What are coding conventions and standards in Python?
Python’s coding conventions and standards are primarily outlined in PEP 8, which stands for Python Enhancement Proposal 8. PEP 8 provides coding guidelines that ensure code written by different authors remains consistent, readable, and maintainable. Adhering to these standards promotes best practices and makes it easier for other developers to understand and work with your code. Here are some key highlights:
- Indentation:
Use 4 spaces per indentation level. Avoid using tabs, and spaces are the preferred method.
- Maximum Line Length:
Limit lines to 79 characters for code, and 72 for comments.
- Imports:
Imports should usually be on separate lines and should be grouped in the following order: standard library imports, third-party imports, and local application/library-specific imports. You should put a blank line between each group of imports.
- Whitespace:
Avoid extra whitespace in situations like inside brackets, before a comma, or before a colon.
- Comments:
Comments should be clear and should be updated if the underlying code changes. Use inline comments sparingly and ensure they’re separated by at least two spaces from the statement.
- Naming Conventions:
– Use `snake_case` for functions and variable names.
– Use `CamelCase` for class names.
– For private names, use a leading underscore (`_private_var`).
- Function and Method Arguments:
If a function or method has many arguments, list them one per line, and align them vertically.
- Error Handling:
Avoid bare `except` clauses. It’s better to specify exceptions explicitly.
- Comparisons to Singletons:
Use `is` or `is not` for comparing singletons like `None`.
- String Quotes:
In Python, single and double quotes are the same. PEP 8 doesn’t make a recommendation, but it’s essential to be consistent within a project.
In addition to PEP 8, there’s PEP 257 that provides conventions for docstrings, ensuring clear documentation for functions, classes, and modules.
While these conventions are highly recommended, it’s essential to remember that readability counts. Sometimes, adhering strictly to PEP 8 might reduce readability, and in those cases, a balanced approach is wise. Using tools like `flake8` or `black` can assist in ensuring adherence to these standards.
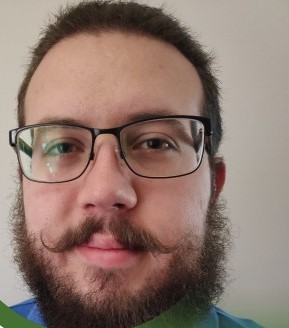
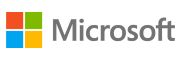