Demystifying PHP’s Variable Variables
PHP, a popular server-side scripting language, offers a plethora of features that enable developers to write dynamic and flexible code. One such feature that might initially appear puzzling is “variable variables.” This concept allows developers to create and manipulate variable names dynamically, opening the door to a wide range of possibilities. In this blog post, we’ll delve into the world of PHP’s variable variables, breaking down the concept, providing practical examples, and exploring use cases.
1. Understanding Variable Variables
At its core, a variable in PHP is a named container that holds a value. A variable’s name is static and determined at the time of coding. However, there are instances when dynamically generating variable names becomes necessary. This is where variable variables come into play. A variable variable is a variable that uses the value of another variable as its name.
Let’s take a look at a simple example:
php $fruit = "apple"; $$fruit = 5; // Creates a variable $apple with the value 5 echo $apple; // Outputs: 5
In this example, the value of the $fruit variable is “apple”. By using $$fruit, we create a new variable named $apple and assign it the value 5.
2. Creating Variable Variables
To create a variable variable, you need to use the double dollar sign ($$) followed by the variable whose value will be used as the name of the new variable. This allows you to generate variable names dynamically based on runtime conditions.
php $language = "PHP"; $$language = "Hypertext Preprocessor"; echo $PHP; // Outputs: Hypertext Preprocessor
Here, the variable variable $$language dynamically creates a variable named $PHP with the value “Hypertext Preprocessor”.
3. Variable Variables and Arrays
Variable variables can also be used with arrays. Consider the following example:
php $fruits = ["apple", "banana", "cherry"]; $selectedFruit = "banana"; $$selectedFruit = "My favorite fruit is banana!"; echo $banana; // Outputs: My favorite fruit is banana!
In this scenario, $$selectedFruit generates a variable named $banana and assigns the value “My favorite fruit is banana!”.
4. Use Cases for Variable Variables
Variable variables can be powerful when used judiciously. They are particularly handy when dealing with repetitive tasks or when generating dynamic content based on user input.
4.1. Loop Iterations
Suppose you want to create a series of variables within a loop. Variable variables make this task manageable:
php for ($i = 1; $i <= 3; $i++) { $$"item$i" = "Item number $i"; echo ${"item$i"} . "\n"; }
In this example, the loop creates variables $item1, $item2, and $item3, each with distinct values.
4.2. Form Handling
When processing form submissions, variable variables can help streamline the code by dynamically generating variable names based on field names:
php foreach ($_POST as $field => $value) { $$field = sanitize($value); // Assume a sanitize function exists }
Here, if the form contains fields named username, email, and message, the loop generates corresponding variables with sanitized values.
4.3. Language Localization
In multilingual applications, you can use variable variables to store and retrieve localized messages:
php $language = getUserLanguage(); // Assume a function to get user's preferred language $messageKey = "welcome_message_" . $language; $$messageKey = getLocalizedMessage($language); // Get the localized welcome message echo $welcome_message_en; // Outputs: Welcome to our website!
5. Potential Pitfalls and Security Considerations
While variable variables offer flexibility, they also introduce potential pitfalls and security concerns. Using variable variables excessively can lead to confusion and hard-to-maintain code. Additionally, if not handled carefully, they can open doors to vulnerabilities like code injection.
To mitigate these risks, consider the following tips:
- Clear Naming Conventions: If you opt to use variable variables, maintain a consistent and clear naming convention to make your code more readable.
- Input Sanitization: When using variable variables based on user input, ensure proper input sanitization to prevent injection attacks.
- Alternative Approaches: In many cases, associative arrays can be a safer and more organized alternative to achieve dynamic naming.
- Code Reviews: Have your code reviewed by peers to identify any potential confusion or security risks introduced by variable variables.
Conclusion
PHP’s variable variables might initially seem perplexing, but once understood, they can become a powerful tool in your development arsenal. With the ability to create and manipulate variable names dynamically, you can tackle a variety of tasks more efficiently. From loop iterations to form handling and beyond, variable variables provide a unique way to write dynamic and adaptable code. However, exercise caution and adhere to best practices to avoid pitfalls and security vulnerabilities. By mastering this feature, you’ll expand your PHP coding capabilities and unlock new possibilities in your projects.
Table of Contents
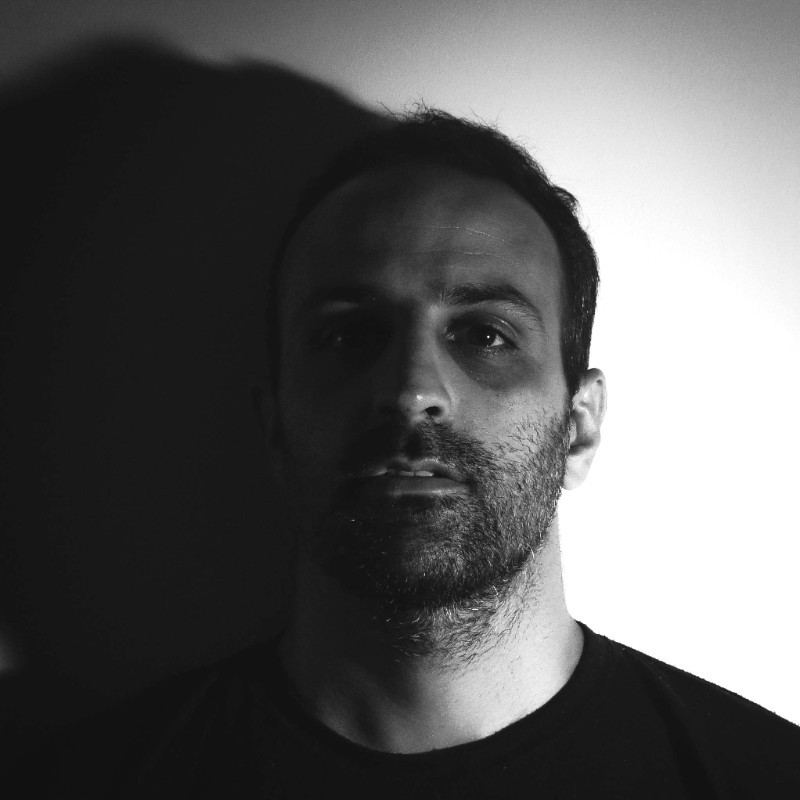
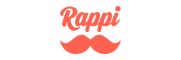