purpose:
php $enteredUsername = "JohnDoe"; $existingUsernames = ["johndoe", "alice", "bob"]; $lowercaseUsername = strtolower($enteredUsername); if (in_array($lowercaseUsername, $existingUsernames)) { echo "Username already exists!"; } else { echo "Username is available!"; }
By converting both the entered username and the existing usernames to lowercase, you ensure that the check is case-insensitive.
Example 3: Search Functionality
Implementing a case-insensitive search feature in a product catalog can significantly improve the user experience. Here’s how you can achieve this using strtolower():
php $userSearchQuery = "Smartphone"; $productNames = ["iPhone 13", "Samsung Galaxy S21", "Google Pixel 6"]; $lowercaseSearchQuery = strtolower($userSearchQuery); foreach ($productNames as $productName) { $lowercaseProductName = strtolower($productName); if (strpos($lowercaseProductName, $lowercaseSearchQuery) !== false) { echo "Found product: $productName"; } }
In this example, both the user’s search query and the product names are converted to lowercase for case-insensitive matching.
4. Best Practices
While strtolower() is a valuable function, it’s essential to use it judiciously and follow best practices to ensure your code remains efficient and maintainable.
4.1. Use strtolower() for Case-Insensitive Comparisons Only
Avoid using strtolower() indiscriminately. It should primarily be used when you need to perform case-insensitive comparisons or when you want to ensure uniformity in stored data. Using it unnecessarily can lead to unexpected results and additional processing overhead.
4.2. Consider Multibyte Character Sets
If your application deals with multibyte character sets, be aware that strtolower() may not handle them correctly. In such cases, consider using functions like mb_strtolower() that are designed to work with multibyte characters.
4.3. Document Your Use of strtolower()
As with any function or code segment, it’s a good practice to document your use of strtolower() to make your code more understandable for future developers (including yourself). Clearly explain why you’re using it and what problem it solves.
4.4. Test Extensively
Before deploying your code to a production environment, thoroughly test its behavior with different inputs, including edge cases. This will help you identify and address any unexpected issues related to case sensitivity.
Conclusion
PHP’s strtolower() function is a valuable tool for simplifying text manipulation and improving user experience in web development. By using it judiciously, you can ensure uniform data handling, implement case-insensitive comparisons, and enhance the functionality of your PHP applications.
Whether you’re working on user registration, search functionality, or any other text-related task, strtolower() can be your ally in creating more robust and user-friendly web applications. So, unlock the power of strtolower() in your PHP projects and take your web development skills to the next level.
Start leveraging the simplicity and versatility of strtolower() today, and watch your PHP applications become more user-friendly and efficient than ever before. Happy coding!
Table of Contents
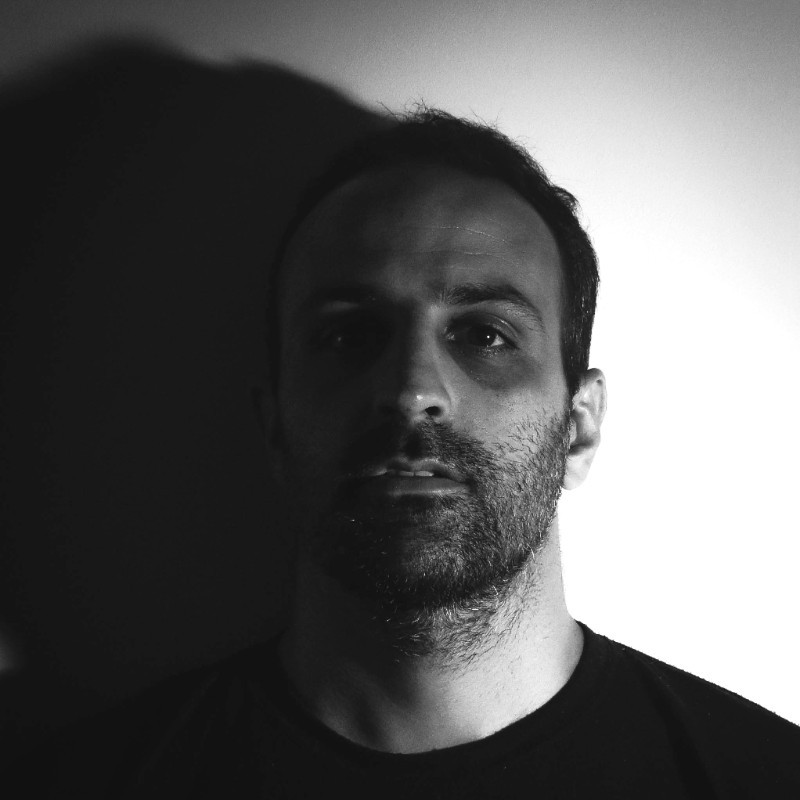
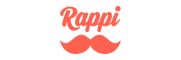