PHP’s strtoupper() Function: Converting Strings to Upper Case
In the world of web development, there are numerous situations where you may need to manipulate and format text strings. One common task is converting strings to uppercase, which can be essential for consistency in your application or for presenting text in a more visually appealing manner. In PHP, this task is made incredibly simple thanks to the strtoupper() function.
Table of Contents
In this blog post, we’ll dive deep into the PHP strtoupper() function, exploring how it works, when and why you might use it, and providing practical examples to help you understand its implementation. So, whether you’re a beginner or an experienced PHP developer, let’s sharpen our skills on converting strings to uppercase with strtoupper().
1. Understanding the strtoupper() Function
1.1. What is strtoupper()?
PHP’s strtoupper() function is a built-in function designed specifically for converting all alphabetic characters in a string to uppercase. It’s a straightforward way to ensure that text is consistently capitalized, which is particularly useful for maintaining data integrity or for presenting information uniformly.
1.2. Syntax of strtoupper()
The syntax for using strtoupper() is simple:
php strtoupper(string $string): string
Here, $string is the input string you want to convert to uppercase. The function returns a new string with all alphabetic characters in uppercase.
2. When to Use strtoupper()
Now that we know what strtoupper() does, let’s explore some common scenarios where you might find it valuable in your PHP applications.
2.1. Data Validation
When processing user input, it’s essential to validate and sanitize the data. Converting user-provided strings to uppercase ensures that data is consistent and easier to work with, reducing the risk of errors caused by variations in letter casing.
php $userInput = $_POST['username']; $uppercaseUsername = strtoupper($userInput); // Now $uppercaseUsername contains the username in uppercase.
2.2. Displaying Data
For presenting data in a more aesthetically pleasing manner, converting text to uppercase can make a significant difference. For instance, you might want to display names, titles, or headings in uppercase for emphasis.
php $name = "john doe"; $uppercaseName = strtoupper($name); echo "HELLO, $uppercaseName!"; // Output: HELLO, JOHN DOE!
2.3. Sorting Data
When working with arrays of strings, such as sorting names or titles, converting the strings to uppercase before sorting ensures that the sorting process is case-insensitive and produces the desired results.
php $names = ["Alice", "bob", "Charlie", "dave"]; sort($names); print_r($names); // Output: Array ( [0] => Alice [1] => Charlie [2] => bob [3] => dave )
To sort the array in a case-insensitive manner:
php $names = ["Alice", "bob", "Charlie", "dave"]; array_walk($names, function (&$value) { $value = strtoupper($value); }); sort($names); print_r($names); // Output: Array ( [0] => Alice [1] => bob [2] => Charlie [3] => dave )
2.4. Search Operations
Converting text to uppercase can be useful when performing case-insensitive search operations within strings or arrays.
php $haystack = "This is a sample string"; $needle = "sample"; if (strpos(strtoupper($haystack), strtoupper($needle)) !== false) { echo "Found!"; } else { echo "Not found!"; } // Output: Found!
3. Practical Examples of strtoupper()
Let’s dive deeper into practical examples of using the strtoupper() function in PHP.
Example 1: Uppercase Conversion
Suppose you have a user-provided title, and you want to ensure it’s displayed in uppercase for consistency. Here’s how you can achieve this:
php $title = "this is a sample title"; $uppercaseTitle = strtoupper($title); echo "Title: $uppercaseTitle"; // Output: Title: THIS IS A SAMPLE TITLE
Example 2: Sorting an Array
Imagine you have an array of product names that you want to sort alphabetically in a case-insensitive manner. You can do this by converting each element to uppercase before sorting:
php $products = ["apple", "Banana", "Cherry", "date"]; array_walk($products, function (&$value) { $value = strtoupper($value); }); sort($products); print_r($products); // Output: Array ( [0] => apple [1] => Banana [2] => Cherry [3] => date )
Example 3: Case-Insensitive Search
Suppose you want to check if a specific word exists within a longer string. By converting both the word and the string to uppercase, you can perform a case-insensitive search:
php $text = "This is a PHP tutorial"; $wordToFind = "php"; $uppercaseText = strtoupper($text); $uppercaseWord = strtoupper($wordToFind); if (strpos($uppercaseText, $uppercaseWord) !== false) { echo "The word \"$wordToFind\" was found!"; } else { echo "The word \"$wordToFind\" was not found."; } // Output: The word "php" was found!
4. Tips and Best Practices
When working with strtoupper(), consider these tips and best practices to make your code more robust and maintainable:
- Input Validation: Always validate user input before applying strtoupper() to avoid potential issues.
- Use Proper Encoding: Be mindful of character encodings, especially when working with non-ASCII characters. The behavior of strtoupper() may vary depending on the encoding.
- Avoid Unnecessary Conversions: Only convert to uppercase when it’s necessary. Overusing strtoupper() can make your code less readable and efficient.
- Consider Multibyte Strings: For multibyte character sets like UTF-8, use mb_strtoupper() for accurate results.
Conclusion
In the realm of PHP development, the strtoupper() function is a powerful tool for converting strings to uppercase. Whether you’re sanitizing user input, presenting data more attractively, sorting arrays, or performing case-insensitive searches, strtoupper() simplifies the process and ensures consistency in your application.
By understanding its syntax, knowing when to apply it, and following best practices, you can leverage strtoupper() to enhance your PHP projects. So, go ahead and capitalize on this handy function to take your web development skills to new heights!
Table of Contents
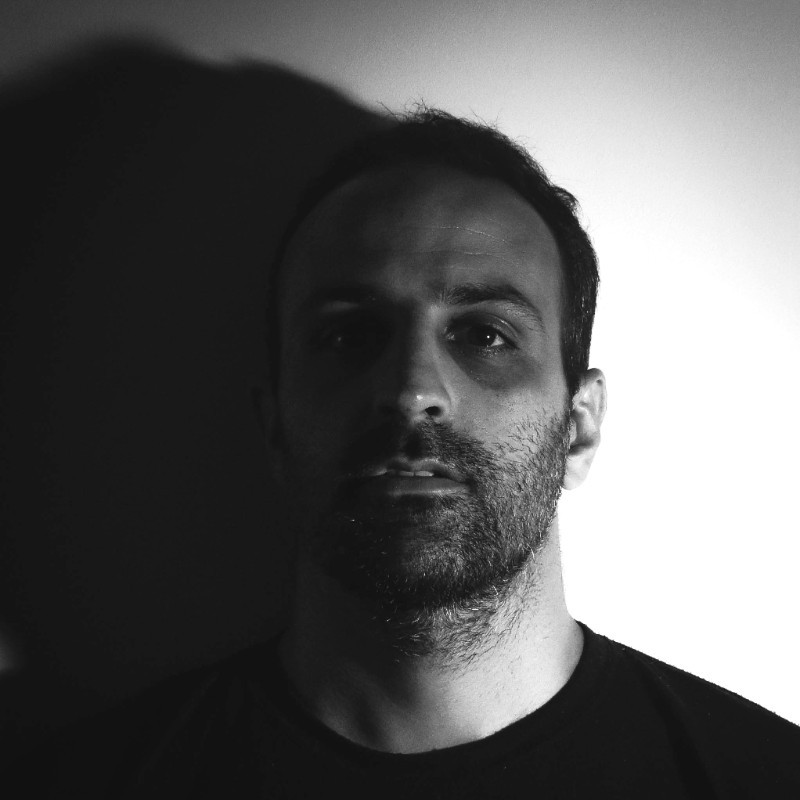
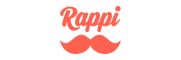