PHP and HTML5: A Powerful Duo for Web Development
In the world of web development, having a strong foundation is crucial for building robust and dynamic websites and applications. Two technologies that have stood the test of time and continue to dominate the web development landscape are PHP and HTML5. PHP is a server-side scripting language, while HTML5 is the latest version of the standard markup language used to structure and present content on the web. When combined, these two technologies form a powerful duo that enables developers to create feature-rich and interactive web experiences. In this blog, we will explore the roles of PHP and HTML5 in web development, understand their synergy, and showcase code samples to demonstrate the magic they create together.
1. The Role of PHP in Web Development
1.1. Server-Side Processing
PHP is primarily known for its role in server-side processing. Unlike client-side languages like JavaScript, PHP code is executed on the server, allowing it to interact with databases, handle form submissions, and perform various operations that require server resources. This server-side processing ensures that sensitive information, like database credentials and backend logic, is not exposed to users, making PHP a secure choice for web development.
1.2. Dynamic Content Generation
Dynamic content is essential to create personalized and engaging web experiences. With PHP, developers can generate dynamic content by embedding PHP code directly into HTML pages. This allows them to display user-specific data, such as personalized greetings, user profiles, and real-time information. The ability to dynamically generate content adds a layer of interactivity to web applications, enhancing user engagement.
1.3. Database Interaction
Modern web applications often rely on databases to store and manage data. PHP has excellent support for database interaction, making it easy for developers to connect to databases, execute queries, and retrieve or modify data. Whether it’s MySQL, PostgreSQL, or other popular database systems, PHP offers extensive libraries and extensions to simplify the database integration process.
1.4. Server-Side Validation
Validating user input is a crucial security measure to prevent data vulnerabilities and ensure data integrity. PHP provides built-in functions and libraries for server-side validation, allowing developers to verify user input and protect against potential threats, such as SQL injection and cross-site scripting (XSS) attacks. By employing server-side validation, developers can build robust and secure web applications.
2. The Role of HTML5 in Web Development
2.1. Semantic Markup
HTML5 introduces a set of new elements and attributes that provide more meaning to the content within a web page. These semantic elements, such as <header>, <nav>, <article>, and <footer>, help search engines and screen readers better understand the structure of a web page. By using semantic markup, developers can enhance accessibility, SEO, and overall code readability.
2.2. Audio and Video Support
HTML5 comes with native support for embedding audio and video content directly into web pages, eliminating the need for third-party plugins like Flash. This native support not only enhances the user experience by allowing seamless media playback but also contributes to better compatibility across different devices and browsers.
2.3. Canvas and SVG
HTML5 introduces the <canvas> element, which enables developers to draw and render graphics and animations directly within the browser using JavaScript. This feature is especially useful for creating interactive games, data visualizations, and other dynamic content. Additionally, HTML5 includes support for Scalable Vector Graphics (SVG), allowing developers to create resolution-independent graphics that scale smoothly on any screen size.
2.4. Offline Web Applications
HTML5 introduces the concept of offline web applications using the Application Cache (AppCache) and Service Workers. By leveraging these features, developers can enable web applications to function even when users are offline or experiencing poor network connectivity. Offline web applications enhance user experience and productivity, making them an invaluable addition to modern web development.
3. The Synergy: PHP and HTML5 Together
3.1. Seamless Integration
One of the significant advantages of using PHP and HTML5 together is their seamless integration. PHP code can be embedded directly into HTML pages, and PHP scripts can generate dynamic HTML content, making it easy for developers to combine both technologies harmoniously. This integration allows developers to create feature-rich web applications that harness the power of server-side processing and interactivity provided by HTML5.
3.2. Data-driven Web Applications
The combination of PHP and HTML5 is particularly powerful for building data-driven web applications. PHP can handle database interactions and process user input, while HTML5 provides the structure and interactivity required to present the data to users. The result is a dynamic web application that can display real-time information, handle user interactions, and respond to user actions promptly.
3.3. Enhanced User Experience
With PHP and HTML5 working together, developers can create web applications that offer an enhanced user experience. The dynamic content generated by PHP keeps users engaged, while HTML5’s multimedia support allows for seamless integration of audio, video, and graphics. Additionally, the use of semantic HTML5 elements improves accessibility and search engine optimization, further enhancing the user experience.
4. Code Samples
4.1. Dynamic Content Generation with PHP
php <!DOCTYPE html> <html> <head> <title>Welcome User</title> </head> <body> <?php $username = "John Doe"; // Replace this with user-specific data from the database echo "<h1>Welcome, $username!</h1>"; ?> </body> </html>
4.2. Database Interaction with PHP
php <?php // Connect to the database (replace db_host, db_username, db_password, and db_name with actual credentials) $conn = mysqli_connect("db_host", "db_username", "db_password", "db_name"); // Check connection if (!$conn) { die("Connection failed: " . mysqli_connect_error()); } // Execute a sample query to retrieve data from the database $sql = "SELECT * FROM users"; $result = mysqli_query($conn, $sql); // Display the retrieved data if (mysqli_num_rows($result) > 0) { echo "<ul>"; while ($row = mysqli_fetch_assoc($result)) { echo "<li>" . $row["username"] . "</li>"; } echo "</ul>"; } else { echo "No users found."; } // Close the database connection mysqli_close($conn); ?>
4.3. Using HTML5 Canvas for Graphics
html <!DOCTYPE html> <html> <head> <title>HTML5 Canvas Example</title> </head> <body> <canvas id="myCanvas" width="400" height="200" style="border:1px solid #000;"></canvas> <script> var canvas = document.getElementById("myCanvas"); var ctx = canvas.getContext("2d"); ctx.fillStyle = "#FF0000"; ctx.fillRect(50, 50, 150, 100); </script> </body> </html>
Conclusion
In conclusion, PHP and HTML5 form a powerful duo for web development, providing developers with the tools they need to build dynamic, interactive, and data-driven web applications. PHP’s server-side processing capabilities and database interaction complement HTML5’s semantic markup and multimedia support, resulting in an enhanced user experience. By harnessing the strengths of both technologies, developers can create modern web applications that cater to the ever-evolving needs of users in the digital age. Whether you’re a seasoned web developer or just starting your journey, mastering the combination of PHP and HTML5 is essential for staying ahead in the competitive world of web development.
Table of Contents
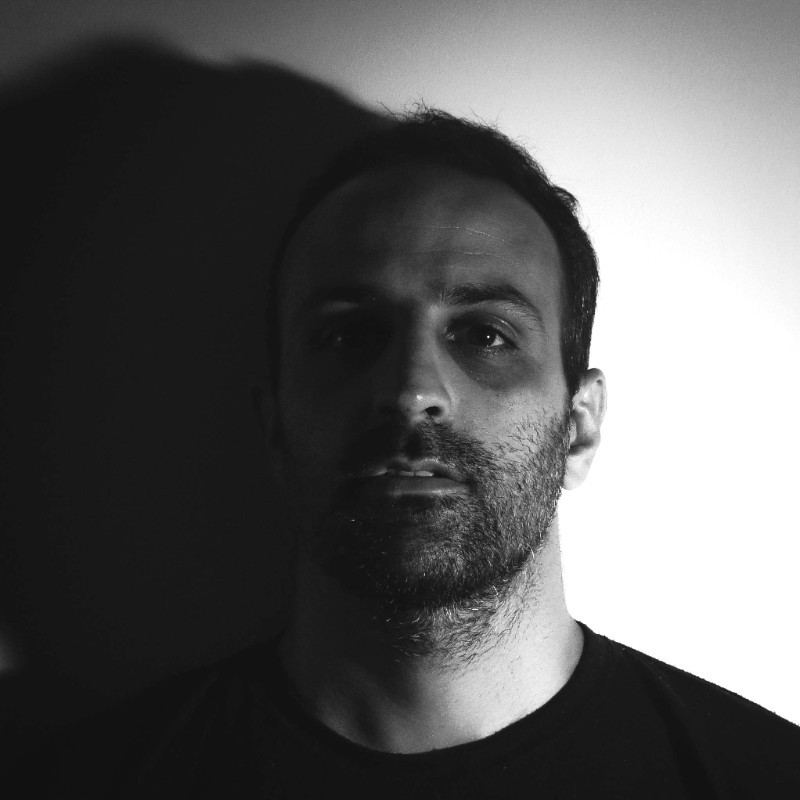
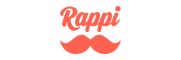