Getting the Most Out of PHP’s var_dump() Function
When it comes to debugging and understanding the inner workings of your PHP code, the var_dump() function is an indispensable tool in your toolkit. Whether you’re a seasoned developer or just starting with PHP, this function can significantly expedite the debugging process and help you gain valuable insights into the structure and values of variables. In this article, we’ll dive deep into the capabilities of the var_dump() function, exploring various features, formatting options, and scenarios where it proves to be an invaluable asset.
Table of Contents
1. Understanding the Basics:
Before we delve into advanced usage, let’s cover the basics of the var_dump() function. At its core, var_dump() is a debugging function in PHP that provides a detailed representation of one or more variables. This output includes the variable’s type, value, and length (for strings and arrays). It’s an excellent alternative to print_r() when you need more comprehensive information about variables.
2. Getting Started:
To use var_dump(), simply pass the variable you want to inspect as an argument. Let’s consider a basic example:
php $sampleArray = [1, 2, 3, 4, 5]; var_dump($sampleArray);
When you run this code, you’ll get an output that displays the variable’s type, size, and individual elements in the array. This can be incredibly helpful when you’re dealing with complex data structures and want a quick way to see their contents.
3. Digging Deeper with Formatting:
While the basic output of var_dump() is informative, the real power lies in its formatting options. Let’s explore some formatting techniques to make the output even more readable.
3.1. Using the var_dump() Return Value:
You can capture the output of var_dump() into a variable using output buffering. This allows you to use the generated information in your code rather than directly printing it to the screen. This can be useful when you want to log debugging information or conditionally display the output.
php $data = [1, 2, 3]; ob_start(); var_dump($data); $dumpOutput = ob_get_clean(); // Now you can use $dumpOutput as needed
3.2. Highlighting with <pre> Tag:
To improve the readability of the output, enclose the var_dump() inside a <pre> tag. This preserves the formatting and newlines, making the output more structured and easier to follow.
php echo '<pre>'; var_dump($data); echo '</pre>';
3.3. Custom Styling:
If you’re working on a web application, you can apply CSS styles to the <pre> tag to enhance the appearance of the var_dump() output. This is especially helpful if you want to match your application’s design aesthetics.
4. Unveiling Hidden Details:
As you become more comfortable with var_dump(), you’ll discover its ability to reveal hidden details about variables that might not be immediately apparent. Here are a couple of scenarios where var_dump() shines:
4.1. Object Exploration:
When dealing with objects, var_dump() unveils not only the public properties but also the private and protected ones. This is immensely helpful for understanding the object’s internal state and the values stored within.
php class MyClass { public $publicProperty = 'public'; private $privateProperty = 'private'; protected $protectedProperty = 'protected'; } $obj = new MyClass(); var_dump($obj);
4.2. Data Discrepancies:
var_dump() can be your best friend when you’re trying to identify discrepancies in variable values. If you’re encountering unexpected behavior, using var_dump() to inspect variables at crucial points in your code can shed light on the issue.
5. Advanced Tips and Tricks:
As you master the fundamentals, consider these advanced tips to further elevate your debugging game:
5.1. Recursive Limit:
By default, var_dump() doesn’t place a limit on recursive depth when inspecting nested arrays and objects. This can lead to overwhelming output, especially with complex data structures. To control the recursive depth, use the xdebug.var_display_max_depth configuration directive in your PHP.ini file.
ini xdebug.var_display_max_depth = 3
This limits the depth of nested elements to three levels.
5.2. Data Types and Values:
The output of var_dump() includes detailed information about data types and values. This can be indispensable when you’re working with type casting or conversion and need to ensure that your data is being processed correctly.
5.3. Conditional Dumping:
To avoid flooding your output with excessive information, you can use conditional statements to selectively display the output of var_dump(). This is particularly useful when you’re dealing with loops or repetitive structures.
Conclusion
In the realm of PHP debugging, the var_dump() function stands as a reliable companion for unraveling the mysteries of your code. From basic variable inspection to in-depth object exploration, var_dump() equips you with the tools to efficiently diagnose issues, ensure data integrity, and streamline your development process. By harnessing its formatting options and diving into its advanced capabilities, you’ll elevate your debugging prowess and become a more effective PHP developer. So, the next time you find yourself tangled in the web of PHP code, remember that var_dump() is your trusted ally in the journey towards bug-free, optimized code.
Table of Contents
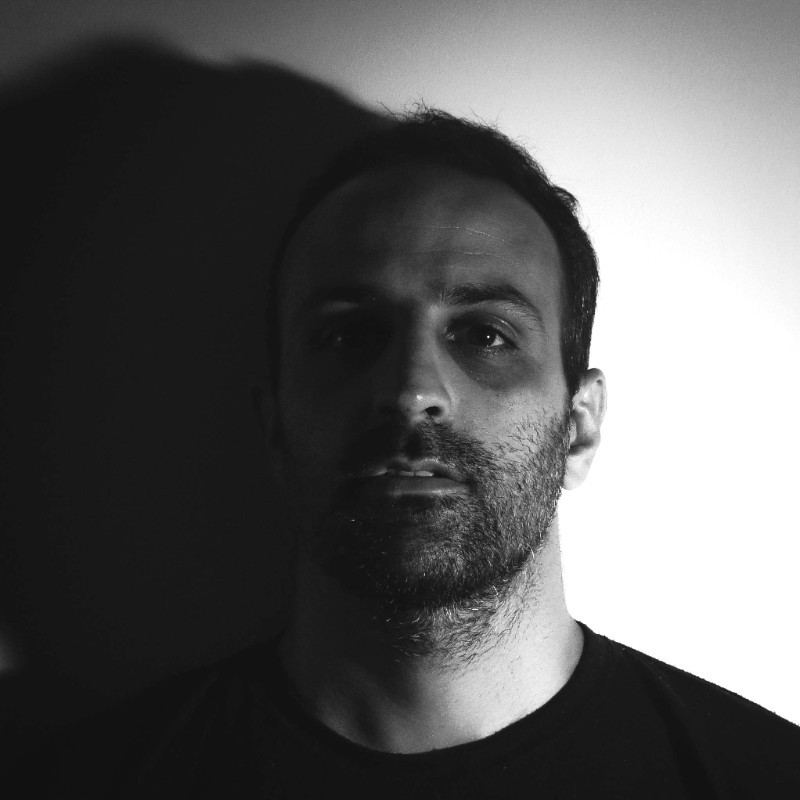
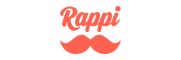