Revolutionizing Web Development with PHP 7
The digital landscape is constantly evolving, and web development is at the forefront of this transformation. PHP, a widely-used server-side scripting language, has played a pivotal role in shaping the web. With the release of PHP 7, a revolutionary leap was made in the world of web development. In this blog, we’ll delve into the game-changing features of PHP 7 that have redefined how developers create dynamic and interactive websites. From improved performance to enhanced syntax, let’s unravel the power of PHP 7.
1. The Need for Evolution:
Before we dive into the details of PHP 7, let’s understand why this evolution was necessary. PHP has been a stalwart in web development for years, but as websites became more complex and demanding, the limitations of earlier versions started to show. Slow performance and inadequate memory usage were among the challenges developers faced. PHP 7 was developed to address these concerns and provide a more efficient and developer-friendly environment.
1.1. Enhanced Performance:
One of the most significant improvements in PHP 7 is its performance boost. Thanks to the implementation of the new Zend Engine 3.0, PHP 7 boasts impressive speed improvements over its predecessor, PHP 5.6. This engine optimization allows PHP 7 to execute code almost twice as fast, leading to quicker loading times and enhanced user experiences.
1.1.1. Engine Optimization and Performance Benchmarks
To understand the impact of PHP 7’s performance enhancement, let’s look at a simple code snippet that demonstrates the difference in execution time between PHP 5.6 and PHP 7.
php // PHP 5.6 $start = microtime(true); for ($i = 0; $i < 1000000; $i++) { // Some computation } $end = microtime(true); $executionTime = $end - $start; echo "Execution time for PHP 5.6: " . $executionTime . " seconds"; // PHP 7 $start = microtime(true); for ($i = 0; $i < 1000000; $i++) { // Some computation } $end = microtime(true); $executionTime = $end - $start; echo "Execution time for PHP 7: " . $executionTime . " seconds";
In most cases, the execution time of PHP 7 will be noticeably faster than that of PHP 5.6, showcasing the performance leap that PHP 7 brings to the table.
1.2. Improved Memory Usage:
Memory consumption is a crucial factor in web applications, especially when handling multiple concurrent users. PHP 7 addresses this concern by optimizing its memory usage, resulting in reduced memory footprint for your applications. This enhancement allows developers to build more scalable and efficient web applications that can handle higher traffic without straining server resources.
1.2.1. Memory Usage Comparison
Let’s consider a scenario where an application needs to process a large dataset and store it in memory. We’ll compare the memory usage of PHP 5.6 and PHP 7 for this task.
php // PHP 5.6 $data = range(1, 1000000); // Creating an array with numbers from 1 to 1000000 echo "Memory used by PHP 5.6: " . memory_get_usage() . " bytes"; // PHP 7 $data = range(1, 1000000); echo "Memory used by PHP 7: " . memory_get_usage() . " bytes";
You’ll likely observe that PHP 7 consumes less memory compared to PHP 5.6 for the same task, showcasing its efficiency in memory management.
1.3. Scalar Type Declarations:
PHP 7 introduced scalar type declarations, allowing developers to specify the data types of function arguments and return values. This feature not only enhances code readability but also provides clearer insights into the expected input and output of functions.
1.3.1. Using Scalar Type Declarations
Consider a function that calculates the area of a rectangle. With scalar type declarations, you can ensure that the function receives the correct data types and returns an appropriate value.
php // Without scalar type declaration (PHP 5.6) function calculateRectangleArea($length, $width) { return $length * $width; } // With scalar type declaration (PHP 7) function calculateRectangleArea(float $length, float $width): float { return $length * $width; }
In PHP 7, using scalar type declarations helps prevent unexpected data type-related errors and enhances the predictability of function behavior.
1.4. Return Type Declarations:
In addition to scalar type declarations, PHP 7 introduced return type declarations. This feature allows developers to specify the data type that a function is expected to return. By doing so, you can ensure that your functions consistently produce the desired output.
1.4.1. Specifying Return Type Declarations
Let’s extend the previous example and add a return type declaration to our function that calculates the area of a rectangle.
php // With return type declaration (PHP 7) function calculateRectangleArea(float $length, float $width): float { return $length * $width; }
By specifying the return type as float, you communicate the expected output of the function and enhance the clarity of your code.
1.5. Null Coalescing Operator:
Handling default values when dealing with potentially null variables can be cumbersome. PHP 7 introduced the null coalescing operator (??) to simplify this process. This operator allows you to provide a default value for a variable if it is null.
1.5.1. Simplifying Default Value Assignment
Consider a scenario where you want to assign a default name if the user’s name is not provided.
php // Without null coalescing operator $userName = isset($_GET['name']) ? $_GET['name'] : 'Guest'; // With null coalescing operator (PHP 7) $userName = $_GET['name'] ?? 'Guest';
Using the null coalescing operator streamlines the process of assigning default values, making your code more concise and readable.
Conclusion
PHP 7 has undoubtedly revolutionized web development by addressing the performance, memory usage, and coding clarity issues that plagued earlier versions. Its enhanced speed, memory optimization, and features like scalar type declarations and the null coalescing operator empower developers to create more efficient, reliable, and maintainable web applications. By embracing PHP 7, you can unlock a new era of web development that caters to the demands of modern digital experiences. So, whether you’re a seasoned developer or just starting on your coding journey, PHP 7 is your gateway to the future of web development.
Table of Contents
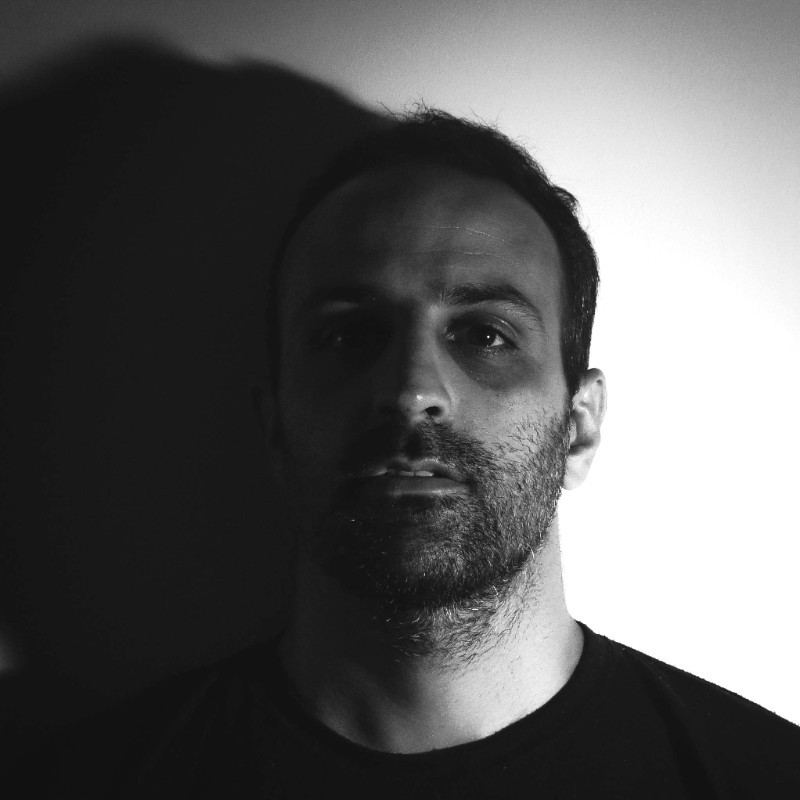
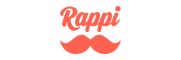