Implementing User Authentication with PHP
In today’s digital landscape, ensuring the security of user data is paramount. Websites and applications often handle sensitive information, making user authentication a crucial aspect of development. User authentication verifies the identity of users, granting access only to authorized individuals. PHP, a popular server-side scripting language, provides a robust framework for implementing effective user authentication. In this guide, we will explore the process of implementing user authentication using PHP, step by step.
1. Why User Authentication Matters
User authentication serves as the first line of defense against unauthorized access to your website or application. Without proper authentication mechanisms, anyone could gain access to restricted content or perform malicious actions. Implementing user authentication helps protect user accounts, sensitive data, and the overall integrity of your platform.
2. Setting Up Your Development Environment
Before diving into the implementation details, ensure you have a working PHP development environment. You can use tools like XAMPP, WAMP, or Docker to set up a local server. This will enable you to test and develop your authentication system without affecting your live website.
3. Creating the User Database
The foundation of user authentication lies in a well-structured database. You need to store user information securely, including usernames, passwords, and any additional relevant data. For this guide, we’ll use MySQL as the database system.
3.1. Designing the Database Schema
Start by designing the database schema. Create a table to store user information, including the user’s ID, username, hashed password, email, and any other relevant fields. Here’s a sample SQL script to create the user table:
sql CREATE TABLE users ( id INT PRIMARY KEY AUTO_INCREMENT, username VARCHAR(50) NOT NULL, email VARCHAR(100) NOT NULL, password VARCHAR(255) NOT NULL );
3.2. Hashing Passwords
It’s crucial to store passwords securely to prevent unauthorized access in case of a data breach. Instead of storing plain text passwords, we’ll store their hashed versions using a strong hashing algorithm like bcrypt.
php // When registering a new user $hashedPassword = password_hash($plainPassword, PASSWORD_BCRYPT); // Storing $hashedPassword in the database
4. Building the User Registration System
A user registration system allows users to create accounts on your platform. Here’s how you can implement it using PHP:
4.1. Registration Form
Create a registration form with fields for the user’s username, email, and password. Ensure that the form includes validation to prevent incorrect or malicious inputs.
html <form action="register.php" method="post"> <input type="text" name="username" placeholder="Username" required> <input type="email" name="email" placeholder="Email" required> <input type="password" name="password" placeholder="Password" required> <input type="password" name="confirm_password" placeholder="Confirm Password" required> <button type="submit">Register</button> </form>
4.2. Registration Logic (register.php)
In the register.php file, process the form submission and insert the user’s information into the database after proper validation.
php <?php if ($_SERVER["REQUEST_METHOD"] === "POST") { $username = $_POST["username"]; $email = $_POST["email"]; $password = $_POST["password"]; $confirmPassword = $_POST["confirm_password"]; // Validate inputs // Check if username and email are unique // Check if passwords match // Hash the password $hashedPassword = password_hash($password, PASSWORD_BCRYPT); // Insert data into the database // Redirect to login page or display success message } ?>
5. Creating the Login System
A login system enables users to access their accounts. Implementing it securely is essential to prevent unauthorized access.
5.1. Login Form
Design a login form with fields for the user’s username/email and password.
html <form action="login.php" method="post"> <input type="text" name="username_or_email" placeholder="Username or Email" required> <input type="password" name="password" placeholder="Password" required> <button type="submit">Login</button> </form>
5.2. Login Logic (login.php)
Handle the login process in the login.php file. Verify the user’s credentials against the database and create a session upon successful authentication.
php <?php session_start(); if ($_SERVER["REQUEST_METHOD"] === "POST") { $usernameOrEmail = $_POST["username_or_email"]; $password = $_POST["password"]; // Retrieve user data from the database based on username or email // Compare the hashed password from the database with the provided password if ($validCredentials) { $_SESSION["user_id"] = $userId; // Store user ID in the session header("Location: dashboard.php"); // Redirect to the dashboard exit(); } else { $error = "Invalid credentials. Please try again."; } } ?>
6. Implementing Session Management
PHP sessions are used to maintain user authentication across multiple pages during a browsing session. Here’s how you can manage sessions:
6.1. Verifying Authentication
On restricted pages, verify whether the user is authenticated before granting access.
php <?php session_start(); if (!isset($_SESSION["user_id"])) { header("Location: login.php"); // Redirect to the login page exit(); } ?>
6.2. Logout Functionality
Provide a logout option that destroys the user’s session and redirects them to the login page.
php <?php session_start(); session_destroy(); header("Location: login.php"); exit(); ?>
7. Enhancing Security
Implementing user authentication is just the beginning. To further enhance security, consider implementing the following measures:
- Password Recovery: Enable users to reset their passwords through a secure process involving email verification or security questions.
- Two-Factor Authentication (2FA): Implement 2FA to add an extra layer of security, requiring users to provide a secondary verification code in addition to their password.
- Session Timeout: Set a session timeout to automatically log out users after a period of inactivity.
Conclusion
In this guide, we’ve covered the fundamental steps of implementing user authentication using PHP. By creating a robust registration system, secure login mechanism, and effective session management, you can significantly enhance the security of your website or application. Remember that security is an ongoing process, so stay updated with best practices and emerging security trends to ensure your users’ data remains protected. As you continue to develop and refine your authentication system, you’ll create a safer and more reliable digital environment for your users.
Table of Contents
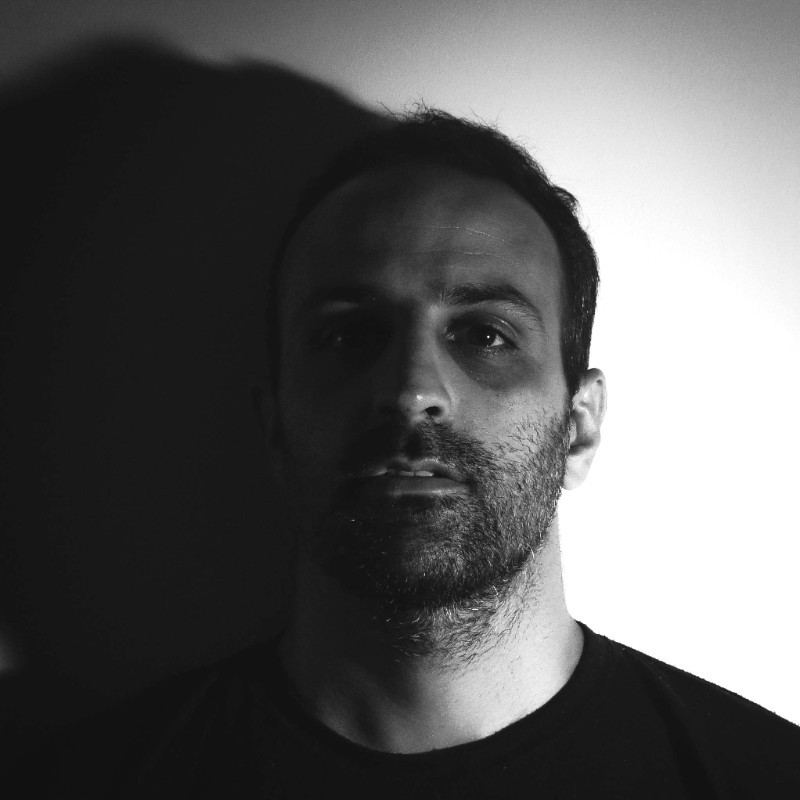
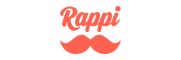