What’s the difference between Python 2 and Python 3
Python 2 and Python 3 represent two major versions of the Python programming language, and while they share many similarities, there are key differences that set them apart.
1. Print Statement vs. Print Function: In Python 2, `print` is a statement and doesn’t require parentheses. For example, `print “Hello, World!”` would suffice. In contrast, Python 3 treats `print` as a function, making parentheses mandatory: `print(“Hello, World!”)`.
2. Integer Division: In Python 2, dividing two integers results in floor division (unless you use a float). So, `5/2` would result in `2`. However, in Python 3, the same operation yields `2.5`, making division behavior more intuitive. If you want floor division in Python 3, you’d use `//`.
3. Unicode Representation: Python 2 uses ASCII as its default string encoding, and one has to prefix strings with ‘u’ to make them Unicode: `u”Hello”`. Python 3, on the other hand, uses Unicode by default for string types, streamlining global character handling.
4. xrange vs. range: In Python 2, there are two functions to produce sequences of numbers: `range()` (which produces a list) and `xrange()` (producing an iterator for better memory efficiency). Python 3 simplifies this by having just `range()`, which behaves like `xrange()` from Python 2.
5. Error Handling: Python 2 uses `not` and `print` syntax for exceptions, e.g., `except ValueError, e:`. Python 3 introduced a cleaner syntax: `except ValueError as e:`.
6. Standard Library Changes: Several standard library modules have been reorganized in Python 3, leading to name changes and division of certain modules.
7. End of Life: It’s vital to note that Python 2 reached its end of life on January 1, 2020, meaning no more updates, including security fixes. Hence, there’s a strong incentive to use or migrate to Python 3 for ongoing and new projects.
While Python 2 and 3 share a foundational philosophy, Python 3 brought enhancements, simplifications, and changes to ensure the language’s longevity and relevance. For new projects, Python 3 is undoubtedly the recommended choice.
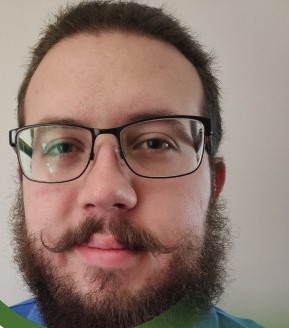
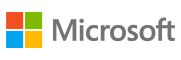