Exploring the Flexibility of PHP’s Variable Scope
When it comes to programming languages, variable scope plays a vital role in determining how variables are accessed and manipulated within different parts of a program. In PHP, a popular server-side scripting language, variable scope is a fundamental concept that every developer should understand to write efficient and maintainable code. PHP’s variable scope is remarkably flexible, allowing developers to control the visibility and accessibility of variables across various parts of their codebase. In this blog post, we’ll dive into the world of PHP’s variable scope, exploring the nuances of global, local, static, and superglobal variables, accompanied by code samples and practical insights.
1. Understanding Variable Scope:
At its core, variable scope refers to the region of a program where a variable can be accessed and manipulated. In PHP, there are several types of variable scope that determine where a variable is valid and accessible.
2. Global Variables:
A global variable is one that can be accessed from any part of the codebase, both inside functions and outside them. This type of variable is declared outside of any function and is available throughout the entire script.
Example:
php $globalVar = 10; function modifyGlobal() { global $globalVar; $globalVar += 5; } modifyGlobal(); echo $globalVar; // Output: 15
In this example, the global keyword is used inside the function to access the global variable $globalVar and modify its value. This flexibility comes with a caveat: overusing global variables can lead to code that is difficult to debug and maintain. It’s considered good practice to limit the use of global variables and opt for more controlled forms of variable scope.
3. Local Variables:
Local variables are defined within a function and are only accessible within that function’s scope. They are created when the function is called and destroyed when the function completes its execution.
Example:
php function calculateSum($a, $b) { $sum = $a + $b; return $sum; } $result = calculateSum(3, 7); echo $sum; // This will result in an error, as $sum is a local variable
In this example, the variable $sum is local to the calculateSum function, and attempting to access it outside the function’s scope will result in an error. This encapsulation ensures that variables within a function do not interfere with variables in other parts of the code.
4. Static Variables:
A static variable is a local variable that retains its value between multiple calls to the same function. It’s declared using the static keyword. Static variables are especially useful when you need to maintain state information across function calls.
Example:
php function countVisitors() { static $visitorCount = 0; $visitorCount++; echo "You are visitor number $visitorCount."; } countVisitors(); // Output: You are visitor number 1. countVisitors(); // Output: You are visitor number 2.
In this example, the variable $visitorCount retains its value across multiple calls to the countVisitors function, allowing us to keep track of the number of visitors.
5. Superglobal Variables:
Superglobal variables are predefined variables in PHP that are always accessible, regardless of scope. They provide essential information and data, such as form inputs, server details, and session information.
Example:
php echo $_GET['id']; // Accessing a URL parameter echo $_POST['username']; // Accessing a form input
In this snippet, $_GET and $_POST are examples of superglobal variables. They hold data passed through URL parameters and form submissions, respectively. These variables are automatically populated by PHP and can be accessed from any part of the script.
6. Variable Scope Hierarchy:
When dealing with variable scope, PHP follows a specific hierarchy to determine which variable to use if there are multiple variables with the same name. The hierarchy is as follows:
- Local scope
- Enclosing functions (if applicable)
- Global scope
- Superglobal scope
This means that if a variable exists in the local scope of a function, that variable will take precedence over variables with the same name in enclosing functions, the global scope, or the superglobal scope.
7. Tips for Effective Variable Scope Management:
- Avoid Global Variables: While global variables offer convenience, they can lead to code that is hard to maintain and debug. Use them sparingly and consider alternatives like passing variables as function arguments or using object-oriented programming principles.
- Use Descriptive Variable Names: Naming variables descriptively can help prevent naming conflicts and improve code readability. This is especially important when dealing with variables across different scopes.
- Limit Variable Lifetime: Declare variables in the smallest scope necessary to fulfill their purpose. This ensures that variables are not accessible longer than required.
- Use Static Variables Wisely: Static variables are valuable for maintaining state, but be cautious when using them. Make sure they are necessary and used appropriately to avoid unexpected behavior.
- Sanitize Superglobal Variables: Superglobal variables can introduce security risks, such as SQL injection or cross-site scripting. Always sanitize and validate input from superglobal variables before using them in your code.
Conclusion
Understanding PHP’s variable scope is essential for writing clean, organized, and maintainable code. By leveraging global, local, static, and superglobal variables effectively, developers can control the visibility and accessibility of data within their applications. Remember that while PHP’s flexibility in variable scope provides power, it also demands responsibility. Strive for a balance between convenience and best practices to ensure that your codebase remains robust and free from unnecessary complications. As you continue to explore PHP’s capabilities, mastering variable scope will undoubtedly enhance your ability to craft efficient and reliable applications.
Table of Contents
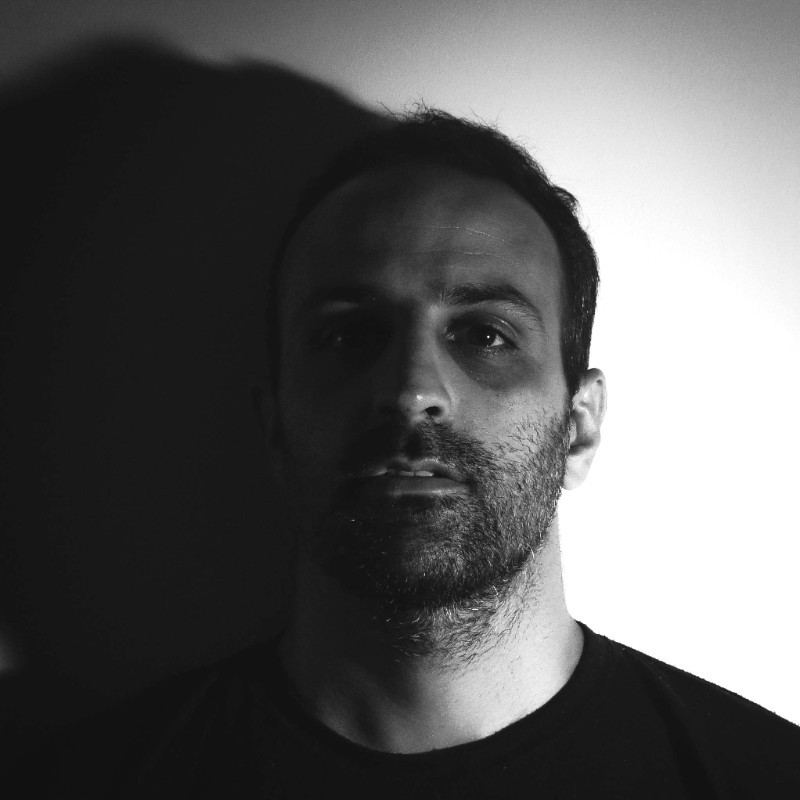
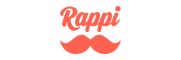