PHP’s ucwords() Function: Capitalizing Words in Strings
When it comes to text manipulation and formatting in PHP, there are numerous functions available at your disposal. One such function is ucwords(), which allows you to capitalize the first letter of each word in a given string. Whether you’re working on a web application, processing user input, or formatting text for display, ucwords() can be a handy tool in your PHP toolkit. In this blog post, we’ll delve into the details of PHP’s ucwords() function, exploring its usage, syntax, and real-world examples.
Table of Contents
1. Understanding the ucwords() Function
The ucwords() function in PHP is specifically designed for capitalizing words within a string. It follows a straightforward logic: it looks for word boundaries (spaces) within the input string and capitalizes the first letter of each word. This function is particularly useful when you need to present data in a more visually appealing and grammatically correct format.
1.1. Syntax of ucwords()
Before we dive into examples, let’s understand the syntax of the ucwords() function:
php ucwords(string $str, string $delimiters = " \t\r\n\f\v")
The ucwords() function accepts two parameters:
- $str (required): This is the input string that you want to capitalize words in.
- $delimiters (optional): This parameter allows you to specify the characters that define word boundaries within the string. By default, it considers spaces and common whitespace characters as delimiters.
Now, let’s explore some practical examples to see how this function can be applied.
Example 1: Basic Usage
php $inputString = "hello world"; $capitalizedString = ucwords($inputString); echo $capitalizedString;
In this example, we start with a simple input string, “hello world.” When we apply ucwords() to this string, it capitalizes the first letter of each word, resulting in the output: “Hello World.”
Example 2: Custom Delimiters
You can customize the delimiters used by ucwords() by providing the second parameter. Let’s say you want to capitalize words in a string where words are separated by hyphens and underscores:
php $inputString = "this-is_an-example"; $capitalizedString = ucwords($inputString, "-_"); echo $capitalizedString;
By specifying “-_” as the delimiters, the function will recognize both hyphens and underscores as word boundaries. As a result, the output will be: “This-Is_An-Example.”
Example 3: Handling Multiple Spaces
The ucwords() function can handle cases where there are multiple spaces between words in the input string. It will still capitalize the first letter of each word while ignoring the extra spaces:
php $inputString = " capitalize words with spaces "; $capitalizedString = ucwords($inputString); echo $capitalizedString;
In this example, the output will be: ” Capitalize Words With Spaces “—notice how the multiple spaces are preserved.
Example 4: Use Case in Form Processing
A common use case for ucwords() is in form processing when you want to ensure that user-submitted data is properly formatted. Let’s say you have a form where users enter their names, and you want to ensure that the names are displayed with the first letter of each word capitalized:
php // Simulating user input from a form $userFirstName = "john"; $userLastName = "doe"; // Capitalize user's first and last name $capitalizedFirstName = ucwords($userFirstName); $capitalizedLastName = ucwords($userLastName); // Display the formatted name echo "Hello, {$capitalizedFirstName} {$capitalizedLastName}!";
In this example, regardless of how the user enters their name (e.g., “john,” “JoHN,” or “JOHN”), the ucwords() function ensures that the name is displayed correctly as “John Doe.”
2. Best Practices and Tips
While ucwords() is a handy function, here are some best practices and tips to keep in mind when using it:
2.1. Validate Input
Before applying ucwords(), validate the input string to ensure it contains the expected data. This helps prevent unexpected results or errors.
2.2. Use Appropriate Delimiters
Choose the delimiters that match the structure of your input data. If your data uses unconventional word boundaries, customize the delimiters accordingly.
2.3. Chain Functions
You can chain multiple string functions together to perform various text transformations in a single line of code. For example, you can combine trim() to remove leading and trailing whitespace with ucwords() for capitalization.
php $inputString = " capitalize words with spaces "; $capitalizedString = ucwords(trim($inputString)); echo $capitalizedString;
2.4. Consider Multibyte Characters
If you’re working with multibyte character encodings like UTF-8, be aware that ucwords() may not behave as expected. Consider using the mb_convert_case() function for proper multibyte support.
Conclusion
PHP’s ucwords() function is a valuable tool for capitalizing words within strings, making it a useful asset in various text formatting scenarios. Whether you’re building web applications, processing user input, or working with data that requires consistent formatting, ucwords() simplifies the task of ensuring proper capitalization.
In this blog post, we’ve covered the function’s syntax, provided practical examples, and shared best practices to help you use ucwords() effectively in your PHP projects. By mastering this function, you can enhance the readability and presentation of your text data, delivering a more polished user experience.
Table of Contents
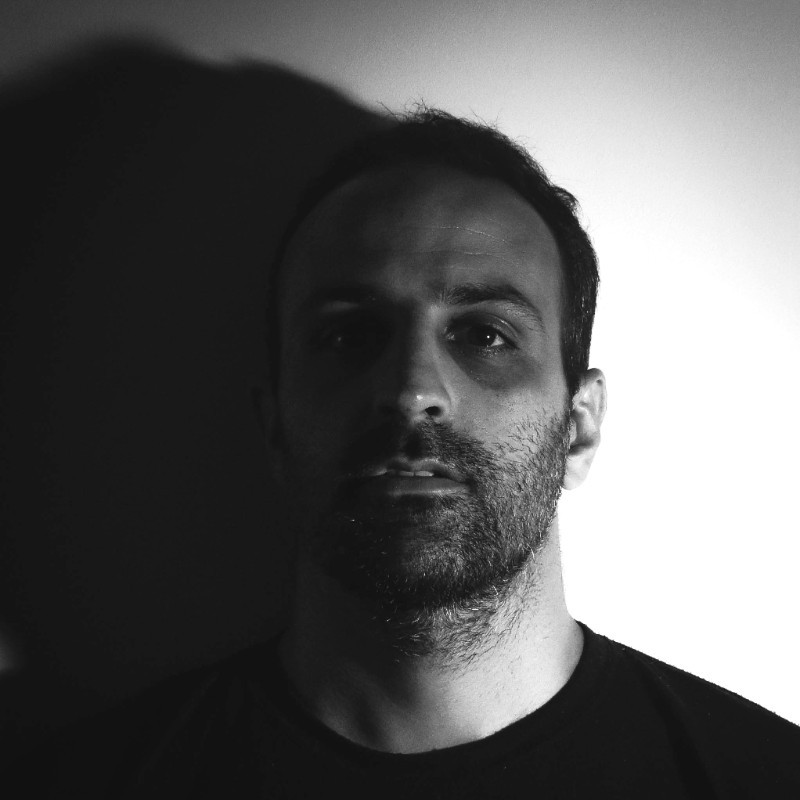
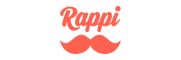