10 Common Python Functions Every Beginner Should Know
When it comes to programming, the significance of having a firm grasp on the basics cannot be overemphasized. Python, a high-level programming language, is no exception. Known for its simplicity and readability, Python is an excellent choice for beginners.
This article will introduce you to ten common Python functions that every novice should know. So, let’s dive in!
1. `print()`
The `print()` function is usually the first function beginners come across when learning Python. It is used to output data to the standard output device (your screen). Here’s a simple example:
print("Hello, World!")
This will output: `Hello, World!` to your screen.
2. `len()`
The `len()` function is used to get the length (the number of items) of an object. This function works with many data types, including strings, lists, tuples, etc. Here’s how you can use `len()`:
my_list = [1, 2, 3, 4, 5] print(len(my_list)) # Outputs: 5
3. `type()`
The `type()` function is used to find out the type of an object. Whether you’re dealing with integers, strings, lists, or more complex objects, `type()` comes in handy for understanding what kind of data you’re working with:
print(type(10)) # Outputs: <class 'int'> print(type('Hello')) # Outputs: <class 'str'>
4. `str()`
The `str()` function is used to convert a specified value into a string. It can convert integers, floats, lists, etc., into their string representation. Here’s how to use it:
print(str(100)) # Outputs: '100' print(str(123.45)) # Outputs: '123.45'
5. `int()`, `float()`
The `int()` and `float()` functions are used to convert a value into an integer or a floating-point number, respectively. These functions are important for performing accurate arithmetic operations.
print(int('100')) # Outputs: 100 print(float('123.45')) # Outputs: 123.45
6. `input()`
The `input()` function is used to take input from the user. By default, the input is taken as a string. If you want the input to be an integer or float, you can use the `int()` or `float()` function, respectively.
name = input("Enter your name: ") print("Hello, " + name)
7. `range()`
The `range()` function is commonly used in looping to generate a sequence of numbers. This function can accept 1, 2, or 3 parameters and returns a sequence of numbers, starting from 0 by default, incrementing by 1 (also default), and ending at a specified number.
for i in range(5): print(i) # Outputs: 0, 1, 2, 3, 4
8. `append()`
The `append()` function is used to add an element at the end of a list. It’s one of the most frequently used functions when working with lists.
my_list = [1, 2, 3] my_list.append(4) print(my_list) # Outputs: [1, 2, 3, 4]
9. `split()`
The `split()` method splits a string into a list where each word is a list item. By default, it splits at each space. However, you can specify the separator.
text = "Hello, World!" print(text.split()) # Outputs: ['Hello,', 'World!']
10. `join()`
The `join()` method takes all items in an iterable and joins them into one string. A string must be specified as the separator.
my_list = ['Hello', 'World'] print(' '.join(my_list)) # Outputs: 'Hello World'
And there you have it – 10 common Python functions that every beginner should know. Familiarizing yourself with these functions can provide a great foundation as you delve deeper into the world of Python programming. They will assist you in everything, from manipulating data types to handling user input, and from working with loops to dealing with strings and lists.
Conclusion
Python’s simplicity and power make it a versatile programming language, both for beginners and seasoned programmers alike. The 10 common Python functions we discussed, including `print()`, `len()`, `type()`, `str()`, `int()`, `float()`, `input()`, `range()`, `append()`, `split()`, and `join()`, form the bedrock of many basic and advanced Python operations.
Understanding these functions not only equips you with fundamental programming tools but also aids in writing more efficient and effective code. Additionally, these functions form a springboard from which you can delve deeper into Python’s expansive libraries and frameworks, which include machine learning, web development, and data analysis capabilities.
However, these are just the tip of the iceberg. Python boasts an incredibly rich set of built-in functions and methods that cater to a variety of needs. So, as you continue your Python journey, don’t stop here. Keep exploring, learning, and challenging yourself to become a proficient programmer. Remember, Rome wasn’t built in a day, and neither is a programmer. Keep practicing, and happy coding!
Table of Contents
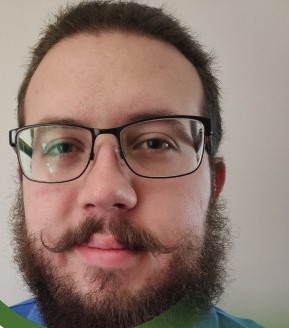
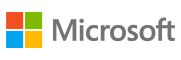