Using PHP in HTML: A Practical Guide
In the realm of web development, combining different technologies often leads to powerful and versatile solutions. One such dynamic duo is PHP and HTML. PHP (Hypertext Preprocessor) is a server-side scripting language that excels at generating dynamic content, while HTML (Hypertext Markup Language) forms the foundation of web pages. Integrating PHP into HTML allows developers to create interactive and data-driven websites that respond to user input and deliver personalized experiences.
In this practical guide, we’ll delve into the art of using PHP within HTML to create feature-rich web pages. We’ll explore the basics, advantages, and step-by-step examples of embedding PHP code within HTML documents.
1. Understanding the Relationship: PHP and HTML
Before we dive into the technical aspects, let’s understand the roles of PHP and HTML. HTML is the backbone of web pages, providing structure and content. It defines the elements that make up a page, such as headings, paragraphs, images, and links. On the other hand, PHP operates on the server-side and can generate dynamic content, interact with databases, and perform various calculations.
1.1. The Role of PHP
PHP acts as the behind-the-scenes worker that processes data and creates dynamic HTML content based on that data. For instance, it can generate personalized greetings based on a user’s name or retrieve and display the latest articles from a blog. PHP scripts are executed on the server before the page is sent to the user’s browser, ensuring that the user receives a customized experience.
1.2. The Structure of HTML
HTML provides the structure and layout of a web page. It consists of a series of tags that define different elements. Tags are enclosed in angle brackets, such as <html>, <head>, <title>, and <body>. Elements can also have attributes that provide additional information, like the href attribute in the <a> tag for hyperlinks. By combining various HTML elements, developers can create visually appealing and structured web pages.
2. Setting Up Your Development Environment
To start working with PHP in HTML, you need a local development environment. This environment simulates a web server on your computer, allowing you to test your PHP code before deploying it to a live server. Popular choices for local server setups include XAMPP, WAMP, and MAMP. These software packages come bundled with Apache (the web server), MySQL (the database), and PHP, forming the core components for web development.
2.1. Installing a Local Server
- XAMPP: Download XAMPP from the official website, and follow the installation instructions for your operating system. Once installed, you can start the Apache and MySQL services from the control panel.
- WAMP: Similarly, for Windows users, WAMP provides an easy installation process. After installation, launch the WAMP control panel to start the Apache and MySQL services.
- MAMP: Mac users can opt for MAMP. After installation, you can configure the Apache and MySQL settings through the MAMP application.
2.2. Creating Project Directories
After setting up your local server, create a directory where you’ll store your HTML and PHP files. This directory will serve as the root of your web development project. Organizing your project into folders and files helps maintain a clear structure as your project grows.
3. Embedding PHP within HTML
There are several ways to integrate PHP into your HTML files, ranging from simple inline PHP to more complex PHP sections. Let’s explore these techniques.
3.1. Using Inline PHP
Inline PHP involves embedding PHP code directly within HTML elements using the <?php … ?> tags. This method is useful for executing small snippets of PHP code within your HTML markup. For instance:
html <!DOCTYPE html> <html> <head> <title>Inline PHP Example</title> </head> <body> <h1><?php echo "Hello, World!"; ?></h1> <p><?php echo "Today is " . date("Y-m-d"); ?></p> </body> </html>
In this example, the PHP code within the <?php … ?> tags generates the current date and displays it within the paragraph element.
3.2. PHP Sections within HTML
For more extensive PHP code, you can create dedicated PHP sections within your HTML file. This approach enhances code readability and maintainability. Consider this example:
html <!DOCTYPE html> <html> <head> <title>PHP Section Example</title> </head> <body> <?php // PHP logic here $name = "Alice"; $age = 30; ?> <h1>Welcome, <?php echo $name; ?>!</h1> <p>You are <?php echo $age; ?> years old.</p> </body> </html>
In this instance, the PHP section above the HTML elements sets variables that are later used to personalize the welcome message.
3.3. Mixing HTML and PHP: The Do’s and Don’ts
While combining PHP and HTML offers immense flexibility, it’s important to follow best practices to maintain clean and readable code.
Do:
- Keep your PHP code organized and well-commented.
- Separate concerns by placing complex PHP logic outside of your HTML structure.
- Utilize PHP’s conditional statements and loops to create dynamic content.
Don’t:
- Avoid placing extensive PHP logic directly within HTML elements.
- Refrain from echoing large amounts of HTML from your PHP code; it can make your code hard to read.
- Minimize the use of PHP closing tags (?>) when you’re not switching back to HTML.
4. Passing Data between PHP and HTML
Interacting between PHP and HTML involves passing data back and forth. This is crucial for creating dynamic and interactive web pages.
4.1. Using Query Parameters
One way to pass data from HTML to PHP is through query parameters in the URL. Consider a scenario where you want to display a user’s name on a page:
html <!-- index.html --> <!DOCTYPE html> <html> <head> <title>Query Parameter Example</title> </head> <body> <a href="profile.php?name=Alice">View Alice's Profile</a> <a href="profile.php?name=Bob">View Bob's Profile</a> </body> </html>
In the above example, clicking on the links navigates to the profile.php page, passing the user’s name as a query parameter.
php // profile.php <!DOCTYPE html> <html> <head> <title>Profile Page</title> </head> <body> <?php $name = $_GET['name']; echo "<h1>Welcome to $name's profile!</h1>"; ?> </body> </html>
Here, PHP accesses the name query parameter using $_GET[‘name’] and dynamically displays the user’s profile name.
4.2. Form Submission and Handling
Forms are essential for user input and interaction. PHP can handle form submissions and process the submitted data.
html <!-- contact.html --> <!DOCTYPE html> <html> <head> <title>Contact Us</title> </head> <body> <form action="contact.php" method="post"> <label for="name">Name:</label> <input type="text" id="name" name="name" required><br> <label for="email">Email:</label> <input type="email" id="email" name="email" required><br> <button type="submit">Submit</button> </form> </body> </html>
The form above submits data to contact.php using the POST method.
php // contact.php <!DOCTYPE html> <html> <head> <title>Contact Form Submission</title> </head> <body> <?php if ($_SERVER["REQUEST_METHOD"] == "POST") { $name = $_POST['name']; $email = $_POST['email']; echo "<p>Thank you, $name, for contacting us at $email!</p>"; } ?> </body> </html>
In contact.php, PHP checks for POST requests and processes the submitted data, providing a thank you message.
4.3. Displaying Dynamic Data
Imagine you have a database of products, and you want to dynamically display them on a page.
php // products.php <!DOCTYPE html> <html> <head> <title>Product List</title> </head> <body> <?php $products = array( array("name" => "Product A", "price" => 19.99), array("name" => "Product B", "price" => 29.99), array("name" => "Product C", "price" => 9.99) ); foreach ($products as $product) { echo "<p>{$product['name']} - {$product['price']}</p>"; } ?> </body> </html>
In this example, PHP iterates through the $products array and generates HTML for each product.
5. Leveraging Control Structures
PHP’s control structures enhance the functionality of your dynamic web pages.
5.1. Conditional Statements (if, else, and elseif)
php // discount.php <!DOCTYPE html> <html> <head> <title>Discount Calculator</title> </head> <body> <?php $subtotal = 100; if ($subtotal > 50) { $discount = 10; } else { $discount = 5; } echo "Subtotal: $subtotal<br>"; echo "Discount: $discount%"; ?> </body> </html>
In this example, PHP calculates a discount based on the subtotal and uses an if-else statement to determine the discount amount.
5.2. Loops (for, while, foreach)
php // multiplication.php <!DOCTYPE html> <html> <head> <title>Multiplication Table</title> </head> <body> <?php $number = 5; echo "<h2>Multiplication Table for $number</h2>"; for ($i = 1; $i <= 10; $i++) { $result = $number * $i; echo "$number x $i = $result<br>"; } ?> </body> </html>
The above example demonstrates using a for loop to generate a multiplication table.
6. Organizing Code Effectively
To maintain a clean and maintainable codebase, follow best practices for code organization.
6.1. Separation of Concerns
Divide your code into logical sections: HTML for structure and presentation, PHP for dynamic logic, and CSS for styling. This separation makes debugging and maintenance easier.
6.2. Including External PHP Files
Break your code into smaller, reusable components by placing PHP code in separate files. You can then include these files in your main HTML document using the include or require statements.
6.3. Modular Approach for Maintainability
Instead of writing all your code in a single file, modularize your application. Each module can focus on specific functionality, which improves code readability and makes it easier to collaborate with other developers.
7. Best Practices for Security
When integrating PHP into HTML, security is paramount. Follow these best practices to ensure the safety of your application.
7.1. Input Validation and Sanitization
Always validate and sanitize user inputs before using them in your PHP code. This prevents potential security vulnerabilities like SQL injection or cross-site scripting (XSS) attacks.
7.2. Preventing SQL Injection
When interacting with databases, use prepared statements or parameterized queries to prevent SQL injection. These techniques ensure that user inputs are treated as data, not executable code.
7.3. Escaping Output to Prevent Cross-site Scripting (XSS)
Escape user-generated content before displaying it on your web pages. This prevents malicious scripts from being executed and protects your users from XSS attacks.
Conclusion
In conclusion, harnessing the power of PHP within HTML opens up a world of possibilities for creating dynamic and interactive web experiences. By understanding the symbiotic relationship between PHP and HTML, setting up a development environment, and following best practices, you can seamlessly integrate server-side functionality into your web pages. Whether it’s displaying dynamic data, processing user input, or creating personalized experiences, PHP empowers you to elevate your web development projects to new heights. So dive in, experiment with code samples, and watch your static HTML pages come to life with the magic of PHP!
Table of Contents
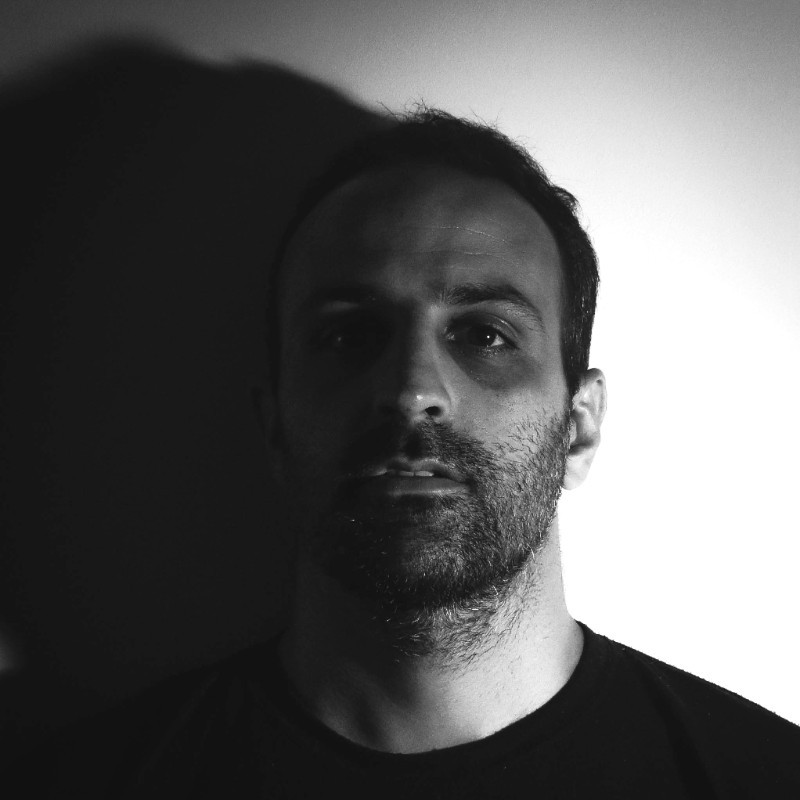
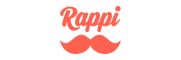