Working with PHP’s substr() Function: A Beginner’s Guide
If you’re a budding web developer diving into PHP programming, you’re likely to encounter scenarios where you need to manipulate strings. PHP offers a plethora of string functions to make your coding journey smoother, and one such versatile function is substr(). Short for “substring,” substr() allows you to extract a portion of a string based on your requirements. In this guide, we’ll take you through the ins and outs of working with PHP’s substr() function, along with practical examples and best practices.
1. Introduction to substr()
In PHP, the substr() function is a fundamental tool for string manipulation. It allows you to extract a portion of a given string, creating a new string containing only the selected part. This function is often employed to process data, manipulate text, or extract specific information from a larger string.
2. Syntax and Parameters
Before diving into examples, let’s familiarize ourselves with the syntax and parameters of the substr() function:
php substr(string $string, int $start, int|null $length = null): string
- string: The input string from which you want to extract the substring.
- start: The starting index from where you want to begin the extraction. This index is 0-based, meaning the first character has an index of 0.
- length (optional): The length of the substring you want to extract. If omitted, substr() will extract all characters from the starting index to the end of the string.
3. Extracting Substrings
Let’s explore some examples to grasp the usage of the substr() function.
Example 1: Basic Substring Extraction
Suppose you have a string that contains the name of a city, and you want to extract the first three characters:
php $city = "San Francisco"; $abbreviation = substr($city, 0, 3); echo $abbreviation; // Output: "San"
In this example, the function starts extracting from index 0 and continues for a length of 3 characters, resulting in “San.”
Example 2: Extracting with Negative Start
You can also use negative values for the starting index, which counts from the end of the string:
php $word = "Hello, World!"; $last_five = substr($word, -5); echo $last_five; // Output: "World!"
The function here starts extracting from the fifth-to-last character and continues to the end, giving us “World!”
Example 3: Extracting with Length Parameter
If you want to extract a substring of a specific length, you can provide the length parameter:
php $quote = "To be or not to be, that is the question."; $excerpt = substr($quote, 14, 12); echo $excerpt; // Output: "not to be, "
In this case, the substring starts at index 14 and spans 12 characters, resulting in “not to be, “.
4. Practical Applications
The substr() function finds its way into various real-world scenarios. Let’s explore a couple of them.
Example 4: Truncating Text for Display
Imagine you’re building a blog platform, and you want to display a snippet of each article on the homepage. You can use substr() to achieve this:
php $article = "In the heart of the enchanted forest, a great adventure unfolded..."; $snippet = substr($article, 0, 100) . "..."; echo $snippet; // Output: "In the heart of the enchanted forest, a great..."
By limiting the length of the extracted text, you create an engaging preview without overwhelming the reader.
Example 5: Parsing Data
Suppose you have a CSV (Comma-Separated Values) file, and you want to extract data from a specific column:
php $dataRow = "John,Doe,30,Developer"; $columns = explode(",", $dataRow); $name = $columns[0]; $occupation = $columns[3]; echo "Name: $name, Occupation: $occupation"; // Output: "Name: John, Occupation: Developer"
Here, we split the CSV row into columns using explode(), and then use substr() to extract specific values.
5. Handling Multibyte Characters
When dealing with languages that use multibyte characters (e.g., Chinese, Japanese), it’s crucial to consider character encoding. PHP offers the mb_substr() function for handling multibyte strings, ensuring accurate extraction without unintended character breaks.
6. Best Practices
To make the most of the substr() function, keep these tips in mind:
- Tip 1: Error Handling for Index Out of Bounds
If you attempt to extract a substring starting from an index beyond the length of the string, PHP will throw a warning. To avoid this, ensure your start index is within the string’s bounds.
- Tip 2: Using mb_substr() for Multibyte Strings
When working with languages that employ multibyte characters, rely on mb_substr() to prevent unintended character truncation. This function is sensitive to character encodings, ensuring accurate extraction.
Conclusion
PHP’s substr() function opens up a world of possibilities for string manipulation, from crafting previews of lengthy content to parsing data from structured text. With a solid grasp of its syntax, parameters, and best practices, you’re equipped to wield this function effectively in your projects. As you continue your PHP journey, remember that understanding and leveraging string functions like substr() will greatly enhance your coding capabilities. Happy coding!
Table of Contents
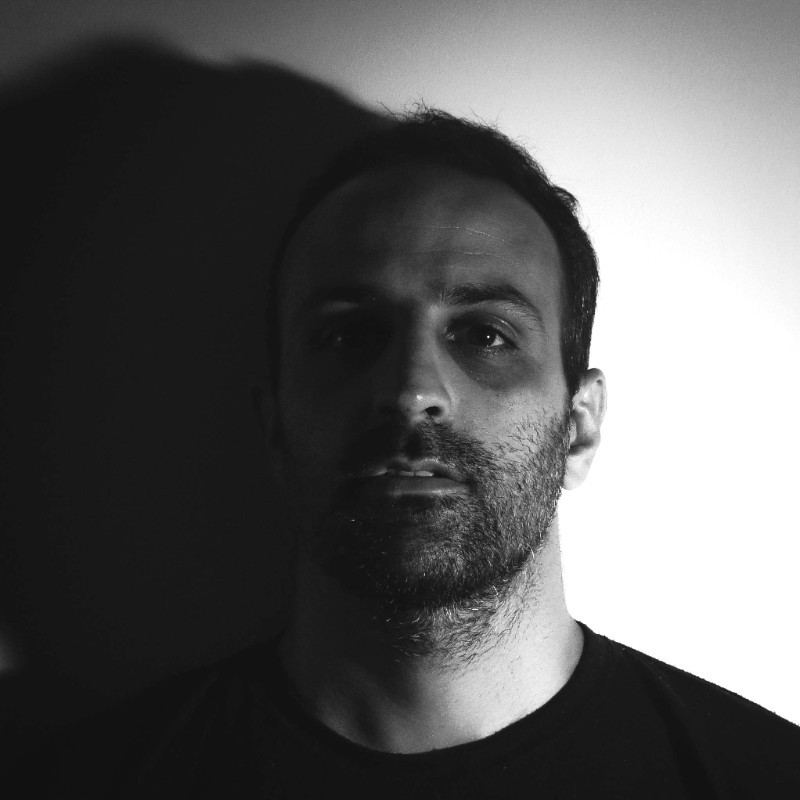
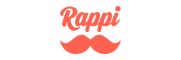