Advanced Topics in PHP: Understanding Namespaces
PHP, one of the most popular programming languages for web development, has evolved significantly over the years. With each version, new features and enhancements have been introduced to improve code quality, maintainability, and reusability. One such powerful feature that was added in PHP 5.3 is namespaces. Understanding namespaces is crucial for any PHP developer seeking to build scalable and modular applications. In this blog, we will dive deep into namespaces, exploring their benefits, implementation, and best practices.
1. What are Namespaces?
In PHP, namespaces provide a way to organize and categorize classes, functions, and constants to avoid naming conflicts between different code blocks. Namespaces allow developers to create a virtual grouping of related elements, making it easier to manage codebases and reuse components efficiently. This prevents collisions between different PHP libraries, packages, or frameworks that may share similar class or function names.
2. The Need for Namespaces
Before namespaces were introduced, developers faced several challenges when integrating third-party libraries or collaborating on large projects. Since PHP lacked the concept of namespaces, there was a high risk of naming collisions, making it difficult to integrate multiple libraries without extensive manual changes. This issue hindered code reusability and made code maintenance and debugging a nightmare. Namespaces were the much-needed solution to address these problems.
3. Declaring Namespaces
To declare a namespace in PHP, the namespace keyword is used. The namespace declaration must be the first statement in the PHP file, before any other code. Here’s an example of a basic namespace declaration:
php // file: my_file.php namespace MyNamespace; class MyClass { // class implementation }
In this example, we’ve created a namespace MyNamespace and placed our MyClass class inside it. Now, any class, function, or constant defined within this file belongs to the MyNamespace.
4. Using Namespaced Elements
Once a namespace is declared, we need to use the fully qualified name (FQN) of elements within that namespace when accessing them from outside the namespace. Alternatively, we can import elements using the use keyword to make the code more readable.
Let’s see how to access elements within a namespace:
php // file: another_file.php require_once 'my_file.php'; // Include the file with the namespace declaration $myObject = new MyNamespace\MyClass(); // Using the fully qualified name // OR use MyNamespace\MyClass; // Import the class using 'use' statement $myObject = new MyClass(); // Use the imported class without the namespace
Using use statements reduces the verbosity of code, making it more concise and readable.
5. Alias Imports
In addition to importing elements directly, we can also provide them with an alias to avoid naming conflicts in our code. This is especially useful when dealing with multiple namespaces or when two namespaces have elements with the same name.
php // file: yet_another_file.php use MyNamespace\MyClass as MyAlias; // Import and assign an alias to the class $myObject = new MyAlias(); // Use the aliased class
6. Global Namespace
In PHP, if a namespace is not explicitly defined, all classes, functions, and constants are placed in the global namespace. It’s essential to avoid polluting the global namespace with elements to prevent naming conflicts. Always prefer defining namespaces to encapsulate code effectively.
7. Nested Namespaces
Namespaces can be nested to create a hierarchical structure for better organization. This enables developers to group related code elements within multiple levels of namespaces.
php namespace MyNamespace\SubNamespace; class SubClass { // class implementation }
Here, we have a nested namespace SubNamespace inside the MyNamespace, and a class SubClass is placed within it.
8. Autoloading and Namespaces
Autoloading is a mechanism that automatically includes the necessary class files when they are needed in code. With namespaces, PHP introduced the concept of autoloading, making it easier to manage class files within different namespaces.
Autoloading can be achieved by using the spl_autoload_register() function or by leveraging the Composer package manager’s autoloader. Composer provides a convenient way to manage dependencies and autoload class files based on namespaces.
Here’s a basic example of autoloading using the spl_autoload_register() function:
php spl_autoload_register(function ($className) { $className = str_replace('\\', DIRECTORY_SEPARATOR, $className); require_once $className . '.php'; });
In this example, we convert the namespace separator \ to the directory separator based on the operating system. Then, we require the corresponding class file.
9. Best Practices for Using Namespaces
To make the most of namespaces in PHP, here are some best practices to follow:
- Consistent and Meaningful Namespaces: Choose namespaces that reflect the logical organization of your codebase. Use vendor names or project names as the base of your namespaces.
- Avoid Overusing Aliases: While aliases can be helpful in resolving naming conflicts, avoid excessive use of aliases, as it can lead to confusion and hinder code readability.
- One Class per File: Stick to the practice of having one class per file with a filename matching the class name. This simplifies autoloading and enhances code navigation.
- Use Namespace Autoloading: Embrace the use of autoloading to automate the inclusion of necessary files, reducing manual require statements.
- Follow PSR Standards: Adhere to the PHP-FIG PSR standards, especially PSR-1 (Basic Coding Standard) and PSR-4 (Autoloading Standard).
Conclusion
Namespaces in PHP offer a powerful way to organize code, promote reusability, and prevent naming collisions. By leveraging namespaces effectively, developers can build scalable, maintainable, and modular applications. Understanding namespaces is crucial for any PHP developer seeking to level up their skills and create efficient and robust codebases. Embrace the power of namespaces and elevate your PHP projects to the next level!
Table of Contents
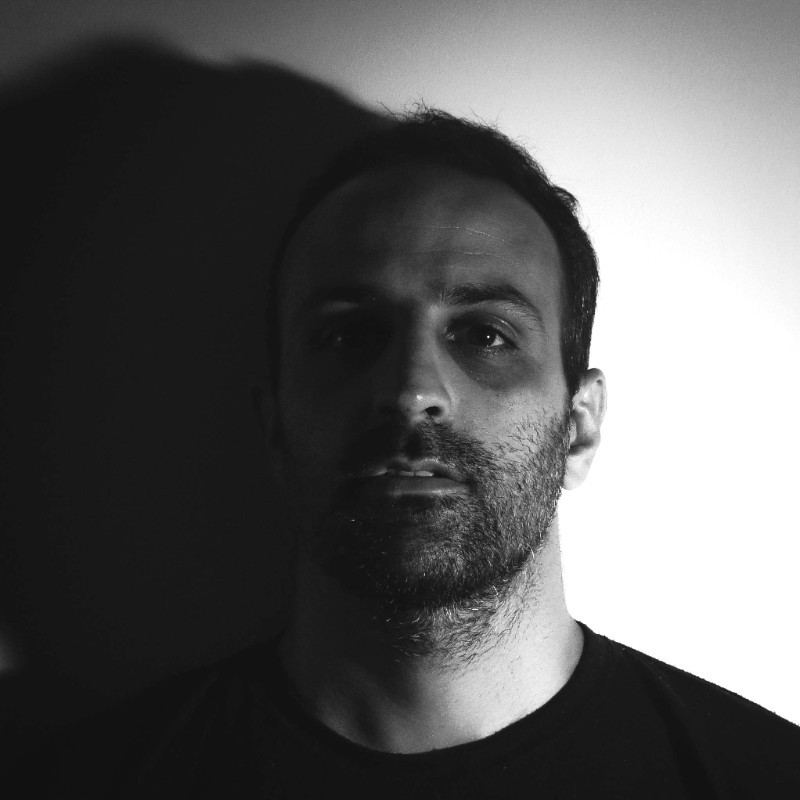
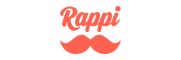