How to implement authentication and authorization in Python web apps?
Implementing authentication and authorization in Python web apps is crucial for ensuring only authorized users can access specific resources. Here’s a concise guide:
- Authentication:
Authentication is about verifying the identity of users. One of the most common methods is using a combination of a username (or email) and password. Once verified, a session or token is created to identify the user in subsequent requests.
– Flask: In Flask, you can use the `Flask-Login` extension for session-based authentication. For token-based, `Flask-JWT-Extended` is a popular choice.
– Django: Django comes with a built-in authentication system. You can use the `authenticate` function to check user credentials and `login` to attach the user to the session. For token-based authentication in Django REST Framework, you can use the `TokenAuthentication` method.
- Authorization:
Once a user is authenticated, authorization determines the permissions of that user, i.e., what they are allowed to do.
– Flask: `Flask-Principal` is a handy extension for role-based access control. For more fine-grained permissions, `Flask-User` and `Flask-Security` provide a range of features to handle user roles and permissions.
– Django: Django’s built-in system offers groups, permissions, and decorators like `@permission_required` to handle authorization. Django REST Framework also has a permissions system that allows for fine-tuning access to API endpoints.
- OAuth and Third-Party Authentication:
For allowing users to log in via third-party platforms (like Google or Facebook), OAuth is the standard protocol. Libraries like `Authlib` for Flask or `django-allauth` for Django can facilitate this integration.
- Password Security:
Always store passwords securely using hashing algorithms. Flask apps can utilize `Werkzeug’s` secure password hashing methods, while Django’s authentication system automatically handles password hashing.
While libraries and frameworks simplify the process, always stay updated with security best practices. Regularly review your code for potential vulnerabilities, especially when dealing with user data and authentication processes.
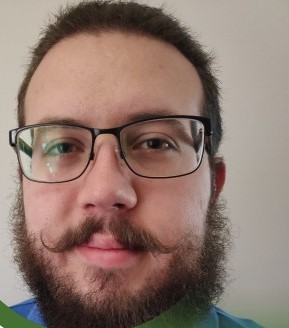
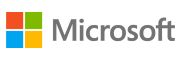