What are built-in data types in Python?
Python comes equipped with a variety of built-in data types that serve as the foundational building blocks for data manipulation and operations in the language. Understanding these types is crucial for effective Python programming.
- Numbers:
– Integers (`int`): Represent whole numbers, both positive and negative, e.g., `5`, `-42`.
– Floating-Point (`float`): Represent real numbers with decimal points, e.g., `3.14`, `-0.01`.
– Complex (`complex`): Represent complex numbers, e.g., `3 + 4j`.
- Boolean (`bool`): Represents truth values and can be either `True` or `False`.
- Strings (`str`): Sequences of Unicode characters enclosed within single, double, or triple quotes, e.g., `”Hello, World!”`.
- Lists (`list`): Ordered, mutable sequences that can contain items of any type. Items are enclosed within square brackets, e.g., `[1, 2, “apple”, 3.14]`.
- Tuples (`tuple`): Ordered, immutable sequences, similar to lists, but enclosed within parentheses, e.g., `(1, “banana”, 3, 4)`.
- Dictionaries (`dict`): Unordered collections of key-value pairs. They provide a way to store data without relying on indexing, e.g., `{“name”: “Alice”, “age”: 30}`.
- Sets (`set`): Unordered collections of unique elements. They are used primarily for membership testing and deduplication, e.g., `{1, 2, 3}`.
- Frozensets (`frozenset`): Immutable versions of sets. Once a frozenset is created, you cannot modify its content.
- Bytes (`bytes`): Immutable sequences of bytes (0-255). Useful for binary data operations.
- Byte Arrays (`bytearray`): Like bytes but mutable.
- Memory Views (`memoryview`): Provide a view object that exposes an array’s buffer interface, useful for handling data internal to an object without copying.
Each of these data types has its specific use cases and associated methods that allow you to manipulate, access, and manage the data they contain. By understanding the fundamental properties and capabilities of each type, Python developers can ensure they choose the most appropriate data structure for their tasks, leading to more efficient and readable code.
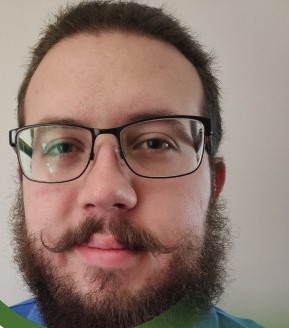
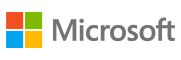