Getting the Most Out of PHP’s unserialize() Function
PHP, being one of the most widely used programming languages for web development, offers a plethora of built-in functions that simplify complex tasks. Among these is the unserialize() function, which plays a crucial role in handling serialized data. Serialization is the process of converting complex data structures, such as arrays and objects, into a string format that can be stored or transmitted easily. Unserialization, on the other hand, is the reverse process, converting the serialized string back into the original data structure. In this blog post, we’ll explore how to maximize the potential of PHP’s unserialize() function, leveraging its features for efficient and secure data manipulation.
1. Understanding Serialization and Unserialization
Before diving into the intricacies of the unserialize() function, let’s grasp the concept of serialization and unserialization. Serialization is employed to transform complex data structures into a linear format, usually a string, which can be easily stored in databases, transferred across networks, or cached. This enables developers to save memory and enhance data persistence.
serialize() is PHP’s companion function to convert data into a serialized format. For instance, consider an associative array:
php $data = [ 'name' => 'John Doe', 'age' => 30, 'email' => 'john@example.com' ]; $serializedData = serialize($data);
Now, let’s delve into unserialization.
2. The Anatomy of unserialize()
2.1. Basic Usage
The unserialize() function restores serialized data back into its original form. Here’s a basic example:
php $serializedData = 'a:3:{s:4:"name";s:8:"John Doe";s:3:"age";i:30;s:5:"email";s:17:"john@example.com";}'; $originalData = unserialize($serializedData); print_r($originalData);
In this example, we serialize an array containing a person’s name, age, and email, and then use unserialize() to convert it back to an array.
2.2. Handling Different Data Types
PHP’s unserialize() function is versatile and can handle various data types. These include strings, integers, floats, arrays, and objects. For instance:
php $serializedInt = 'i:42;'; $unserializedInt = unserialize($serializedInt); $serializedString = 's:12:"Hello, World!";'; $unserializedString = unserialize($serializedString); $serializedArray = 'a:2:{i:0;s:5:"apple";i:1;s:6:"orange";}'; $unserializedArray = unserialize($serializedArray); // Similarly, objects can be unserialized too
2.3. Dealing with Nested Structures
unserialize() is not limited to simple data structures; it can handle nested arrays and objects as well. Let’s consider an example where we serialize a nested data structure:
php $nestedData = [ 'person' => [ 'name' => 'Jane Smith', 'age' => 28 ], 'products' => ['apple', 'banana', 'cherry'] ]; $serializedNestedData = serialize($nestedData);
To unserialize this nested structure:
php $unserializedNestedData = unserialize($serializedNestedData); print_r($unserializedNestedData);
3. Error Handling and Security Measures
3.1. Handling Unserialization Failures
Unserialization may fail for various reasons, such as corrupted data or incompatible PHP versions. To handle such scenarios, it’s essential to wrap the unserialize() function call in a try-catch block:
php $serializedData = 'a:3:{s:4:"name";s:8:"John Doe";s:3:"age";i:30;s:5:"email";s:17:"john@example.com";}'; try { $originalData = unserialize($serializedData); print_r($originalData); } catch (Throwable $e) { echo 'Unserialization failed: ' . $e->getMessage(); }
3.2. Mitigating Security Risks
While unserialize() is a powerful tool, it can introduce security vulnerabilities if used recklessly. Attackers can exploit vulnerabilities by injecting malicious code into serialized data. To mitigate these risks:
- Input Validation: Validate and sanitize input before unserialization. Avoid unserializing user-provided data directly.
- Whitelisting: Maintain a whitelist of allowed classes that can be unserialized. This prevents unauthorized classes from being instantiated.
4. Best Practices for Effective Usage
4.1. Data Validation Before Unserialization
As mentioned earlier, validating data before unserialization is crucial for security. Only unserialize data from trusted sources and perform thorough data validation. For instance, if unserializing data from a file:
php $serializedData = file_get_contents('serialized_data.txt'); if ($serializedData !== false) { try { $originalData = unserialize($serializedData); print_r($originalData); } catch (Throwable $e) { echo 'Unserialization failed: ' . $e->getMessage(); } } else { echo 'Failed to read serialized data from file.'; }
4.2. Utilizing Custom Serialization
PHP provides the Serializable interface, which allows you to define custom serialization and unserialization methods for your classes. This can be particularly useful when dealing with complex objects. By implementing the Serializable interface, you can control the serialization and unserialization process:
php class CustomSerializableClass implements Serializable { private $data; public function __construct() { $this->data = 'Hello, World!'; } public function serialize() { return serialize($this->data); } public function unserialize($serialized) { $this->data = unserialize($serialized); } } $customObject = new CustomSerializableClass(); $serializedCustomObject = serialize($customObject); $unserializedCustomObject = unserialize($serializedCustomObject);
Conclusion
The unserialize() function is a powerful tool in PHP for handling serialized data, enabling efficient storage, transmission, and manipulation of complex data structures. By understanding its usage, handling errors, and adhering to security best practices, you can unlock its full potential. Remember to validate data before unserialization, be cautious of security risks, and leverage custom serialization methods when dealing with complex objects. Mastering unserialize() empowers you to efficiently manage data in your PHP applications while ensuring security and reliability.
Table of Contents
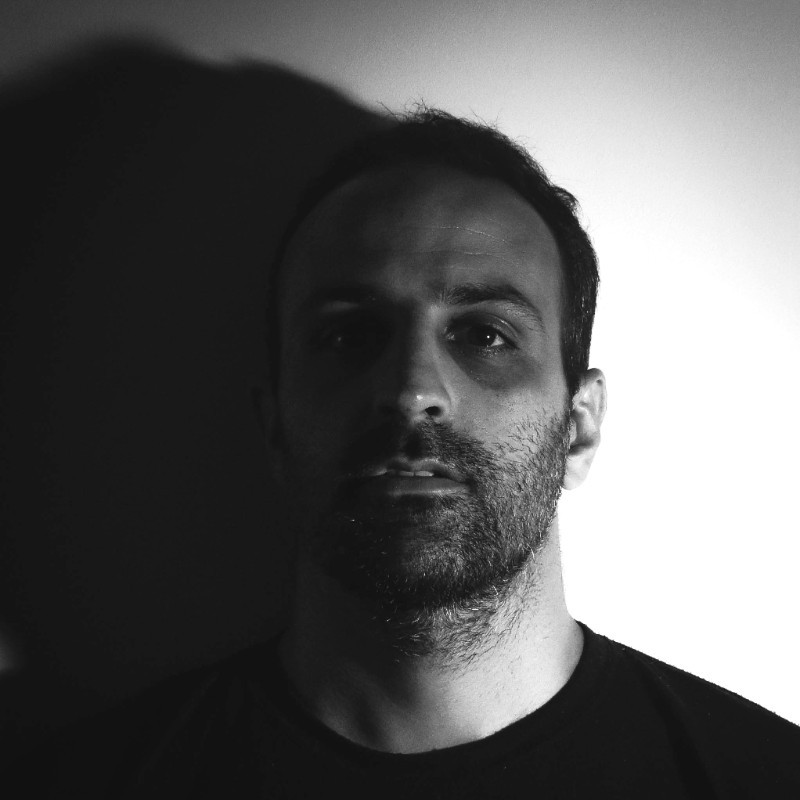
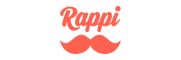