Using Composer with PHP: A Practical Guide
In the ever-evolving landscape of PHP development, managing project dependencies is a crucial aspect that can significantly impact productivity and code quality. Composer, a widely used PHP package manager, comes to the rescue by simplifying dependency management, allowing developers to focus on writing code rather than manually handling libraries and packages.
This practical guide will take you on a journey through the world of Composer, helping you understand its core concepts, installation process, and integration into PHP projects. By the end, you’ll have a solid grasp of Composer’s capabilities and how to leverage them effectively to enhance your PHP projects.
1. What is Composer?
1.1. Overview of Composer
Composer is a dependency management tool for PHP that allows you to declare, manage, and install external libraries, packages, and dependencies for your projects. It simplifies the process of adding third-party code into your PHP applications and ensures that all required dependencies are available and compatible.
1.2. Benefits of Using Composer
- Simplified Dependency Management: Composer enables you to specify project dependencies in a human-readable format, making it easy to add, update, and remove packages as needed.
- Automatic Class Autoloading: Composer automatically generates and manages the autoloading of classes, eliminating the need for manual include or require statements.
- Semantic Versioning Support: Composer supports semantic versioning, allowing you to define specific version constraints for each package, ensuring compatibility and preventing unexpected breaking changes.
- Vibrant Ecosystem: The PHP community actively maintains thousands of packages on Packagist, the default package repository for Composer, providing a rich ecosystem of reusable code for various purposes.
2. Getting Started with Composer
2.1. Installing Composer
Before diving into Composer, you need to install it on your system. The installation process varies depending on your operating system. For instance, on a Unix-based system, you can use the following commands:
bash curl -sS https://getcomposer.org/installer | php sudo mv composer.phar /usr/local/bin/composer
On Windows, you can download the installer from the Composer website and follow the on-screen instructions.
2.2. Composer’s Basic Commands
Composer offers a range of commands to manage your PHP project’s dependencies effectively:
- composer init: Creates a composer.json file interactively, helping you define your project’s metadata and initial dependencies.
- composer install: Installs all the packages defined in the composer.json file and their dependencies. It reads the file, resolves package versions, and downloads them into the vendor directory.
- composer require: Adds a new package to your project and automatically updates the composer.json file with the package information.
- composer update: Updates all the packages or a specific package to their latest versions, respecting the version constraints specified in the composer.json file.
2.3. Creating a composer.json File
To begin using Composer in your project, you need to create a composer.json file. This file serves as the heart of your project’s dependency management system, containing information about your project and its required packages.
2.3.1. Let’s create a basic composer.json file for a PHP project:
json { "name": "your-username/project-name", "description": "A brief description of your project", "type": "project", "license": "MIT", "authors": [ { "name": "Your Name", "email": "your@email.com" } ], "require": { "php": ">=7.3" } }
In this example, we’ve defined the project’s name, description, type, license, and the minimum PHP version required. The require section specifies that our project requires at least PHP 7.3 to run.
3. Managing Dependencies
3.1. Declaring Project Dependencies
To include external packages or libraries in your project, you can declare them in the require section of your composer.json file. Let’s say you want to include the popular Monolog library for logging in your project:
json { "require": { "php": ">=7.3", "monolog/monolog": "^2.0" } }
The ^2.0 notation signifies that you want to allow versions from 2.0.0 up to, but not including, 3.0.0. Composer will install the latest version of Monolog that fits this range.
3.2. Version Constraints and Semantic Versioning
Version constraints are essential to ensure that your project’s dependencies are compatible and don’t introduce breaking changes inadvertently. Composer follows Semantic Versioning (SemVer) for packages, which means each version is represented as MAJOR.MINOR.PATCH.
- MAJOR: A major version update includes backward-incompatible changes.
- MINOR: A minor version update includes backward-compatible new features.
- PATCH: A patch version update includes backward-compatible bug fixes.
- You can specify version constraints using comparison operators like >, >=, <, <=, and exact version numbers.
3.3. Resolving Package Versions
When you run composer install or composer update, Composer analyzes the composer.json file and attempts to resolve the best versions of all required packages, considering version constraints and inter-package dependencies. It ensures that all dependencies are compatible and satisfy the declared constraints.
3.4. Updating Dependencies
To update your project’s dependencies to their latest versions while respecting the version constraints, use the composer update command:
bash composer update
4. Autoloading Classes with Composer
4.1. Class Autoloading Explained
Class autoloading is a critical feature that eliminates the need to manually include or require PHP files containing class definitions. Composer leverages the PSR-4 autoloading standard, which associates namespaces with directory structures.
For example, if you have the following class namespace and directory structure:
php namespace MyApp\Util; class MyClass { // Class implementation }
You would typically place the MyClass.php file in the src/Util/ directory.
4.2. Configuring Autoloading in Composer
To enable class autoloading with Composer, you need to add the autoload section to your composer.json file. Specify the namespace and the corresponding directory where your class files are located:
json { "autoload": { "psr-4": { "MyApp\\": "src/" } } }
In this example, we’re telling Composer that classes under the MyApp namespace should be loaded from the src/ directory.
After adding the autoload section, run composer dump-autoload to generate the autoloader files:
bash composer dump-autoload
Now, whenever you use a class from the MyApp namespace in your code, Composer will automatically load the corresponding file.
5. Using Composer with Frameworks
5.1. Laravel and Composer
Composer is an integral part of Laravel, one of the most popular PHP frameworks. Laravel projects come with a pre-configured composer.json file, and developers can use Composer to add new dependencies easily.
For instance, to include the Laravel Debugbar package, which provides a beautiful debug bar for your Laravel applications, run:
bash composer require barryvdh/laravel-debugbar --dev
The –dev flag indicates that this package is only required for development and debugging purposes.
5.2. Symfony and Composer
Symfony, another well-known PHP framework, relies heavily on Composer for dependency management. When you create a new Symfony project using the Symfony CLI, Composer is automatically used to set up the project and fetch all the required components.
Symfony’s documentation provides detailed information on managing dependencies and using Composer effectively.
6. Handling Development and Production Dependencies
6.1. require vs. require-dev
The require section in the composer.json file is used to define packages that are essential for the project’s operation in both development and production environments. However, sometimes you may need additional packages for development or debugging purposes that aren’t required in production.
To separate development-only dependencies from production dependencies, use the require-dev section:
json { "require": { "php": ">=7.3", "monolog/monolog": "^2.0" }, "require-dev": { "phpunit/phpunit": "^9.0" } }
The packages specified under require-dev will not be installed in production environments when you run composer install –no-dev. This helps keep production environments lean and secure.
6.2. Optimizing Autoloading for Production
In a production environment, you can optimize autoloading to improve the performance of your PHP application. By default, Composer generates an autoloader that searches for classes across all the installed packages.
To optimize autoloading, use the –optimize flag when running composer install or composer update:
bash composer install --optimize
This flag generates a more efficient autoloader that reduces the number of file system operations when resolving classes.
7. Creating and Publishing Your Own Packages
7.1. Structuring a Package
Creating your own reusable package is a great way to contribute to the PHP community and share your code with others. To start, organize your package in a way that adheres to the PSR standards and is easy for others to integrate into their projects.
Consider the following package structure:
plaintext your-package/ src/ YourClass.php tests/ YourClassTest.php composer.json README.md
The src directory contains your package’s PHP classes, and the tests directory contains the corresponding test classes.
7.2. Defining Package Metadata
In your package’s composer.json file, define metadata such as the package’s name, description, and version:
json { "name": "your-username/your-package", "description": "A brief description of your package", "type": "library", "license": "MIT", "authors": [ { "name": "Your Name", "email": "your@email.com" } ], "require": { "php": ">=7.3" }, "autoload": { "psr-4": { "YourNamespace\\": "src/" } } }
7.3. Publishing on Packagist
To share your package with the world, you can publish it on Packagist, the default package repository for Composer. Create an account on Packagist (if you haven’t already) and submit your package. After approval, users can easily install your package using Composer.
8. Using Composer Plugins
8.1. What Are Composer Plugins?
Composer supports a rich ecosystem of plugins that extend its functionality. Plugins can add new commands, enhance the autoloader, or modify the behavior of existing commands.
8.2. Popular Composer Plugins and Their Functions
hirak/prestissimo: This plugin speeds up the installation process of Composer packages by allowing parallel downloads. It’s especially beneficial when working with large projects with numerous dependencies.
composer/package-versions-deprecated: This plugin helps you identify deprecated package versions in your composer.json file and recommends updating to the latest versions.
phpstan/phpstan: PHPStan is a powerful static analysis tool for PHP that helps you identify bugs and potential issues in your code. It can be integrated into your Composer workflow via this plugin.
Conclusion
Composer is a game-changer for PHP developers, providing an efficient way to manage dependencies and streamline the development process. With its ability to handle package installation, autoloading, and versioning, Composer significantly improves PHP projects’ organization and maintainability.
In this practical guide, we covered the basics of Composer, including installation, dependency management, autoloading, and integration with popular frameworks. We also explored the process of creating and publishing your own packages to contribute to the PHP community.
By mastering Composer, you’ll have a powerful tool at your disposal that will enhance your PHP projects and pave the way for more efficient and collaborative development.
Remember, Composer is just one of the many tools available to PHP developers, but it’s undoubtedly an essential one that you should add to your PHP development arsenal. So, start using Composer today and experience the benefits it brings to your PHP projects!
Table of Contents
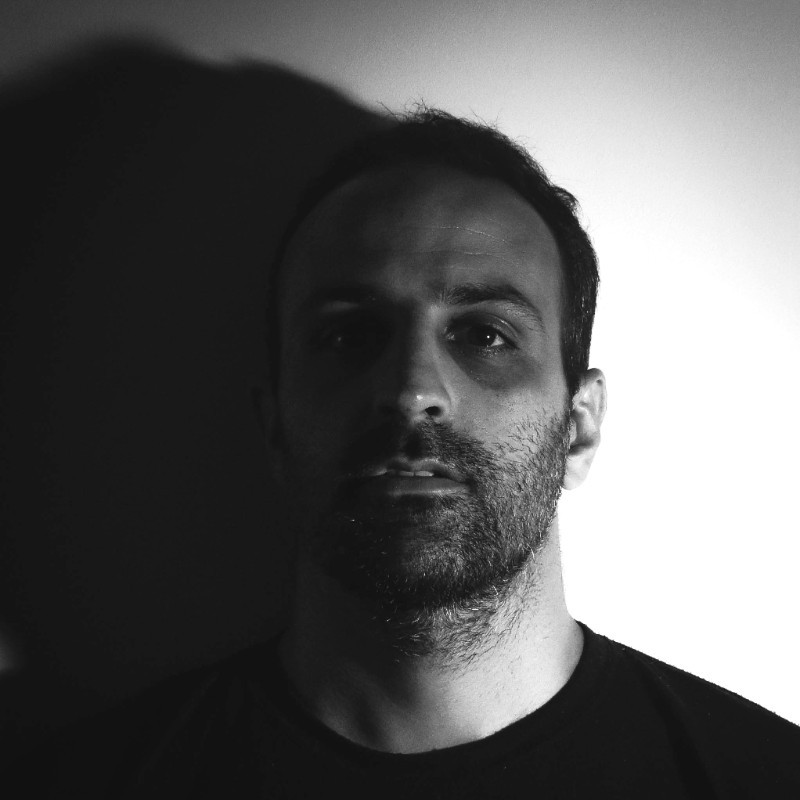
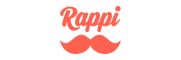