How to use async and await in Python?
`async` and `await` are two essential keywords introduced in Python 3.5, forming the core of asynchronous programming in Python. Their primary purpose is to write non-blocking code that performs tasks concurrently without the need for complex callback structures or threads. Here’s a brief overview:
- async: This keyword is used to declare a function as an asynchronous function. An asynchronous function doesn’t return the final result directly; instead, it returns an “awaitable” object, usually an instance of `asyncio.Future`. It indicates that the function will contain asynchronous operations and might pause its execution, giving control back to the event loop to perform other tasks.
- await: Within an `async` function, the `await` keyword is used before a function call to indicate that the current function should pause and wait for the awaited function to complete, without blocking the entire program’s flow. The awaited function should also be asynchronous. When the awaited function completes, control is given back to the original function, and it continues executing from where it left off.
Here’s the practical implication: Suppose you’re building a web scraper. Instead of waiting for each web page to download sequentially (which is time-consuming), you can start downloading multiple pages concurrently. While one page is being fetched, your code can proceed to initiate the download for the next page without waiting for the previous one to finish.
To leverage `async` and `await`, you often pair them with an asynchronous framework or library like `asyncio`. It provides tools like event loops, which manage and schedule the execution of multiple asynchronous tasks.
`async` and `await` offer a more readable and efficient way to write asynchronous code in Python. It allows for concurrent operations, making the most of system resources and potentially reducing the execution time of I/O-bound operations. Properly utilized, these constructs can greatly enhance the performance and structure of many Python applications.
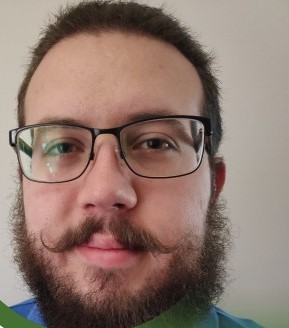
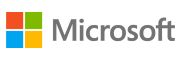