Exploring PHP’s strtotime(): Converting Strings to Dates
In the realm of web development, handling date and time is an indispensable task. Whether it’s displaying publication dates, scheduling events, or processing user input, dealing with dates is a fundamental aspect. PHP, a versatile scripting language widely used for web development, offers a powerful function called strtotime() that simplifies the conversion of date strings into more usable date formats. In this blog post, we’ll embark on a journey to explore the capabilities of strtotime(), from its basics to advanced usage, complete with code samples and practical applications.
1. Understanding strtotime() – A Brief Overview
strtotime() is a remarkable function in PHP that converts human-readable date and time expressions into Unix timestamps, which are integers representing the number of seconds since the Unix epoch (January 1, 1970, at 00:00:00 UTC). This ingenious function allows developers to convert a wide variety of date and time formats into a standard format that can be easily manipulated and formatted to suit different needs.
2. Basic Usage of strtotime()
Let’s start with the basics. The strtotime() function takes a string representing a date or time as its parameter and returns a Unix timestamp. Here’s a simple example:
php $dateString = "2023-08-19"; $timestamp = strtotime($dateString); echo "Unix Timestamp: " . $timestamp;
In this example, the date string “2023-08-19” is converted into a Unix timestamp, which represents August 19, 2023. This timestamp can then be used for various calculations and formatting tasks.
3. Date Expressions and Relative Formats
One of the most impressive features of strtotime() is its ability to understand a wide range of date expressions and relative formats. This flexibility enables developers to work with complex date strings without having to manually parse them. Let’s take a look at some examples:
3.1. Relative Formats
php $now = strtotime("now"); $tomorrow = strtotime("+1 day"); $nextWeek = strtotime("+1 week"); $nextMonth = strtotime("+1 month"); echo "Now: " . date("Y-m-d", $now) . "<br>"; echo "Tomorrow: " . date("Y-m-d", $tomorrow) . "<br>"; echo "Next Week: " . date("Y-m-d", $nextWeek) . "<br>"; echo "Next Month: " . date("Y-m-d", $nextMonth);
In this example, the strtotime() function understands the relative formats like “now,” “+1 day,” “+1 week,” and “+1 month.” The resulting Unix timestamps are then formatted using the date() function to display the corresponding dates.
3.2. Date Expressions
php $nextChristmas = strtotime("25 December"); $lastMonday = strtotime("last Monday"); $secondThursday = strtotime("second Thursday of August 2023"); echo "Next Christmas: " . date("Y-m-d", $nextChristmas) . "<br>"; echo "Last Monday: " . date("Y-m-d", $lastMonday) . "<br>"; echo "Second Thursday: " . date("Y-m-d", $secondThursday);
In this example, strtotime() understands date expressions like “25 December,” “last Monday,” and even more complex expressions like “second Thursday of August 2023.” This flexibility makes it incredibly useful for handling various date-related scenarios.
4. Dealing with User Input
When it comes to working with user-provided date input, things can get tricky due to the potential variability in formats. Thankfully, strtotime() can handle this efficiently by interpreting a wide range of formats. Let’s consider an example of parsing user-provided dates:
php $userInput = "08/19/2023"; $timestamp = strtotime($userInput); $formattedDate = date("Y-m-d", $timestamp); echo "User Input: " . $userInput . "<br>"; echo "Formatted Date: " . $formattedDate;
In this example, the user inputs a date in the “mm/dd/yyyy” format. The strtotime() function is capable of understanding this format and converting it into a Unix timestamp, which is then formatted into the “yyyy-mm-dd” format using the date() function.
5. Handling Ambiguities and Edge Cases
While strtotime() is incredibly versatile, it’s important to note that it might not handle all date formats perfectly, especially ambiguous formats like “03/04/2023.” In such cases, it might interpret “03” as the month and “04” as the day, leading to incorrect results. To mitigate this, you can rely on the DateTime class for more precise parsing and handling of edge cases.
6. Advanced Usage: Using strtotime() with Time Zones
strtotime() can also work with time zones, allowing you to perform conversions across different time zones seamlessly. By providing an additional parameter, you can specify the time zone for accurate conversions. Here’s an example:
php $dateString = "2023-08-19 15:00:00"; $timestamp = strtotime($dateString . " UTC"); echo "Original Timestamp: " . $timestamp . "<br>"; echo "Original Date: " . date("Y-m-d H:i:s", $timestamp) . "<br>"; // Convert to a different time zone $timestampNewYork = strtotime($dateString . " America/New_York"); echo "New York Timestamp: " . $timestampNewYork . "<br>"; echo "New York Date: " . date("Y-m-d H:i:s", $timestampNewYork);
In this example, we start with a date string in UTC and convert it to the New York time zone. The strtotime() function, along with specifying the desired time zone, handles the conversion effortlessly.
Conclusion
The strtotime() function in PHP is a versatile tool that simplifies the process of converting human-readable date and time expressions into Unix timestamps. Its ability to handle various date formats, relative expressions, and time zones makes it an indispensable asset in any developer’s toolkit. However, while strtotime() is powerful, developers should also be aware of its limitations and consider alternatives like the DateTime class for more complex scenarios. By harnessing the capabilities of strtotime(), developers can efficiently tackle date-related tasks and enhance the functionality and user experience of their web applications. So, the next time you find yourself wrestling with date strings, remember the magic of strtotime() and let it simplify your code and your life.
Table of Contents
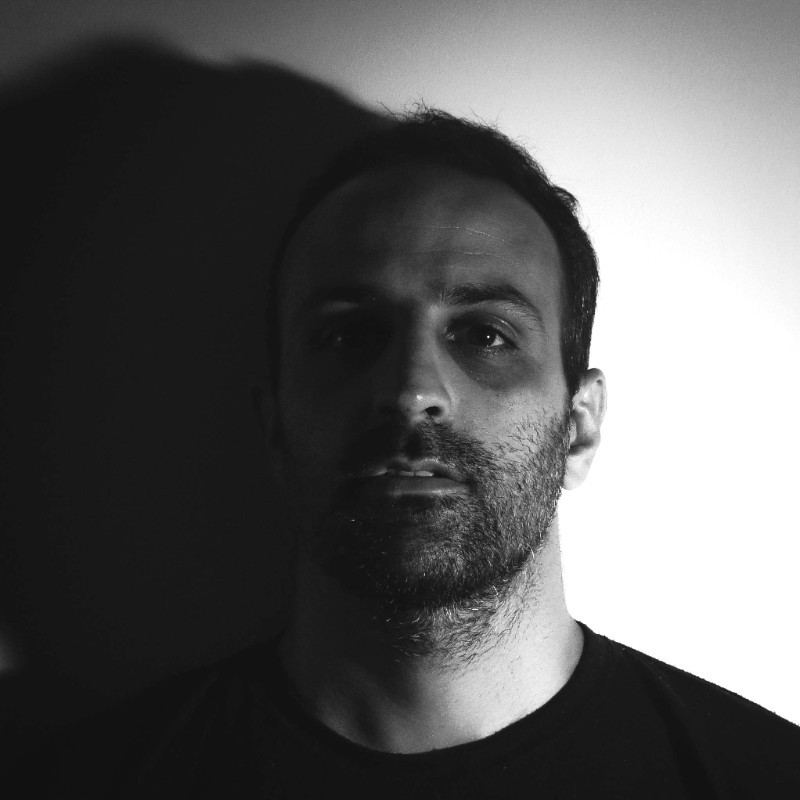
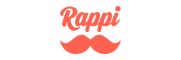