PHP’s usort() Function: A Practical Guide
Sorting arrays is a fundamental operation in programming, and PHP provides a versatile tool to achieve this with the usort() function. This function allows you to sort an array using a custom comparison function, giving you the flexibility to implement complex sorting logic tailored to your specific needs. In this guide, we’ll dive into the practical aspects of using usort() to effectively organize your data.
Table of Contents
1. Understanding the Basics of Sorting in PHP
Sorting arrays is a common operation when working with data. PHP offers a variety of sorting functions like sort(), asort(), and rsort() that are suitable for simple sorting needs. However, when you encounter scenarios where more complex sorting logic is required, the usort() function becomes invaluable.
1.1. How Does usort() Differ?
Unlike the conventional sorting functions, usort() lets you define a custom comparison function to determine the order of elements in an array. This opens up a world of possibilities, as you’re not limited to comparing values directly. Instead, you can implement intricate comparisons based on multiple attributes or conditions.
2. Syntax and Parameters of usort()
Before delving into examples, let’s break down the syntax and parameters of the usort() function.
php bool usort( array &$array, callable $comparison_function )
Here’s a brief explanation of the parameters:
- $array: The array you want to sort.
- $comparison_function: A callable that defines the custom comparison logic.
2.1. The Role of the Comparison Function
The comparison function plays a crucial role in determining the sorting order. It should accept two parameters (typically referred to as $a and $b) representing the elements being compared. The function should return an integer:
- A negative value if $a should come before $b.
- Zero if $a and $b are considered equal in terms of sorting.
- A positive value if $a should come after $b.
php function customComparison($a, $b) { // Custom comparison logic if ($a < $b) { return -1; } elseif ($a > $b) { return 1; } else { return 0; } }
3. Writing a Custom Comparison Function
Let’s take a practical example to illustrate how to write a custom comparison function. Suppose you have an array of objects representing products, and you want to sort them by their prices in ascending order.
php class Product { public $name; public $price; public function __construct($name, $price) { $this->name = $name; $this->price = $price; } } $products = [ new Product('Widget A', 25), new Product('Widget B', 15), new Product('Widget C', 40) ];
Now, we can define a comparison function to sort the products based on their prices:
php function compareByPrice($a, $b) { return $a->price - $b->price; } usort($products, 'compareByPrice');
The $products array will now be sorted in ascending order of prices.
4. Sorting Arrays of Different Data Types
The flexibility of usort() isn’t limited to arrays of objects. You can apply it to arrays containing various data types, such as strings, numbers, or a combination of both. Just tailor your comparison function to suit the data type you’re working with.
Suppose you have an array of strings that represent dates in the format ‘YYYY-MM-DD’, and you want to sort them chronologically:
php $dates = ['2023-05-15', '2022-11-03', '2023-01-20']; function compareDates($a, $b) { return strtotime($a) - strtotime($b); } usort($dates, 'compareDates');
5. Error Handling and Considerations
When using usort(), it’s important to handle potential errors that might arise from the comparison function. If the comparison function fails to provide valid integer return values, the sorting process could lead to unexpected results.
Additionally, keep performance considerations in mind, especially when dealing with large arrays. Custom comparison functions can introduce overhead, so it’s a good practice to optimize your code for efficiency.
6. Real-world Examples of usort()
Example 1: Sorting an Array of Associative Arrays
Consider an array of associative arrays representing students and their scores. You want to sort the students based on their scores in descending order:
php $students = [ ['name' => 'Alice', 'score' => 85], ['name' => 'Bob', 'score' => 92], ['name' => 'Eve', 'score' => 78] ]; function compareByScore($a, $b) { return $b['score'] - $a['score']; } usort($students, 'compareByScore');
Example 2: Sorting Using Multiple Criteria
Suppose you have an array of employees, and you want to sort them by department and then by salary within each department:
php class Employee { public $name; public $department; public $salary; public function __construct($name, $department, $salary) { $this->name = $name; $this->department = $department; $this->salary = $salary; } } $employees = [ new Employee('John', 'HR', 60000), new Employee('Jane', 'IT', 75000), new Employee('Smith', 'IT', 70000), new Employee('Emily', 'HR', 55000) ]; function compareByDepartmentAndSalary($a, $b) { if ($a->department !== $b->department) { return strcmp($a->department, $b->department); } return $b->salary - $a->salary; } usort($employees, 'compareByDepartmentAndSalary');
Conclusion
The usort() function in PHP is a powerful tool that gives you the flexibility to sort arrays using custom comparison logic. By understanding its syntax, parameters, and writing effective comparison functions, you can confidently tackle a wide range of sorting scenarios, from simple to complex. Whether you’re working with arrays of objects, associative arrays, or other data types, usort() empowers you to organize your data in ways that align with your specific needs. Experiment with different scenarios, explore real-world examples, and unlock the full potential of sorting with usort() in your PHP projects.
Table of Contents
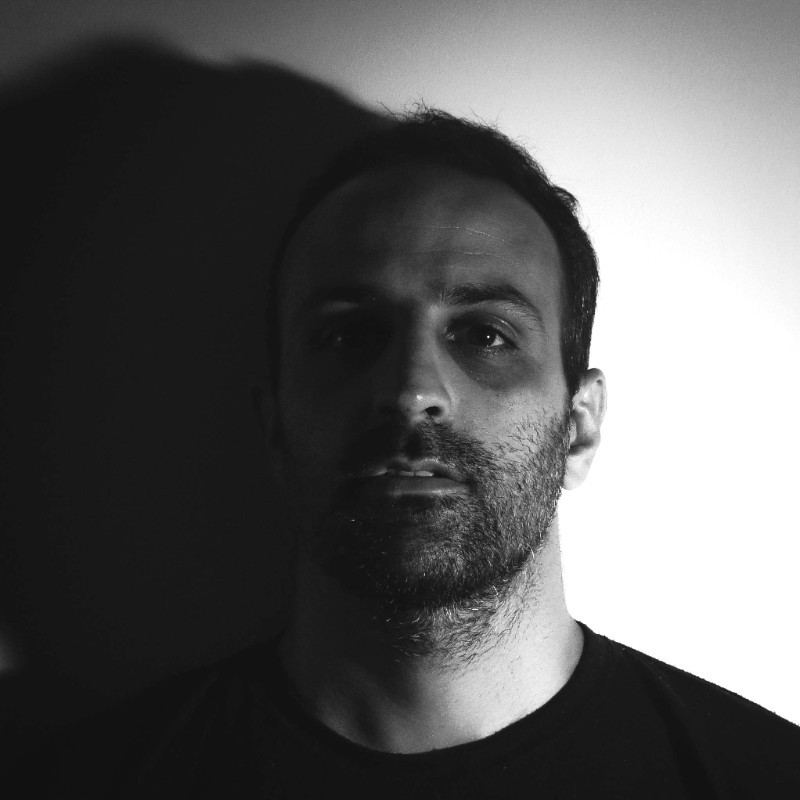
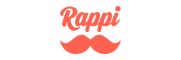