Getting Started with PHPUnit: Testing in PHP
Quality assurance is a crucial aspect of software development, ensuring that your code behaves as expected and minimizing the chances of bugs slipping through the cracks. One powerful tool in the arsenal of every PHP developer is PHPUnit—a unit testing framework that simplifies the process of writing and executing tests. In this guide, we’ll delve into the world of PHPUnit, from understanding the fundamental concepts to setting up your testing environment and writing effective tests.
1. What is PHPUnit?
PHPUnit is a widely-used testing framework for PHP. It’s designed to facilitate the creation and execution of unit tests, which are a type of testing that focuses on validating the smallest units of code in isolation. These units could be individual functions, methods, or classes. PHPUnit provides a set of tools and conventions that help developers write tests, execute them, and analyze the results.
2. Why PHPUnit?
Writing tests may seem like additional work, but it pays off significantly in the long run. Here are a few reasons why PHPUnit is a must-have tool in your PHP development toolbox:
- Bugs Prevention: By writing tests, you catch bugs early in the development process, reducing the risk of them reaching production.
- Code Refactoring: Tests provide a safety net when making changes to your codebase. You can confidently refactor or modify code knowing that your tests will identify if something breaks.
- Documentation: Tests serve as documentation for your code. They demonstrate how your code should be used and what behavior is expected.
- Collaboration: When working in teams, tests help ensure that everyone’s code integrates smoothly and doesn’t break existing functionality.
- Confidence: Writing tests gives you confidence that your code works as intended, even after numerous changes.
3. Setting Up PHPUnit
3.1. Installation
Getting started with PHPUnit is relatively simple. It’s recommended to install PHPUnit using Composer, a dependency management tool for PHP projects. If you don’t have Composer installed, head over to getcomposer.org and follow the installation instructions.
Once Composer is ready, open your terminal and navigate to your project’s root directory. Then, run the following command to install PHPUnit:
bash composer require --dev phpunit/phpunit
This command fetches the latest version of PHPUnit and adds it as a development dependency in your project.
3.2. Configuration
PHPUnit relies on a configuration file named phpunit.xml. You can generate this file using the following command:
bash ./vendor/bin/phpunit --generate-configuration
This command creates a basic phpunit.xml file in your project directory. You can customize this file to suit your testing needs. It allows you to define test directories, filter tests, set up bootstrap files, and configure various aspects of PHPUnit’s behavior.
4. Anatomy of a Test
Let’s explore the key components of a PHPUnit test.
4.1. Writing Test Cases
A test case in PHPUnit is a class that defines test methods. These methods are responsible for asserting the expected behavior of the code you’re testing. Each test method’s name typically starts with the word “test.”
Here’s a basic example of a test case:
php use PHPUnit\Framework\TestCase; class MathTest extends TestCase { public function testAddition() { $result = 2 + 2; $this->assertEquals(4, $result); } }
In this example, the MathTest class extends TestCase, which is provided by PHPUnit. The testAddition method asserts that the result of adding 2 and 2 equals 4 using the assertEquals assertion.
4.2. Assertions
Assertions are the core of testing. They allow you to verify if a certain condition is true. PHPUnit provides a wide range of assertions, including assertEquals, assertTrue, assertFalse, assertNull, and many more. These assertions help you validate your code’s behavior against expected outcomes.
4.3. Running Tests
To run your tests, execute the following command in your project’s root directory:
bash ./vendor/bin/phpunit
PHPUnit will discover and execute all the test cases in your project and provide a detailed report of the results.
5. Test Suites and Test Runners
As your project grows, you’ll likely have a significant number of test cases. Organizing these tests is crucial for maintainability. PHPUnit supports the concept of test suites and test runners.
A test suite is a collection of test cases. You can create a test suite by extending the TestSuite class or by using annotations. Test runners allow you to run specific test cases or suites instead of running all tests.
6. Data Providers
Data providers allow you to test the same piece of code with multiple sets of data. This is particularly useful when you want to ensure that your code behaves correctly under different circumstances.
php use PHPUnit\Framework\TestCase; class MathTest extends TestCase { /** * @dataProvider additionProvider */ public function testAddition($a, $b, $expected) { $result = $a + $b; $this->assertEquals($expected, $result); } public function additionProvider() { return [ [2, 2, 4], [0, 0, 0], [-1, 1, 0], ]; } }
In this example, the additionProvider method provides an array of input values and expected results. The testAddition method is executed for each set of data provided by the data provider.
7. Test Doubles
Test doubles are objects used in tests to stand in for real objects. PHPUnit supports several types of test doubles:
7.1. Mocks
Mocks are objects that simulate real objects but allow you to set expectations on their methods. You can define how many times a method should be called, what arguments it should receive, and what it should return.
7.2. Stubs
Stubs are simpler than mocks. They provide predefined responses to method calls and are useful when you want to isolate the code you’re testing from its dependencies.
7.3. Spies
Spies are similar to mocks but record information about method calls, such as the number of times a method was called and with what arguments.
8. Testing Exceptions
PHPUnit provides specialized assertions for testing exceptions. You can assert that a specific exception is thrown during the execution of a code block.
php use PHPUnit\Framework\TestCase; class ExceptionTest extends TestCase { public function testException() { $this->expectException(\InvalidArgumentException::class); $this->expectExceptionMessage('Invalid argument'); // Code that should throw an exception throw new \InvalidArgumentException('Invalid argument'); } }
9. Organizing Test Code
As your test suite grows, maintaining organization becomes crucial. Follow best practices for file and directory structure, such as keeping tests separate from production code and organizing them by functionality or module.
10. Continuous Integration and PHPUnit
Integrating PHPUnit into your continuous integration (CI) pipeline ensures that your tests are automatically executed whenever changes are pushed to your repository. Popular CI platforms like Jenkins, Travis CI, and GitHub Actions have built-in support for PHPUnit.
11. Best Practices for Effective Testing
- Keep Tests Independent: Tests should be isolated from each other to avoid dependencies and ensure consistent results.
- Test Edge Cases: Don’t just test common scenarios. Cover edge cases, boundary conditions, and unexpected inputs.
- Maintain Test Coverage: Strive for a high test coverage percentage to ensure you’re testing most of your codebase.
- Use Meaningful Test Names: Clear and descriptive test names make it easier to understand what’s being tested.
- Regularly Update Tests: As your codebase evolves, update your tests to reflect changes in functionality.
Conclusion
PHPUnit is a powerful tool for unit testing in PHP, allowing you to validate your code’s behavior, catch bugs early, and ensure the reliability of your applications. By following the concepts and practices outlined in this guide, you’ll be well-equipped to harness the full potential of PHPUnit and create robust, well-tested PHP code. Remember, effective testing is an investment that pays off by enhancing the quality of your software and streamlining your development process. Happy testing!
Table of Contents
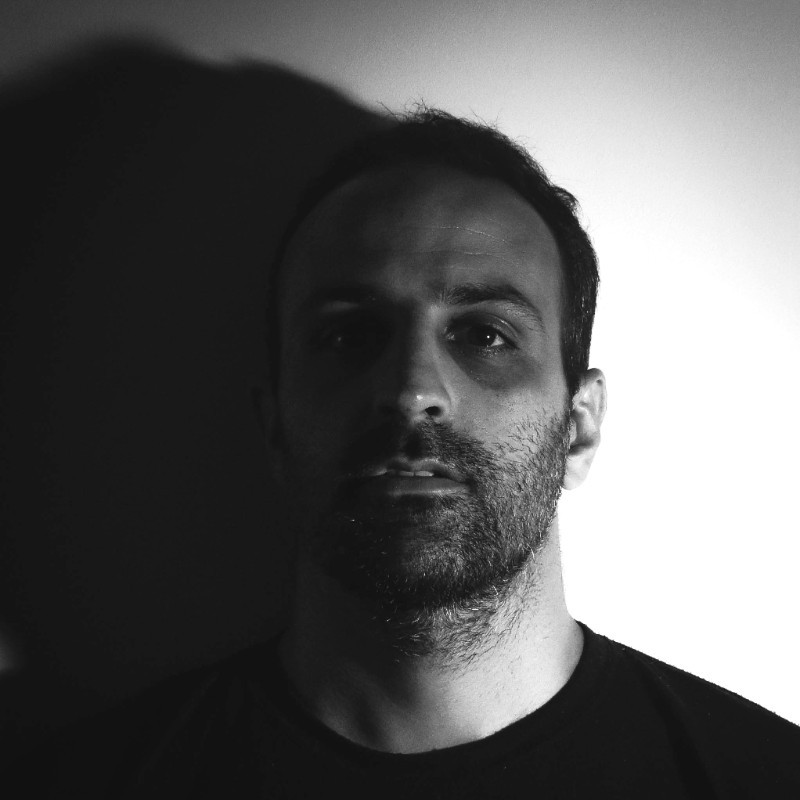
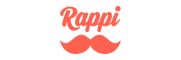