Understanding the Built-in Functions of Python
Python, as a versatile and powerful programming language, comes with a wide range of built-in functions that streamline and simplify various tasks. Whether you are a beginner or an experienced developer, understanding these built-in functions is essential for writing efficient and concise code.
In this blog, we will delve into the most commonly used built-in functions in Python and explore their functionalities with illustrative examples.
1. Introduction to Built-in Functions
What are Built-in Functions?
Built-in functions in Python are pre-defined functions that are readily available and don’t require any additional imports or installations. These functions are built into the Python interpreter and cover a wide array of operations, from simple calculations to complex data manipulations.
Advantages of Using Built-in Functions
Using built-in functions offers several advantages, including:
- Code Readability: Built-in functions provide intuitive and descriptive names, making your code easier to understand and maintain.
- Code Reusability: Instead of writing the same logic repeatedly, you can utilize built-in functions, promoting code reusability.
- Improved Efficiency: Built-in functions are optimized for performance and implemented in C, resulting in faster execution times.
- Shorter Code: By leveraging built-in functions, you can accomplish complex tasks with concise and elegant code.
2. Built-in Functions for Numbers
abs(): Absolute Value
The abs() function returns the absolute value of a number. It is used to convert negative numbers to positive numbers and leaves positive numbers unchanged.
python num = -10 absolute_value = abs(num) print(absolute_value) # Output: 10
round(): Rounding Numbers
The round() function rounds a number to the nearest integer or to the specified number of decimal places.
pi = 3.14159 rounded_pi = round(pi) print(rounded_pi) # Output: 3 number = 2.71828 rounded_number = round(number, 2) print(rounded_number) # Output: 2.72
max(): Maximum Value
The max() function returns the largest element from a collection of numbers or the largest of two or more arguments.
python numbers = [5, 8, 3, 10, 2] max_value = max(numbers) print(max_value) # Output: 10 x = 15 y = 25 maximum = max(x, y) print(maximum) # Output: 25
min(): Minimum Value
The min() function returns the smallest element from a collection of numbers or the smallest of two or more arguments.
python numbers = [5, 8, 3, 10, 2] min_value = min(numbers) print(min_value) # Output: 2 x = 15 y = 25 minimum = min(x, y) print(minimum) # Output: 15
3. Built-in Functions for Strings
len(): Length of a String
The len() function returns the length of a string, which is the number of characters in the string.
python message = "Hello, Python!" length = len(message) print(length) # Output: 14
str(): Converting Data to Strings
The str() function converts different data types into strings.
python number = 42 number_str = str(number) print(number_str) # Output: "42" pi = 3.14159 pi_str = str(pi) print(pi_str) # Output: "3.14159"
upper(), lower(), capitalize(): Case Manipulation
Python provides several functions to manipulate the case of strings.
python message = "hello, python!" upper_case = message.upper() print(upper_case) # Output: "HELLO, PYTHON!" lower_case = message.lower() print(lower_case) # Output: "hello, python!" capitalized = message.capitalize() print(capitalized) # Output: "Hello, python!"
split(): Splitting Strings
The split() function divides a string into a list of substrings based on a specified separator.
python sentence = "Python is amazing!" words = sentence.split() print(words) # Output: ['Python', 'is', 'amazing!'] csv_data = "John,Doe,30,New York" csv_list = csv_data.split(',') print(csv_list) # Output: ['John', 'Doe', '30', 'New York']
join(): Joining Strings
The join() function merges a sequence of strings into a single string using a specified separator.
python words = ['Python', 'is', 'amazing!'] sentence = ' '.join(words) print(sentence) # Output: "Python is amazing!" csv_list = ['John', 'Doe', '30', 'New York'] csv_data = ','.join(csv_list) print(csv_data) # Output: "John,Doe,30,New York"
4. Built-in Functions for Lists
len(): Length of a List
The len() function also works for lists, returning the number of elements in the list.
python numbers = [5, 8, 3, 10, 2] length = len(numbers) print(length) # Output: 5
sum(): Sum of List Elements
The sum() function calculates the sum of all elements in a list.
python numbers = [1, 2, 3, 4, 5] total = sum(numbers) print(total) # Output: 15
max(): Maximum Element in a List
The max() function can also find the maximum value from a list.
python numbers = [5, 8, 3, 10, 2] max_value = max(numbers) print(max_value) # Output: 10
min(): Minimum Element in a List
The min() function finds the minimum value from a list.
python numbers = [5, 8, 3, 10, 2] min_value = min(numbers) print(min_value) # Output: 2
sorted(): Sorting Lists
The sorted() function returns a new sorted list from the elements of any iterable.
python numbers = [5, 8, 3, 10, 2] sorted_numbers = sorted(numbers) print(sorted_numbers) # Output: [2, 3, 5, 8, 10] words = ["banana", "apple", "orange"] sorted_words = sorted(words) print(sorted_words) # Output: ['apple', 'banana', 'orange']
list(): Converting Data to Lists
The list() function is used to convert other data types, such as strings or tuples, to lists.
python text = "Python" char_list = list(text) print(char_list) # Output: ['P', 'y', 't', 'h', 'o', 'n'] tuple_data = (1, 2, 3, 4, 5) list_data = list(tuple_data) print(list_data) # Output: [1, 2, 3, 4, 5]
5. Built-in Functions for Dictionaries
len(): Length of a Dictionary
The len() function can determine the number of key-value pairs in a dictionary.
python person = {"name": "John", "age": 30, "city": "New York"} dict_length = len(person) print(dict_length) # Output: 3
keys(): Retrieving Dictionary Keys
The keys() function returns a view object of all the keys in a dictionary.
python person = {"name": "John", "age": 30, "city": "New York"} keys = person.keys() print(keys) # Output: dict_keys(['name', 'age', 'city'])
values(): Retrieving Dictionary Values
The values() function retrieves a view object containing all the values in a dictionary.
python person = {"name": "John", "age": 30, "city": "New York"} values = person.values() print(values) # Output: dict_values(['John', 30, 'New York'])
items(): Retrieving Dictionary Items
The items() function returns a view object of all the key-value pairs in a dictionary.
python person = {"name": "John", "age": 30, "city": "New York"} items = person.items() print(items) # Output: dict_items([('name', 'John'), ('age', 30), ('city', 'New York')])
6. Built-in Functions for Iterables
map(): Applying a Function to Elements
The map() function applies a given function to all items of an iterable and returns an iterator.
python numbers = [1, 2, 3, 4, 5] squared_numbers = map(lambda x: x**2, numbers) print(list(squared_numbers)) # Output: [1, 4, 9, 16, 25]
filter(): Filtering Elements
The filter() function creates an iterator from elements of an iterable for which a function returns True.
python numbers = [1, 2, 3, 4, 5] even_numbers = filter(lambda x: x % 2 == 0, numbers) print(list(even_numbers)) # Output: [2, 4]
any(): Checking for True values
The any() function returns True if any element in the iterable is True. Otherwise, it returns False.
python numbers = [0, 1, 0, 0, 0] result = any(numbers) print(result) # Output: True numbers = [0, False, '', None] result = any(numbers) print(result) # Output: False
all(): Checking for All True values
The all() function returns True if all elements in the iterable are True. If any element is False, it returns False.
python numbers = [1, 2, 3, 4, 5] result = all(x > 0 for x in numbers) print(result) # Output: True numbers = [1, 2, 3, 0, 5] result = all(x > 0 for x in numbers) print(result) # Output: False
7. Built-in Functions for Type Conversion
int(): Converting to Integer
The int() function converts a given value to an integer.
python num_str = "42" number = int(num_str) print(number) # Output: 42
float(): Converting to Float
The float() function converts a given value to a floating-point number.
python pi_str = "3.14159" pi = float(pi_str) print(pi) # Output: 3.14159
str(): Converting to String
The str() function converts a value to a string.
python number = 42 number_str = str(number) print(number_str) # Output: "42"
list(): Converting to List
The list() function converts an iterable (e.g., a string, tuple, or dictionary) to a list.
python text = "Python" char_list = list(text) print(char_list) # Output: ['P', 'y', 't', 'h', 'o', 'n'] tuple_data = (1, 2, 3, 4, 5) list_data = list(tuple_data) print(list_data) # Output: [1, 2, 3, 4, 5]
dict(): Converting to Dictionary
The dict() function creates a new dictionary from an iterable of key-value pairs.
python items = [("name", "John"), ("age", 30), ("city", "New York")] person = dict(items) print(person) # Output: {'name': 'John', 'age': 30, 'city': 'New York'}
Conclusion
In this blog, we have explored the fundamental built-in functions of Python and their applications. These built-in functions provide an incredible amount of utility and efficiency, making Python a popular choice among programmers.
By understanding and leveraging these built-in functions, you can write more expressive, readable, and concise code while saving valuable development time.
Remember, the best way to grasp these functions is through practice. Try experimenting with different examples and scenarios, and you’ll become proficient in using Python’s built-in functions to their full potential.
Happy coding!
Table of Contents
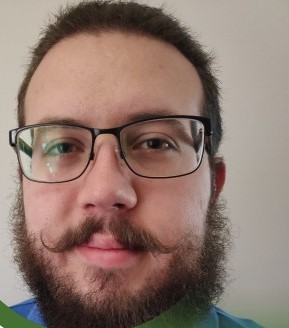
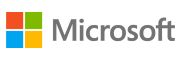