Exploring PHP’s strtotime() Function: A Comprehensive Guide
PHP is a versatile scripting language used for web development, and it offers a wide range of functions to manipulate dates and times. One such function that deserves a closer look is strtotime(). Whether you’re a seasoned developer or just starting with PHP, understanding how to use strtotime() can significantly simplify date and time handling in your applications. In this comprehensive guide, we’ll dive deep into strtotime(), exploring its syntax, examples, and practical applications.
Table of Contents
1. Understanding the Basics
Before we dive into the nitty-gritty details, let’s establish a fundamental understanding of what strtotime() does. At its core, strtotime() is a function that parses a date/time string and converts it into a Unix timestamp. A Unix timestamp represents the number of seconds that have elapsed since January 1, 1970, at 00:00:00 UTC (Coordinated Universal Time).
1.1. Syntax
The basic syntax of strtotime() is as follows:
php strtotime(string $time, int $now = time())
- $time: This is the input string containing the date and time information you want to convert. It can be in various formats, as we will explore later.
- $now: An optional parameter representing the current timestamp. If not provided, it defaults to the current time when the function is called.
2. Date and Time Formats
One of the strengths of strtotime() is its ability to interpret a wide range of date and time formats. This flexibility makes it incredibly useful in real-world scenarios where you might encounter dates and times in various formats.
2.1. Relative Formats
Let’s start with relative formats. These are phrases like “yesterday,” “3 days ago,” “next week,” or “last Thursday.” strtotime() can handle these intuitively:
php $yesterday = strtotime("yesterday"); $threeDaysAgo = strtotime("3 days ago"); $nextWeek = strtotime("next week"); $lastThursday = strtotime("last Thursday");
Relative formats are particularly handy for creating dynamic date calculations in your applications.
2.2. Absolute Formats
strtotime() can also work with absolute date and time formats, such as:
- “2023-09-30 14:30:00”
- “2023-09-30”
- “14:30:00”
php $timestamp1 = strtotime("2023-09-30 14:30:00"); $timestamp2 = strtotime("2023-09-30"); $timestamp3 = strtotime("14:30:00");
The function is intelligent enough to recognize these formats and provide the corresponding Unix timestamps.
2.3. Combining Formats
What if you have a string with both a date and a relative element? strtotime() can handle that too:
php $nextMondayAtNoon = strtotime("next Monday 12:00:00");
This code snippet will give you the Unix timestamp for the upcoming Monday at noon.
3. Handling Timezones
Dealing with timezones is a common challenge in web development, especially when your applications cater to users across the globe. Fortunately, strtotime() has built-in support for timezones.
You can specify the timezone for the input string using the timezone parameter:
php $dateTimeString = "2023-09-30 14:30:00"; $timezone = new DateTimeZone("America/New_York"); $timestamp = strtotime($dateTimeString, 0, $timezone);
In this example, we’ve set the timezone to “America/New_York.” Now, when strtotime() parses the date and time string, it will consider it as being in the specified timezone.
4. Error Handling
It’s essential to consider error handling when working with date and time functions, as parsing date strings isn’t foolproof. strtotime() can return false if it fails to parse the input string. To handle errors gracefully, you can check the return value:
php $timestamp = strtotime("invalid date string"); if ($timestamp === false) { echo "Invalid date string"; } else { echo "Unix timestamp: " . $timestamp; }
This code snippet ensures that your application doesn’t break when it encounters invalid date/time strings.
5. Practical Examples
Now that we’ve covered the basics of strtotime(), let’s explore some practical examples of how you can use this function in your PHP applications.
Example 1: Scheduling Future Events
Imagine you’re building an event management system, and you want to allow users to schedule events for the future. strtotime() can help you calculate the exact date and time when an event should occur based on user input:
php $userInput = "2 weeks from now"; $eventTimestamp = strtotime($userInput); // Save $eventTimestamp to your database
In this example, the user inputs “2 weeks from now,” and strtotime() calculates the exact timestamp for that date. You can then store this timestamp in your database for future reference.
Example 2: Displaying Relative Time Ago
Many social media platforms display timestamps as “3 hours ago” or “a month ago.” You can achieve this easily using strtotime():
php $postTimestamp = strtotime("2023-09-15 10:00:00"); $currentTimestamp = time(); $elapsedSeconds = $currentTimestamp - $postTimestamp; if ($elapsedSeconds < 60) { $timeAgo = $elapsedSeconds . " seconds ago"; } elseif ($elapsedSeconds < 3600) { $minutes = floor($elapsedSeconds / 60); $timeAgo = $minutes . " minutes ago"; } elseif ($elapsedSeconds < 86400) { $hours = floor($elapsedSeconds / 3600); $timeAgo = $hours . " hours ago"; } else { $days = floor($elapsedSeconds / 86400); $timeAgo = $days . " days ago"; } echo $timeAgo;
This code calculates the time elapsed since a post was created and displays it in a user-friendly format.
Example 3: Managing Subscription Renewals
Suppose you run a subscription-based service, and you need to determine when a user’s subscription should renew. You can use strtotime() to calculate the renewal date:
php $subscriptionStart = strtotime("2023-09-01"); $subscriptionDuration = "1 year"; // The duration of the subscription $renewalTimestamp = strtotime("+" . $subscriptionDuration, $subscriptionStart); // Send a reminder to the user when it's time to renew
Here, we’ve specified the subscription’s start date and its duration as “1 year.” strtotime() then calculates the renewal date, allowing you to send a renewal reminder to the user.
6. Tips and Best Practices
To make the most of strtotime(), consider the following tips and best practices:
- Use Specific Formats: When working with absolute dates, provide the date and time in a format that leaves no room for ambiguity, like “YYYY-MM-DD HH:MM:SS.”
- Sanitize User Input: If you’re using strtotime() with user-provided input, ensure that the input is properly validated and sanitized to prevent security vulnerabilities.
- Combine with DateTime: For more advanced date and time manipulation, consider combining strtotime() with PHP’s DateTime class, which provides additional functionality and flexibility.
- Timezone Awareness: Always be mindful of timezones when working with date and time functions. Specify the timezone explicitly when necessary to avoid unexpected results.
- Error Handling: As mentioned earlier, check for false return values to handle parsing errors gracefully.
Conclusion
PHP’s strtotime() function is a powerful tool for working with dates and times in your web applications. Its ability to handle a wide range of date formats, work with timezones, and perform relative date calculations makes it a valuable asset for developers.
By mastering strtotime(), you can streamline date and time-related tasks, making your code more efficient and user-friendly. Whether you’re building event schedulers, displaying relative time ago, or managing subscriptions, strtotime() is a versatile and indispensable function in your PHP toolkit.
In your journey as a PHP developer, embracing strtotime() will undoubtedly simplify your date and time handling challenges, allowing you to focus on delivering exceptional user experiences in your web applications.
Table of Contents
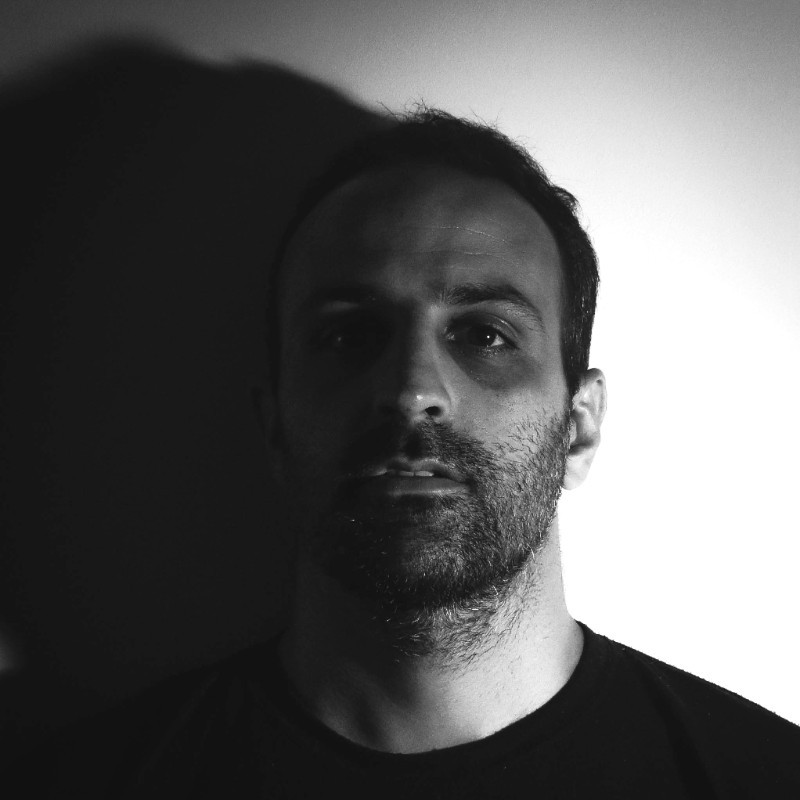
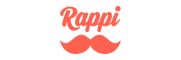