What is the difference between `==` and `is` in Python?
In Python, both `==` and `is` are comparison operators, but they serve distinct purposes and have crucial differences in their behavior and usage.
- `==` (Equality Operator):
– Purpose: The `==` operator checks for **value equality**. It compares whether the values on its left and right-hand sides are the same.
– Behavior: When you use `==`, Python checks if the objects referred to have the same content or value. It doesn’t concern itself with the actual memory addresses of the objects.
– Example: Two different lists with identical elements will be considered equal when compared using `==`.
- `is` (Identity Operator):
– Purpose: The `is` operator checks for **object identity**. It determines whether two variables refer to the exact same memory location (i.e., they reference the same object in memory).
– Behavior: When you use `is`, Python checks the memory addresses of the two objects. If they point to the same location, the result is `True`; otherwise, it’s `False`.
– Example: Even if two distinct lists have the same elements, using `is` to compare them will return `False` because they reside at different memory addresses.
Practical Implications:
– For atomic data types like numbers and strings, unexpected behaviors can arise. For instance, due to Python’s memory optimization strategies, small integers or strings might be stored at the same memory location. However, one shouldn’t rely on this behavior when using `is` to compare such values.
– It’s common to use `is` when comparing an object to `None`, as there’s only one instance of `None` in a Python program.
While both `==` and `is` are comparison operators in Python, they serve different roles. `==` checks if two objects have the same content or value, while `is` checks if two variables refer to the exact same memory location. Understanding this distinction is vital for preventing subtle bugs and writing clear, intention-revealing Python code.
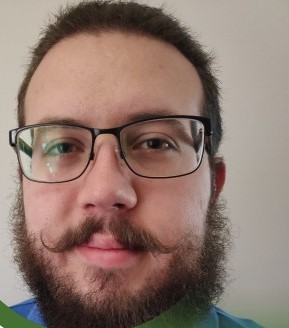
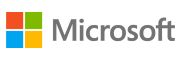