How to work with command-line arguments in Python?
Handling command-line arguments in Python is both a fundamental and invaluable skill, especially when creating scripts or utilities. The built-in module, `sys`, and the more advanced module, `argparse`, are typically utilized for this purpose.
- Using the `sys` module:
The `sys.argv` list from the `sys` module allows you to fetch command-line arguments. `sys.argv[0]` is the script name itself, and the actual arguments start from `sys.argv[1]`.
```python import sys print(sys.argv) # Output: ['script_name.py', 'arg1', 'arg2', ...] ```
However, while `sys` provides a direct way to access arguments, it doesn’t offer functionalities like parsing named arguments, generating help messages, or type checking.
- Using the `argparse` module:
For a more robust approach to handle command-line arguments, `argparse` is preferred.
Here’s a basic example:
```python import argparse parser = argparse.ArgumentParser(description="A simple script with command-line arguments.") parser.add_argument('-a', '--argument', help="Specify an argument.", required=True) args = parser.parse_args() print(args.argument) ```
Running this script with `-h` will automatically produce a helpful message explaining how to use the provided arguments. The module allows for easy specification of argument data types, default values, choices, and more.
While the `sys` module offers a quick and simple way to work with command-line arguments, the `argparse` module brings in robustness, making it the preferred choice for more intricate scripts and applications. By leveraging `argparse`, you can provide users with clear guidance, enforce argument structures, and parse input more effectively, ensuring that your Python programs are both user-friendly and resilient.
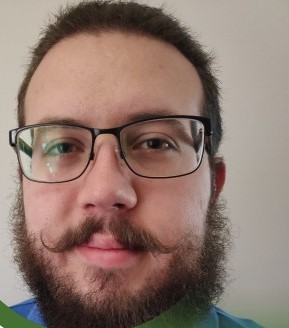
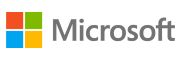