Exploring PHP’s ucfirst() Function: A Comprehensive Guide
PHP is a versatile programming language known for its wide range of built-in functions that simplify various tasks. One such function that comes in handy when dealing with string manipulation is ucfirst(). This function allows you to capitalize the first letter of a string, which can be useful in many situations. In this comprehensive guide, we’ll dive deep into PHP’s ucfirst() function, exploring its usage, examples, and best practices.
Table of Contents
1. Introduction to ucfirst()
1.1. What is ucfirst()?
The ucfirst() function in PHP is a built-in function used to capitalize the first letter of a string. It takes a string as input and returns a new string with the first letter capitalized.
php $string = "hello, world!"; $capitalizedString = ucfirst($string); echo $capitalizedString; // Outputs: "Hello, world!"
As you can see in the example above, ucfirst() transformed the lowercase “h” in “hello” to an uppercase “H,” leaving the rest of the string unchanged.
1.2. Why use ucfirst()?
There are several scenarios in web development and data processing where capitalizing the first letter of a string is necessary or improves the user experience. Some common use cases include:
- Usernames: When displaying usernames, you may want to ensure that the first letter is capitalized for a more polished look.
- Names: When dealing with names, it’s a common practice to capitalize the first letter to follow standard naming conventions.
- Titles and Headings: If you’re working on content management systems or generating titles and headings dynamically, you can use ucfirst() to ensure the first word is capitalized.
Now that we understand the basics, let’s delve into the syntax and parameters of the ucfirst() function.
2. Syntax and Parameters
2.1. Syntax of ucfirst()
The ucfirst() function in PHP has a simple syntax:
php ucfirst(string $str): string
$str (required): The input string you want to capitalize the first letter of.
2.2. Parameters
$str (required): This is the input string you want to modify. It can contain a single word or multiple words separated by spaces or other characters.
The function takes the input string and returns a new string with the first letter capitalized while preserving the case of the rest of the string. It’s important to note that ucfirst() does not modify the original string; instead, it returns the modified version.
3. Examples of Using ucfirst()
Let’s explore some practical examples of using ucfirst() to capitalize strings.
3.1. Capitalizing a Single Word
In this basic example, we will capitalize the first letter of a single word:
php $word = "apple"; $capitalizedWord = ucfirst($word); echo $capitalizedWord; // Outputs: "Apple"
3.2. Capitalizing Multiple Words
You can also use ucfirst() to capitalize the first letter of each word in a string. To achieve this, you can split the string into words, apply ucfirst() to each word, and then join them back together. Here’s how you can do it:
php $phrase = "the quick brown fox"; $words = explode(" ", $phrase); $capitalizedWords = array_map('ucfirst', $words); $capitalizedPhrase = implode(" ", $capitalizedWords); echo $capitalizedPhrase; // Outputs: "The Quick Brown Fox"
3.3. Handling Special Characters
ucfirst() works not only with letters but also with special characters. Here’s an example where it capitalizes the first letter of a string containing special characters:
php $string = "@php is awesome!"; $capitalizedString = ucfirst($string); echo $capitalizedString; // Outputs: "@php is awesome!"
In this case, the function capitalized the “@” symbol, resulting in no change to the rest of the string.
4. Best Practices
4.1. Sanitizing User Input
When working with user-generated content, it’s essential to sanitize and format data correctly. ucfirst() can be a helpful tool in ensuring that usernames, names, or other user-provided information are properly formatted.
Here’s an example of how you might use ucfirst() to format a username:
php $userInput = $_POST['username']; // Get the username from a form $cleanedUsername = ucfirst(strtolower($userInput)); // Ensure the first letter is capitalized, and the rest is in lowercase
By applying ucfirst() in combination with strtolower(), you ensure that the username is consistently formatted, regardless of how the user entered it.
4.2. Formatting Usernames
As mentioned earlier, formatting usernames is a common use case for ucfirst(). Let’s consider an example where we want to display a list of usernames with the first letter capitalized:
php $usernames = ["john_doe", "jane_smith", "admin_user"]; foreach ($usernames as $username) { $formattedUsername = ucfirst($username); echo $formattedUsername . "<br>"; }
This code snippet iterates through an array of usernames and displays them with the first letter capitalized. It results in a more visually appealing presentation of the usernames.
4.3. Displaying Names
When dealing with names in your application, it’s a best practice to capitalize the first letter for consistency and readability. Here’s how you might use ucfirst() to format a name:
php $fullName = "john doe"; $parts = explode(" ", $fullName); $formattedName = ucfirst($parts[0]) . " " . ucfirst($parts[1]); echo $formattedName; // Outputs: "John Doe"
This code splits the full name into first and last name, capitalizes the first letter of each, and then combines them back together.
5. Performance Considerations
While ucfirst() is a convenient function for capitalizing the first letter of a string, it’s essential to consider performance in scenarios where you need to process a large volume of data. In some cases, custom implementations may be more efficient.
5.1. Benchmarking ucfirst() vs. Custom Function
Let’s compare the performance of ucfirst() with a custom function for capitalizing the first letter of a string. We’ll use the microtime() function to measure execution time:
php // Using ucfirst() $start = microtime(true); for ($i = 0; $i < 1000000; $i++) { $word = "apple"; $capitalizedWord = ucfirst($word); } $end = microtime(true); $timeTaken = $end - $start; echo "Time taken using ucfirst(): " . $timeTaken . " seconds<br>"; // Using a custom function function customUcfirst($str) { return strtoupper(substr($str, 0, 1)) . strtolower(substr($str, 1)); } $start = microtime(true); for ($i = 0; $i < 1000000; $i++) { $word = "apple"; $capitalizedWord = customUcfirst($word); } $end = microtime(true); $timeTaken = $end - $start; echo "Time taken using custom function: " . $timeTaken . " seconds";
In this benchmark, we capitalize the word “apple” one million times using both ucfirst() and a custom function. While the performance difference may not be significant for small-scale operations, you should consider custom solutions for large-scale applications where performance optimization is crucial.
Conclusion
PHP’s ucfirst() function is a handy tool for capitalizing the first letter of strings, and it finds its use in various scenarios, such as formatting usernames, names, and titles. Understanding its syntax, parameters, and best practices can help you improve the consistency and readability of your web applications. However, for high-performance situations, it’s worth considering custom implementations to ensure optimal execution times. As with any PHP function, the key is to apply it appropriately to meet your specific requirements and enhance the user experience.
In summary, ucfirst() is a valuable addition to your PHP toolkit when it comes to string manipulation and formatting, and mastering its usage can make your web development tasks more efficient and user-friendly.
Table of Contents
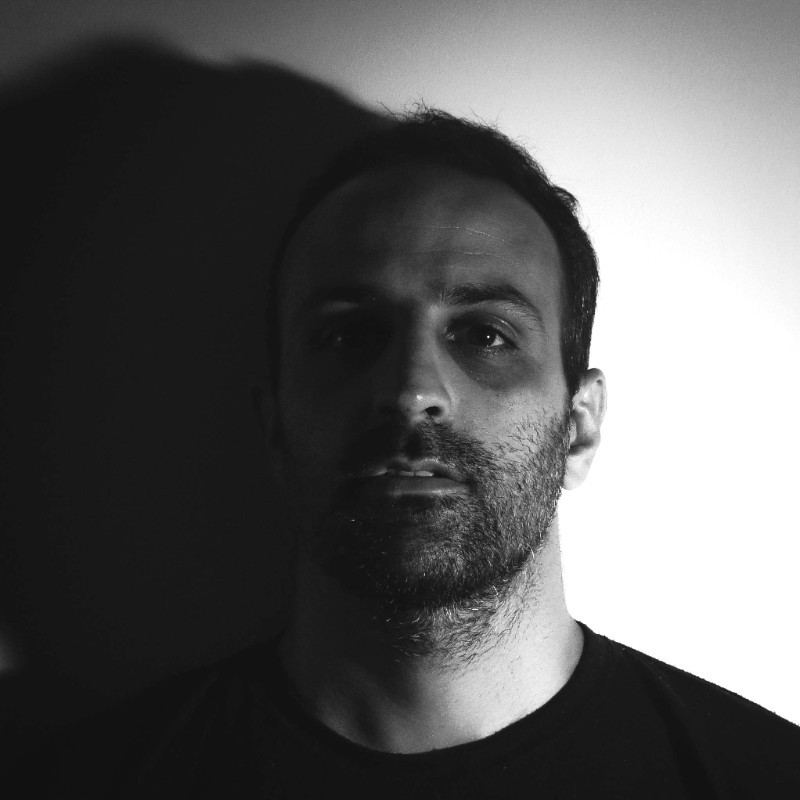
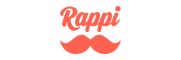