How to Use Python Functions to Calculate the Fibonacci Sequence
Python is an incredible language known for its versatility and user-friendly nature, making it a popular choice among beginners and experienced developers alike. Today, we will explore how to leverage Python’s power to calculate the Fibonacci sequence, an iconic series in the mathematical realm.
Before we dive into the code, let’s revisit the basics of the Fibonacci sequence. This sequence is a series of numbers in which each number is the sum of the two preceding ones, typically starting with 0 and 1. For example, the sequence begins as follows: 0, 1, 1, 2, 3, 5, 8, 13, and so forth.
Iterative Method
The most straightforward way to calculate the Fibonacci sequence is by using an iterative method. In Python, we can use a `for` loop for this purpose:
def fibonacci_iterative(n): if n <= 0: return "Input must be a positive integer" elif n == 1: return 0 elif n == 2: return 1 else: a, b = 0, 1 for i in range(2, n): a, b = b, a + b return b
In this function, `n` represents the position in the Fibonacci sequence you want to compute. If `n` is 1, the function returns 0, and if `n` is 2, it returns 1. For any `n` greater than 2, the function uses a `for` loop to calculate the Fibonacci number at that position.
The iterative method is a good start, but it has limitations. For example, it can be inefficient to calculate large Fibonacci numbers because the function must iterate through each number in the sequence.
Recursive Method
An alternative approach is to use recursion, which involves a function calling itself. Recursive methods are often more concise and may seem more ‘mathematical’. However, they can also be more challenging to understand initially:
def fibonacci_recursive(n): if n <= 0: return "Input must be a positive integer" elif n == 1: return 0 elif n == 2: return 1 else: return fibonacci_recursive(n-1) + fibonacci_recursive(n-2)
This recursive method directly implements the mathematical definition of the Fibonacci sequence. Unfortunately, it can be quite inefficient. The function makes two recursive calls for each call to `fibonacci_recursive`, resulting in an exponential number of calls as `n` increases.
Memoization Method
To address the inefficiencies of the recursive method, we can use a technique called memoization, which stores the results of expensive function calls and reuses them when the same inputs occur again:
def fibonacci_memoization(n, memo={}): if n <= 0: return "Input must be a positive integer" elif n in memo: return memo[n] elif n == 1: return 0 elif n == 2: return 1 else: result = fibonacci_memoization(n-1, memo) + fibonacci_memoization(n-2, memo) memo[n] = result return result
In the `fibonacci_memoization` function, `memo` is a dictionary that stores previously calculated Fibonacci numbers. Before the function makes any calculations, it first checks if the result is already in `memo`. If it is, the function returns the stored result, saving computation time.
Generator Method
Finally, Python provides a powerful concept known as generators. A generator is a function that behaves like an iterator, meaning it can be looped over to produce a sequence of results. Here’s how we can calculate the Fibonacci sequence using a generator:
def fibonacci_generator(n): a, b = 0, 1 for _ in range(n): yield a a, b = b, a + b
This function uses the `yield` keyword, which means that instead of calculating all Fibonacci numbers up to `n` immediately, it generates each number on-the-fly as you iterate over the function. This method is very memory efficient as it only stores the last two numbers in the sequence.
Conclusion
Python, with its user-friendly syntax and vast functionality, provides several ways to calculate the Fibonacci sequence. We’ve explored four methods today – iterative, recursive, memoization, and generator – each with its own strengths and weaknesses. Understanding these methods can not only help you calculate Fibonacci numbers efficiently but also aid in grasping essential programming concepts. As you continue your Python journey, remember that there’s often more than one way to solve a problem. The best method depends on your specific needs and constraints. Happy coding!
Table of Contents
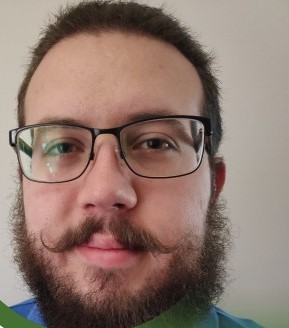
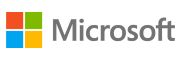